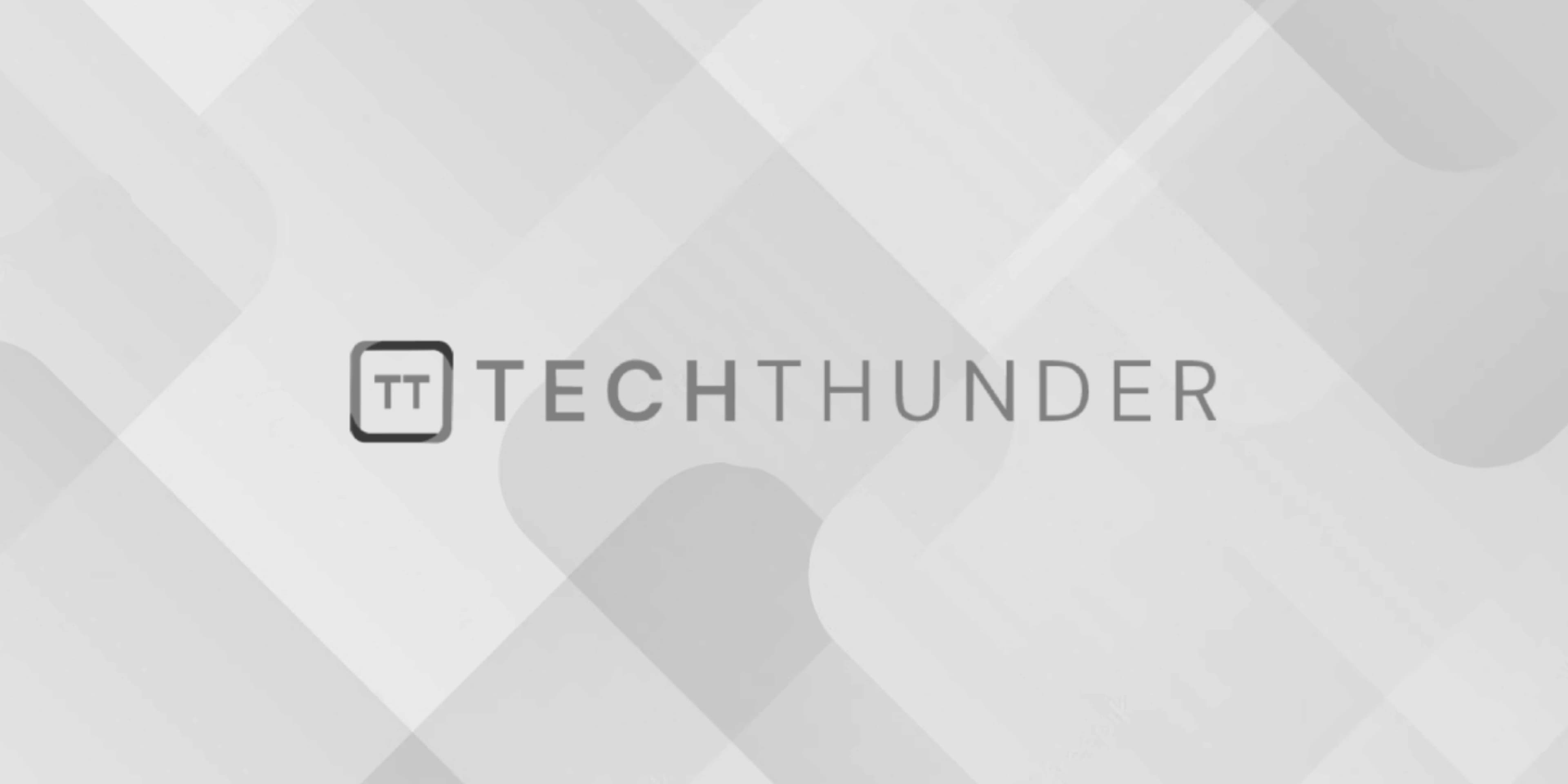
JavaScript ternary operator
The ternary operator, also known as the conditional operator, is a shorthand way to write simple if-else statements in JavaScript. It allows you to write a compact expression that evaluates a condition and returns a value based on the result of that condition.
The syntax of the ternary operator is as follows:
condition ? valueIfTrue : valueIfFalse;
Here’s how it works:
- The
condition
is an expression that evaluates to either true or false. - If the
condition
is true, the expression returnsvalueIfTrue
. - If the
condition
is false, the expression returnsvalueIfFalse
.
Here’s an example to illustrate the usage of the ternary operator:
var age = 25;
var message = age >= 18 ? "You are an adult" : "You are a minor";
console.log(message);
In this example, if the age
is greater than or equal to 18, the condition age >= 18
evaluates to true, and the value "You are an adult"
is assigned to the message
variable. Otherwise, if the condition is false, the value "You are a minor"
is assigned to the message
variable.
The ternary operator can be useful when you need to assign a value based on a simple condition in a concise and readable manner. However, for more complex conditions or multiple branches, it is generally better to use if-else statements for better readability and maintainability.