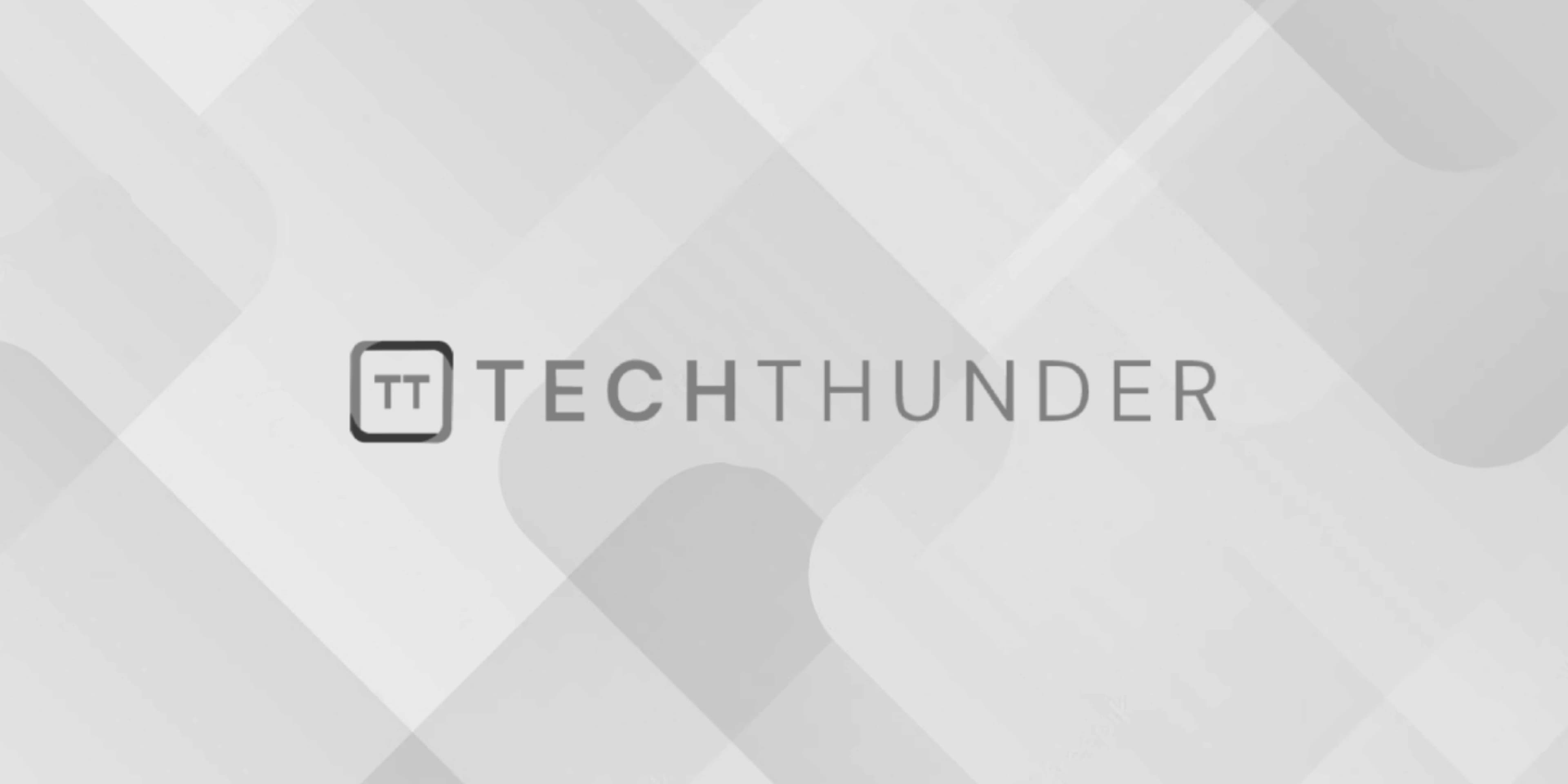
JavaScript scrollIntoView
The scrollIntoView()
method is a built-in method in JavaScript that allows you to scroll a specific element into the viewport. It automatically scrolls the document or container so that the specified element is visible to the user.
Here’s the syntax of the scrollIntoView()
method:
element.scrollIntoView([options]);
element
: The DOM element that you want to scroll into view.options
(optional): An object that specifies the scroll behavior options. It can have the following properties:behavior
: Specifies the scroll behavior. It can be set to'auto'
,'smooth'
, orundefined
. By default, it is set toundefined
.block
: Specifies the vertical alignment of the element in the viewport. It can be set to'start'
,'center'
,'end'
, or'nearest'
. By default, it is set to'start'
.inline
: Specifies the horizontal alignment of the element in the viewport. It can be set to'start'
,'center'
,'end'
, or'nearest'
. By default, it is set to'nearest'
.
Here’s an example usage of the scrollIntoView()
method:
<!DOCTYPE html>
<html>
<head>
<style>
#scrollable {
height: 200px;
overflow: auto;
}
.item {
height: 100px;
margin-bottom: 10px;
background-color: #ddd;
}
</style>
</head>
<body>
<div id="scrollable">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
<div class="item">Item 5</div>
<div class="item">Item 6</div>
<div class="item">Item 7</div>
<div class="item">Item 8</div>
<div class="item">Item 9</div>
<div class="item">Item 10</div>
</div>
<script>
const item = document.getElementById('scrollable').querySelector('.item:last-child');
item.scrollIntoView({ behavior: 'smooth', block: 'end' });
</script>
</body>
</html>
In this example, we have a scrollable container with multiple items. We select the last item using querySelector('.item:last-child')
, and then call the scrollIntoView()
method on that element.
The scrollIntoView()
method is called with an options object specifying behavior: 'smooth'
to enable smooth scrolling and block: 'end'
to align the item to the bottom of the container.
When you run this code, the container will smoothly scroll to make the last item visible at the bottom of the container.
The scrollIntoView()
method is commonly used to scroll to specific elements in a document, such as when implementing navigation or scrolling animations.