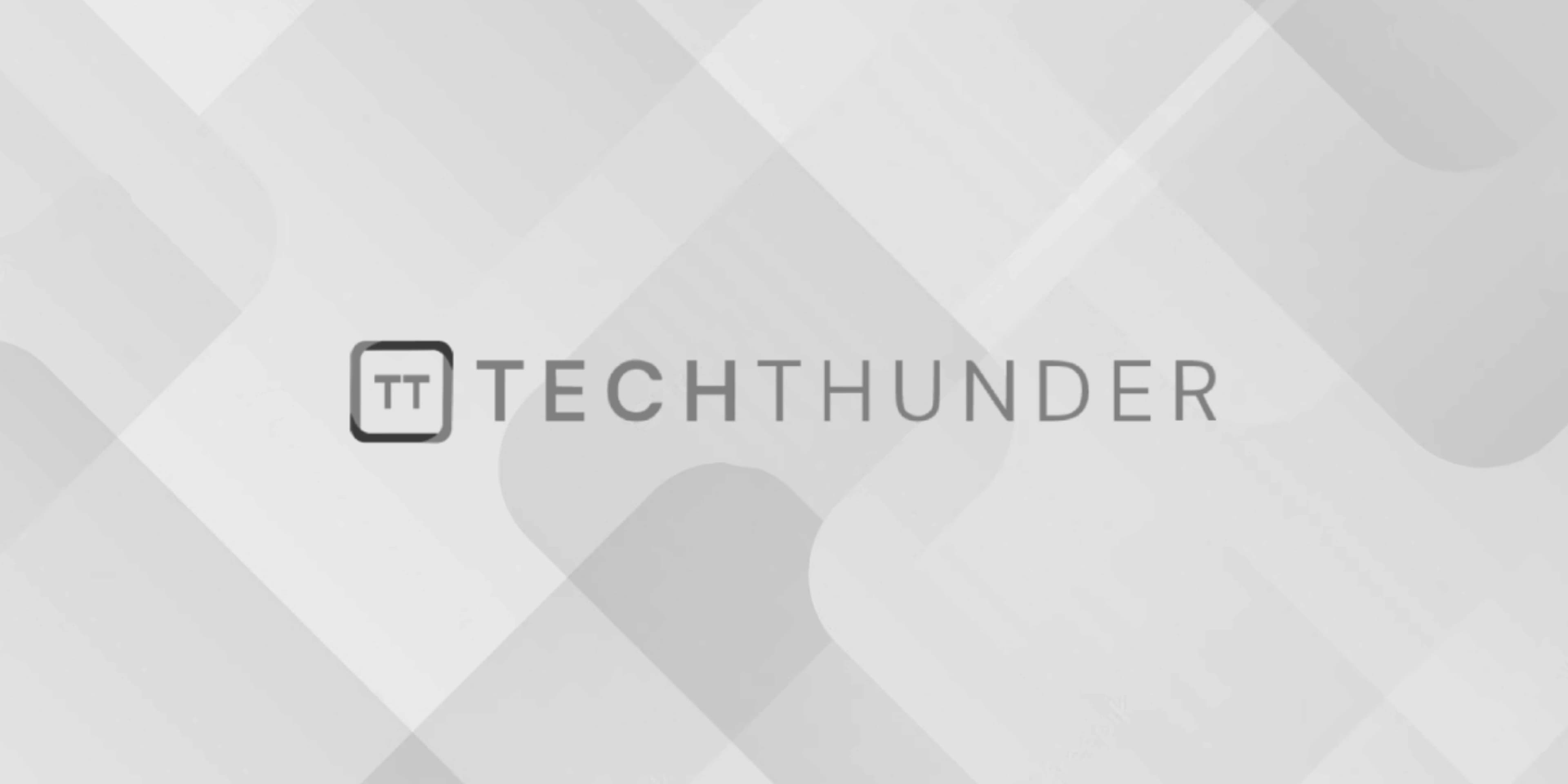
JavaScript WeakMap
JavaScript WeakMap
is a built-in object that represents a collection of key-value pairs in which the keys are weakly referenced. This means that if there are no other references to a key in your code, the key and its corresponding value can be garbage collected.
Unlike Map
, WeakMap
keys must be objects, and the keys are not enumerable, meaning you can’t iterate over the keys using methods like forEach
or for...of
.
Here’s an example of how to use WeakMap
:
// Create a WeakMap
const myWeakMap = new WeakMap();
// Create an object as a key
const key = {};
// Set a value in the WeakMap using the key
myWeakMap.set(key, 'Value');
// Get the value associated with the key
const value = myWeakMap.get(key);
console.log(value); // Output: Value
// Check if the key exists in the WeakMap
const hasKey = myWeakMap.has(key);
console.log(hasKey); // Output: true
// Delete the key-value pair from the WeakMap
myWeakMap.delete(key);
// Check if the key still exists
const hasKeyAfterDeletion = myWeakMap.has(key);
console.log(hasKeyAfterDeletion); // Output: false
In the above example, we create a WeakMap
called myWeakMap
. We create an object key
and use it as the key to set a value in the WeakMap
. We can then retrieve the value using get()
, check if the key exists using has()
, and delete the key-value pair using delete()
.
One important thing to note is that because the keys in WeakMap
are weakly referenced, they cannot be used for iterating over the WeakMap
or obtaining the size of the WeakMap
. WeakMap
is primarily useful in scenarios where you need to associate additional data with existing objects without affecting their normal garbage collection behavior.