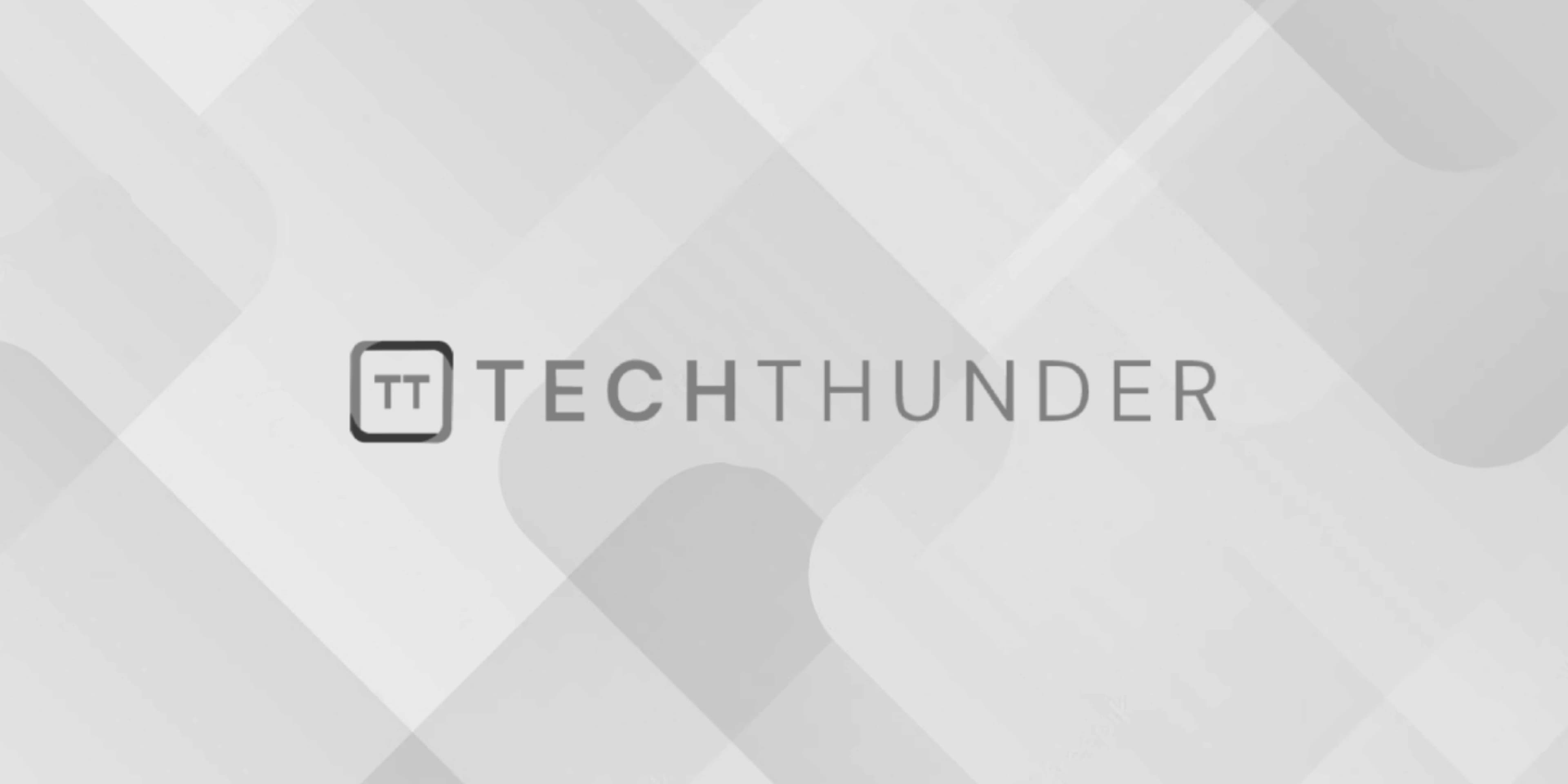
JavaScript this Keyword
The JavaScript this
keyword refers to the context in which a function is executed or an object on which a method is invoked. It provides a way to access and manipulate properties and methods within the current execution context.
The value of this
is determined dynamically at runtime based on how a function is called or how a method is invoked. The behavior of this
can vary depending on the context in which it is used. Here are some common use cases:
- Global scope: In the global scope (outside of any function),
this
refers to the global object, which is usually thewindow
object in a web browser. - Function context: When
this
is used inside a regular function (not an arrow function), its value is determined by how the function is called. It can refer to different objects or have different values based on the function invocation. - Object method: In the context of an object method,
this
refers to the object itself. It allows you to access the object’s properties and invoke other methods. - Constructor function: When a function is used as a constructor with the
new
keyword,this
refers to the newly created instance of the object. - Event handlers: In event handler functions,
this
typically refers to the element on which the event occurred.
Here’s an example to illustrate the usage of this
:
var person = {
name: 'John',
sayHello: function() {
console.log('Hello, ' + this.name);
}
};
person.sayHello(); // Output: Hello, John
In this example, this
inside the sayHello
method refers to the person
object. By using this.name
, we can access the name
property of the person
object.
It’s important to note that the value of this
is not lexically scoped like regular variables. It is determined dynamically at runtime based on the context of the function invocation. To ensure that this
behaves as expected, you need to understand the rules and context in which the function is called or the method is invoked.
It’s also worth mentioning that arrow functions (=>
) have a different behavior for this
, where they inherit this
from the surrounding scope and do not bind their own this
context.