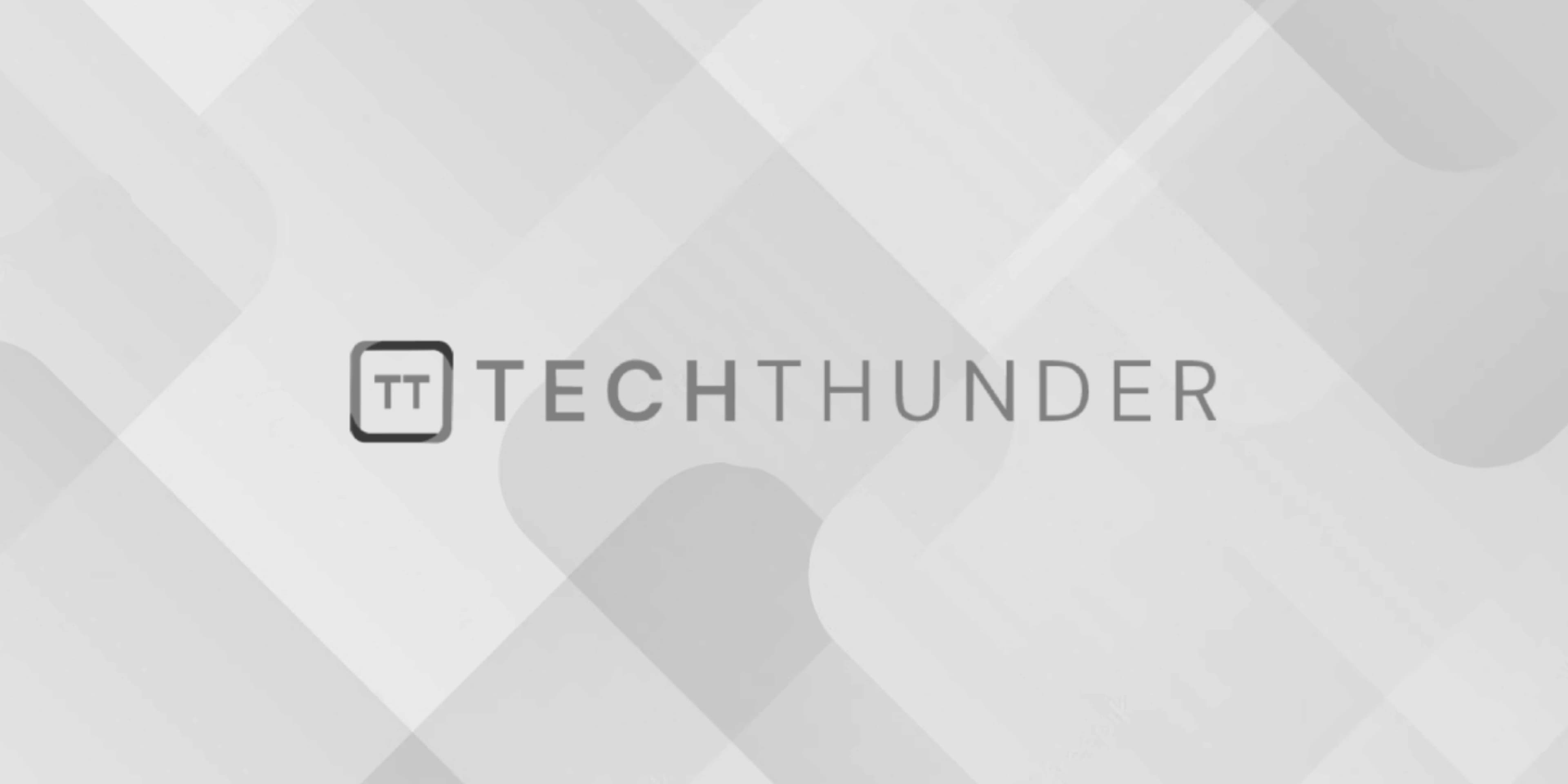
Fibonacci series in JavaScript
The generateFibonacciSeries function takes a parameter n which represents the number of Fibonacci numbers to generate. Example of how you can generate a Fibonacci series in JavaScript using a loop:
function generateFibonacciSeries(n) {
const fibonacciSeries = [0, 1]; // Initial values of Fibonacci series
for (let i = 2; i < n; i++) {
const nextNumber = fibonacciSeries[i - 1] + fibonacciSeries[i - 2];
fibonacciSeries.push(nextNumber);
}
return fibonacciSeries;
}
const n = 10; // Number of Fibonacci numbers to generate
const fibonacciSeries = generateFibonacciSeries(n);
console.log(fibonacciSeries);
In this example, the generateFibonacciSeries
function takes a parameter n
which represents the number of Fibonacci numbers to generate. It initializes the fibonacciSeries
array with the initial values [0, 1]
.
Then, using a for
loop starting from index 2, it calculates the next Fibonacci number by adding the previous two numbers and pushes it into the fibonacciSeries
array.
After the loop finishes, the function returns the fibonacciSeries
array.
In the code snippet, we set n
to 10
to generate the first 10 Fibonacci numbers. You can change the value of n
to generate a different number of Fibonacci numbers.
Finally, we log the fibonacciSeries
array to the console to see the result.
When you run the code, you will get the following output:
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
This is the Fibonacci series with the first 10 numbers.