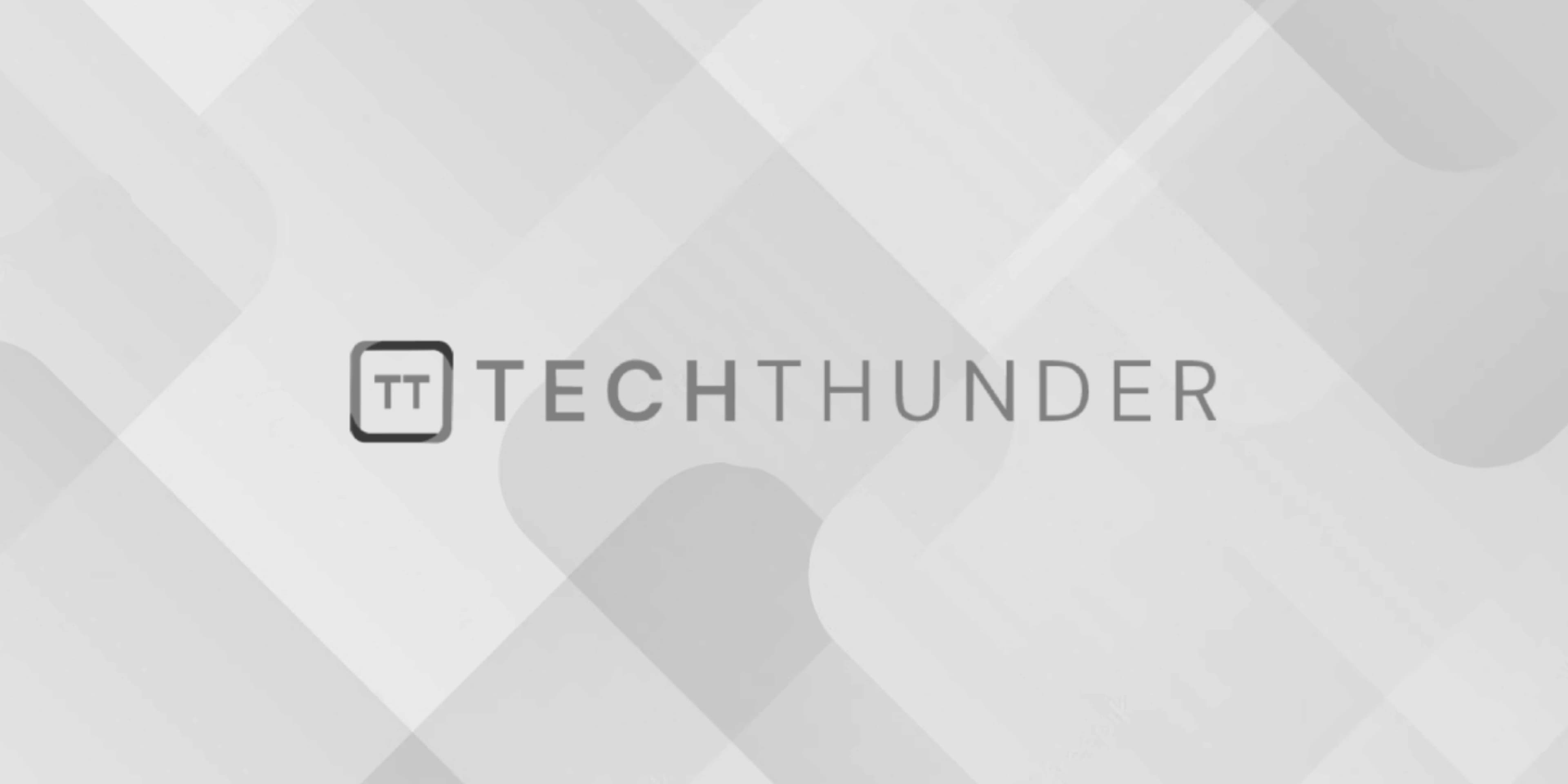
JavaScript static Method
The static methods are methods that are associated with a class rather than an instance of the class. They are defined directly on the class itself, not on the class’s prototype. Static methods are useful for defining utility functions or operations that are not specific to any particular instance of the class.
To define a static method in JavaScript, you use the static
keyword before the method name. Here’s an example:
class MathUtils {
static add(a, b) {
return a + b;
}
static multiply(a, b) {
return a * b;
}
}
console.log(MathUtils.add(5, 3)); // Output: 8
console.log(MathUtils.multiply(4, 2)); // Output: 8
In the above example, the MathUtils
class has two static methods: add
and multiply
. These methods can be called directly on the class without creating an instance of the class. Static methods are accessed using the class name followed by the method name, as shown in the console.log
statements.
Static methods cannot access the properties or methods of any specific instance of the class because they are not bound to any instance. They are self-contained and operate solely on the parameters passed to them.
Static methods are commonly used for utility functions or operations that do not require access to instance-specific data. They provide a way to organize related functionality within a class without the need for object instantiation.