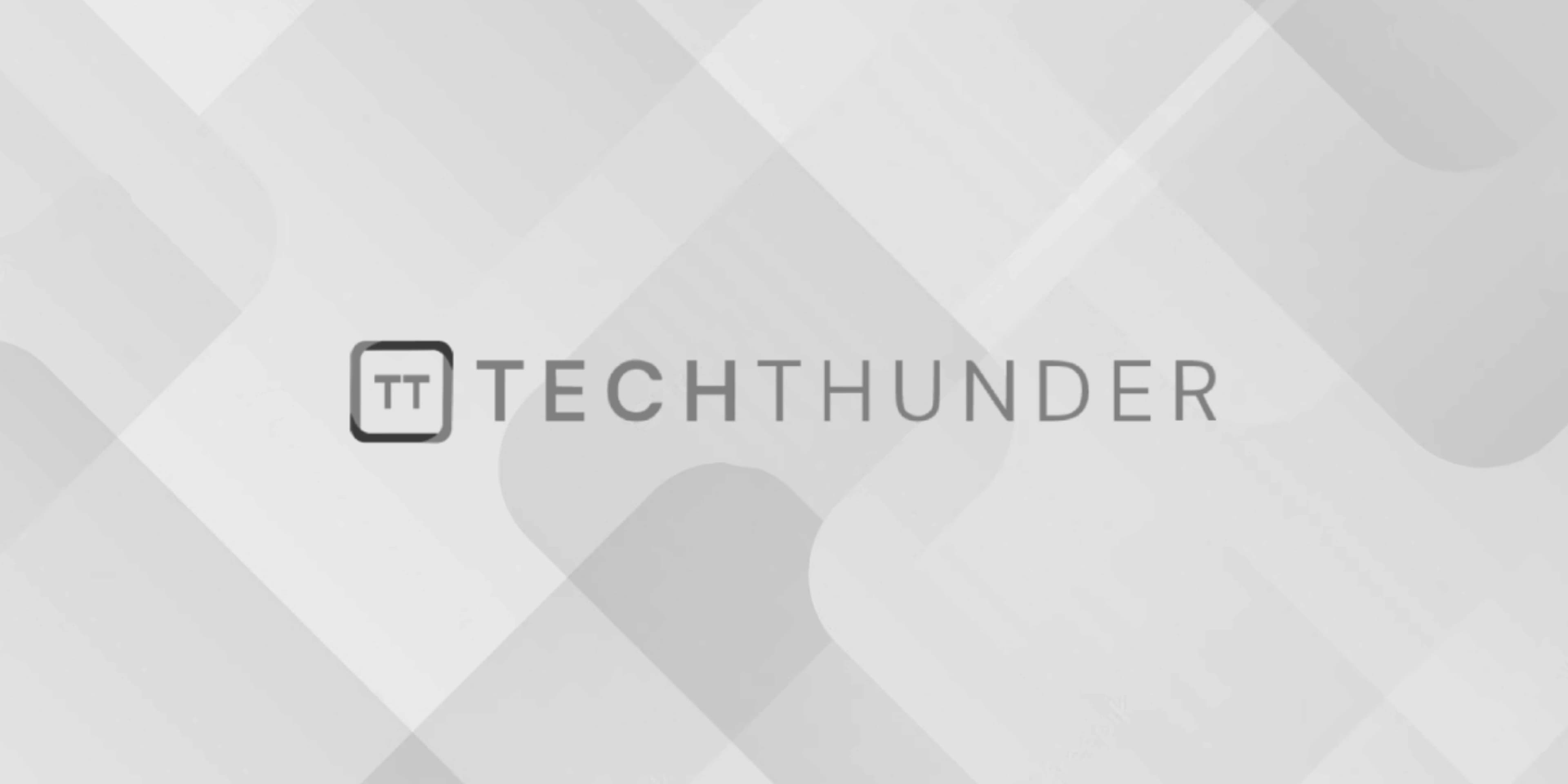
JavaScript Switch
The javascript switch
statement is a conditional statement that evaluates an expression and executes the corresponding case block that matches the value of the expression. It provides a more concise way to write if-else-if chains.
The syntax of the switch statement is as follows:
switch (expression) {
case value1:
// code block to be executed if expression matches value1
break;
case value2:
// code block to be executed if expression matches value2
break;
case value3:
// code block to be executed if expression matches value3
break;
...
default:
// code block to be executed if expression doesn't match any of the values
}
The expression
is evaluated once and its value is compared with each case value. If a match is found, the corresponding block of code is executed. The break
statement is used to terminate the switch statement and prevent fall-through to the next case.
Here is an example of using the switch statement in JavaScript:
let day = new Date().getDay();
let dayName;
switch (day) {
case 0:
dayName = "Sunday";
break;
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Saturday";
break;
default:
dayName = "Unknown";
}
console.log("Today is " + dayName);
In this example, the switch statement evaluates the value of the day
variable and executes the corresponding case block to set the dayName
variable. Finally, the console.log()
statement prints the name of the current day.