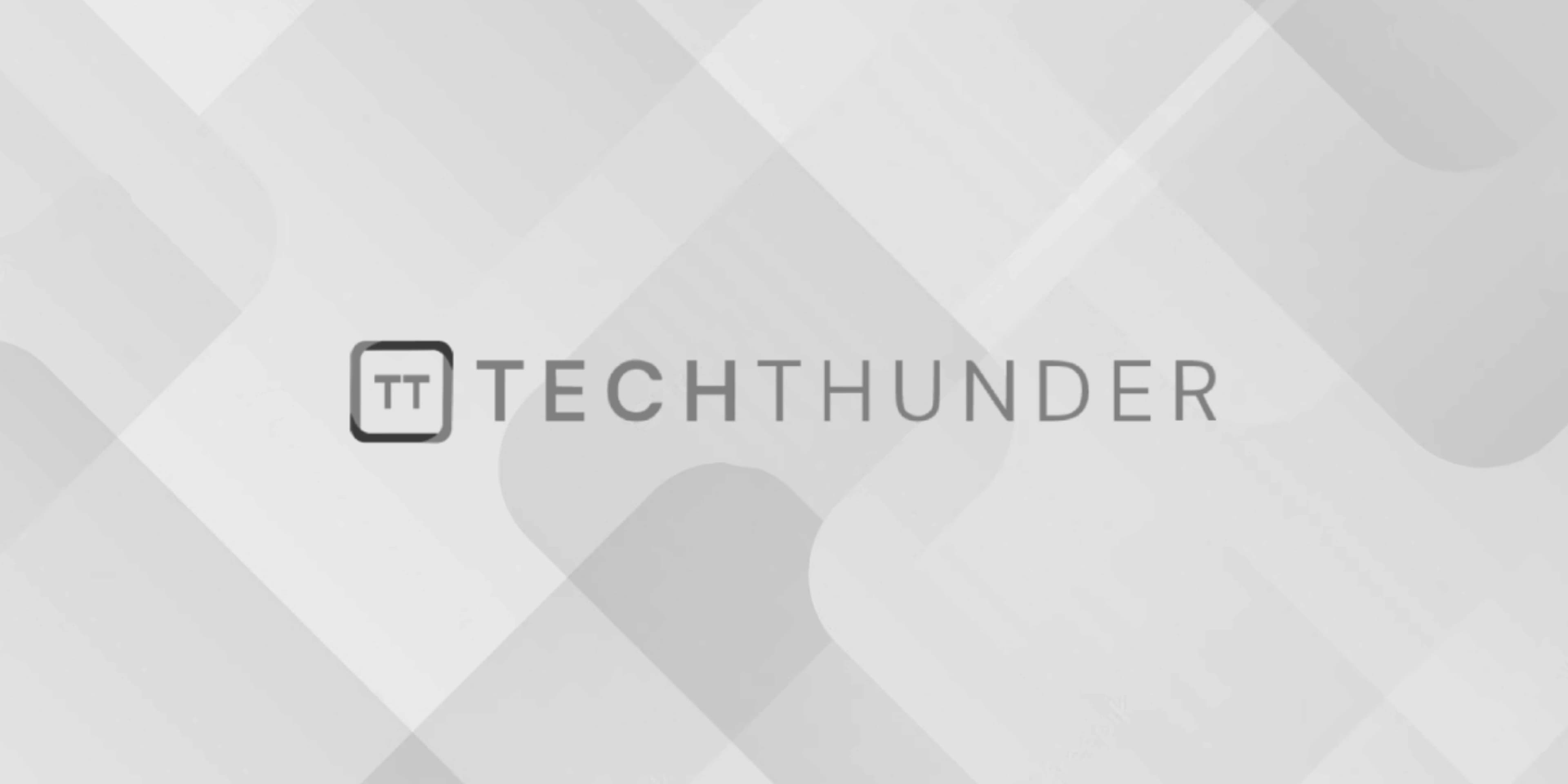
JavaScript try-catch
The JavaScript try-catch
statement is used for error handling. It allows you to execute a block of code and handle any exceptions (errors) that may occur during its execution.
The try
block contains the code that you want to monitor for exceptions. If an exception is thrown within the try
block, the execution of the block is immediately stopped, and the control is passed to the corresponding catch
block.
The syntax for the try-catch
statement is as follows:
try {
// Code that may throw an exception
} catch (error) {
// Code to handle the exception
}
Here’s how it works:
- The code inside the
try
block is executed sequentially. - If an exception occurs within the
try
block, the execution of the block is immediately halted. - The exception is caught by the
catch
block, and the code within thecatch
block is executed. - The
catch
block receives the exception object as a parameter (typically namederror
), which provides information about the error. - After the
catch
block finishes executing, the program continues with the code that follows.
Here’s an example that demonstrates the usage of try-catch
:
try {
// Code that may throw an exception
var result = 10 / 0; // Division by zero error
console.log(result); // This line won't be executed
} catch (error) {
// Code to handle the exception
console.log('An error occurred: ' + error.message);
}
In this example, the code inside the try
block attempts to perform a division by zero, which will throw a TypeError
. Since division by zero is not allowed in JavaScript, an exception occurs. The execution of the try
block is immediately halted, and the control is transferred to the catch
block. The catch
block logs an error message to the console.
Using try-catch
is beneficial for handling unexpected errors and preventing them from crashing your program. It allows you to gracefully handle exceptions and provide appropriate error messages or take necessary actions to recover from the error.