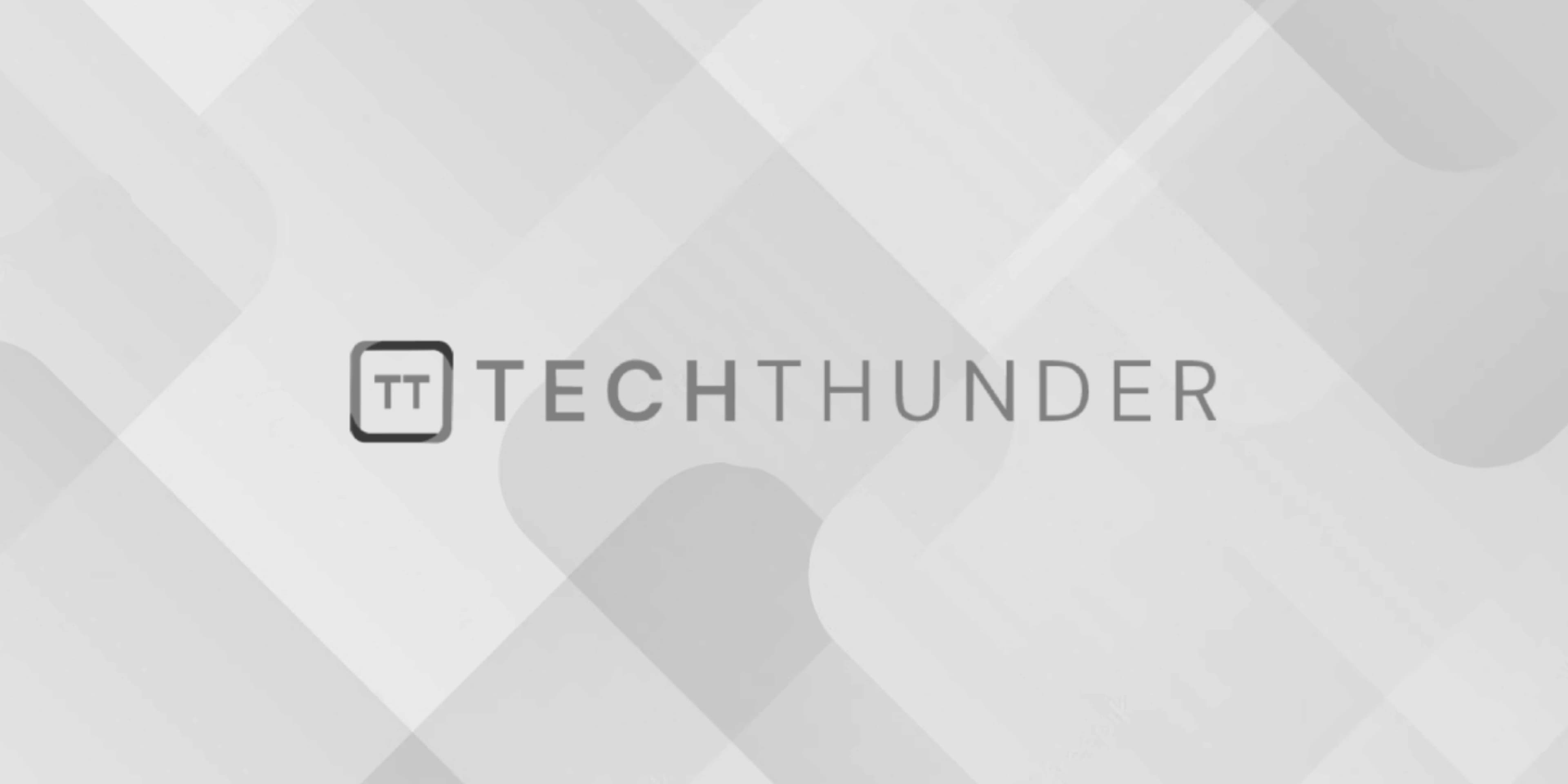
JavaScript getAttribute() method
The getAttribute()
method in JavaScript is used to retrieve the value of a specified attribute from an HTML element. It allows you to access the value of both standard and custom attributes on an element.
The syntax for using the getAttribute()
method is as follows:
element.getAttribute(attributeName)
Here, element
refers to the HTML element you want to retrieve the attribute from, and attributeName
is a string representing the name of the attribute you want to get.
For example, let’s say you have an HTML element with an id
attribute:
<div id="myDiv"></div>
You can use the getAttribute()
method to retrieve the value of the id
attribute:
var divElement = document.getElementById('myDiv');
var idValue = divElement.getAttribute('id');
console.log(idValue); // Output: myDiv
In this example, divElement
is assigned the reference to the HTML element with the id “myDiv”. Then, getAttribute('id')
is used to retrieve the value of the id
attribute, which is “myDiv”. The value is stored in the idValue
variable and printed to the console.
The getAttribute()
method can also be used to get the value of custom attributes:
<div data-custom-attribute="example"></div>
var divElement = document.querySelector('div');
var customAttribute = divElement.getAttribute('data-custom-attribute');
console.log(customAttribute); // Output: example
In this case, the getAttribute()
method is used to retrieve the value of the data-custom-attribute
attribute, which is “example”.
It’s important to note that getAttribute()
returns a string value or null
if the attribute does not exist on the element. If you want to access standard properties or attributes, you can often use the corresponding property directly on the element object instead of using getAttribute()
.