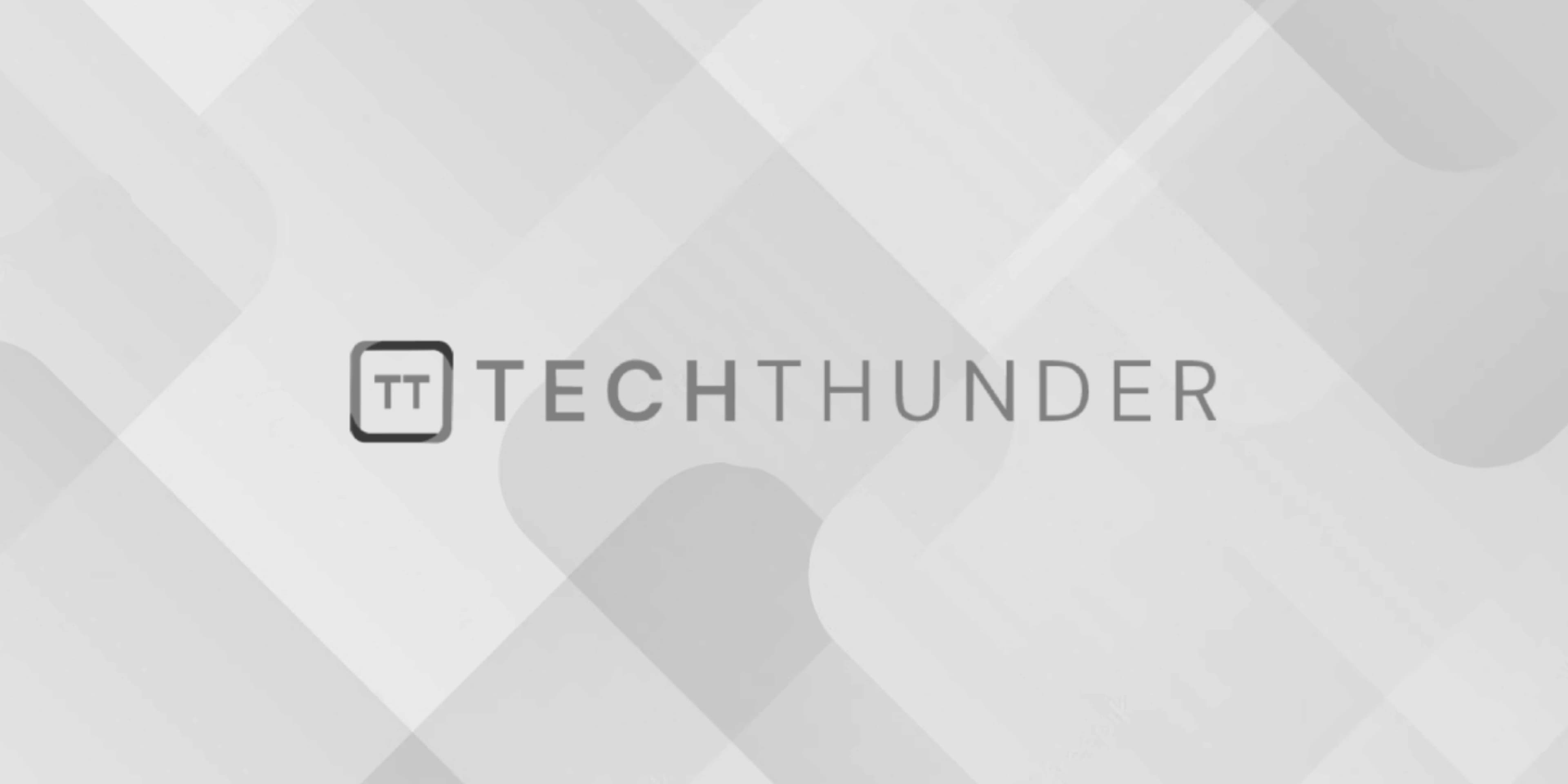
331 views
Implementing JavaScript Stack Using Array
Implementing a JavaScript stack using an array is relatively straightforward. A stack follows the Last-In-First-Out (LIFO) principle, meaning the most recently added item is the first one to be removed.
Sure! Here’s an implementation of a stack data structure using an array in JavaScript:
JavaScript
class Stack {
constructor() {
this.items = [];
}
// Push an element onto the stack
push(element) {
this.items.push(element);
}
// Pop the top element from the stack and return it
pop() {
if (this.isEmpty()) {
return null;
}
return this.items.pop();
}
// Return the top element of the stack without removing it
peek() {
if (this.isEmpty()) {
return null;
}
return this.items[this.items.length - 1];
}
// Check if the stack is empty
isEmpty() {
return this.items.length === 0;
}
// Return the size of the stack
size() {
return this.items.length;
}
// Clear the stack
clear() {
this.items = [];
}
}
// Example usage
const stack = new Stack();
stack.push(10);
stack.push(20);
stack.push(30);
console.log(stack.peek()); // Output: 30
console.log(stack.pop()); // Output: 30
console.log(stack.size()); // Output: 2
console.log(stack.isEmpty()); // Output: false
stack.clear();
console.log(stack.isEmpty()); // Output: true
In this implementation, the Stack
class has an items
array that stores the elements of the stack. The class provides several methods to manipulate the stack:
push(element)
: Pushes an element onto the stack by adding it to the end of theitems
array.pop()
: Pops the top element from the stack by removing and returning the last element of theitems
array.peek()
: Returns the top element of the stack without removing it by accessing the last element of theitems
array.isEmpty()
: Checks if the stack is empty by verifying if theitems
array has a length of 0.size()
: Returns the number of elements in the stack by returning the length of theitems
array.clear()
: Clears the stack by setting theitems
array to an empty array.
You can create a new instance of the Stack
class and use its methods to perform stack operations such as pushing, popping, peeking, checking emptiness, getting the size, and clearing the stack.