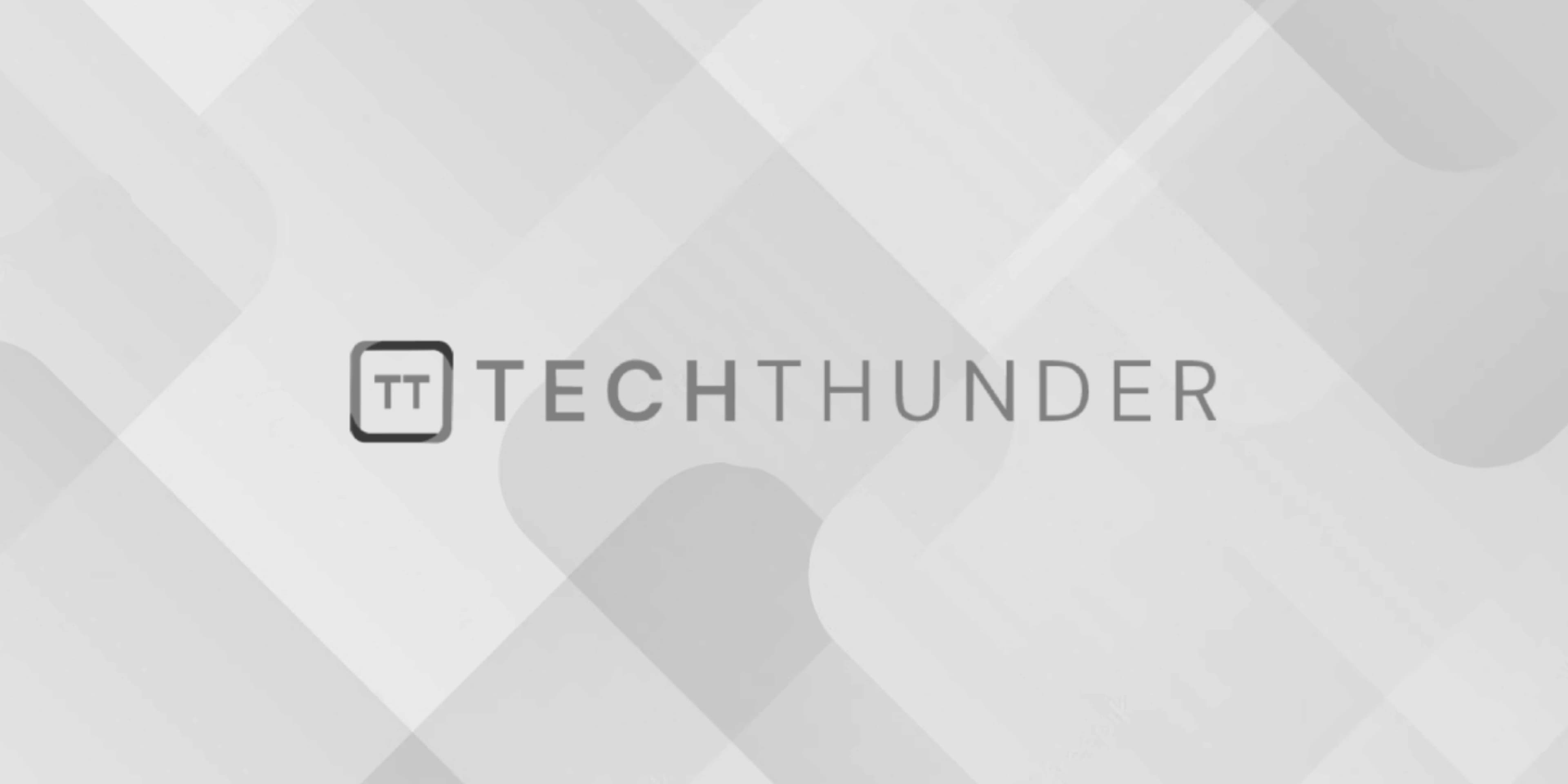
Calculate age using JavaScript
To calculate the age based on the birthdate using JavaScript, you can follow these steps:
1. Obtain the current date:
const currentDate = new Date();
2. Obtain the birthdate from the user:
Let’s assume the birthdate is entered as a string in the format “YYYY-MM-DD”. You can use any method to get the birthdate from the user, such as an input field or a date picker.
const birthdateString = '1990-05-15'; // Example birthdate
const birthdate = new Date(birthdateString);
3. Calculate the age:
Subtract the birthdate from the current date and extract the relevant information such as years, months, and days.
const ageInMilliseconds = currentDate - birthdate;
const ageDate = new Date(ageInMilliseconds);
const age = Math.abs(ageDate.getUTCFullYear() - 1970);
The getUTCFullYear()
method returns the year, and subtracting 1970 is necessary because the ageDate
is initialized with the Unix epoch time.
4. Display the age:
You can display the calculated age wherever you want, such as an HTML element.
const ageElement = document.getElementById('age'); // Example element
ageElement.textContent = `Age: ${age}`;
Replace 'age'
with the appropriate ID of the HTML element where you want to display the age.
That’s it! With these steps, you can calculate and display the age based on the given birthdate.