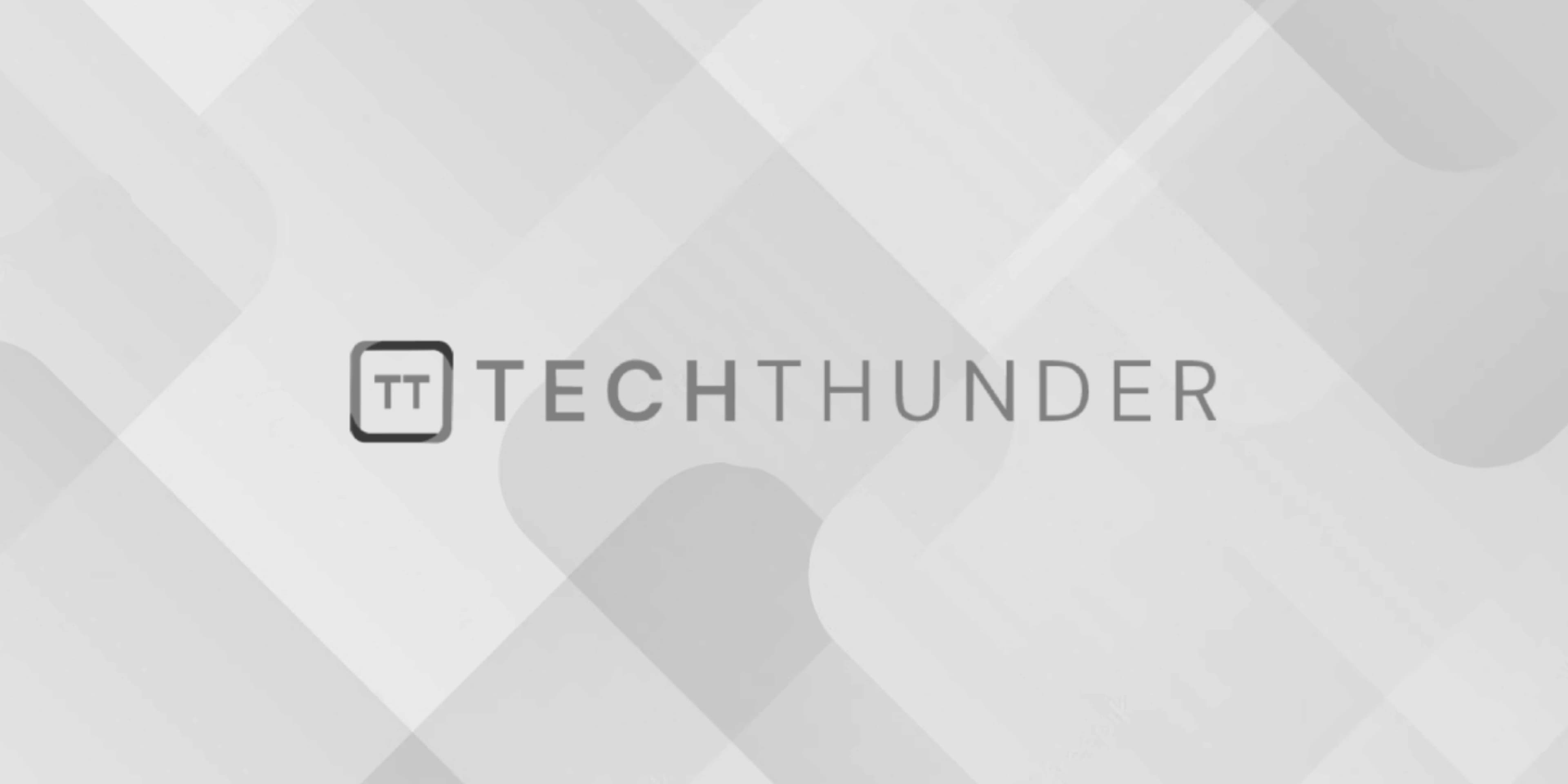
JavaScript for…in Loop
Th for...in
loop is used to iterate over the properties of an object. It allows you to loop through the enumerable properties of an object and perform actions on each property. Here’s the syntax of the for...in
loop:
for (var property in object) {
// code to be executed
}
In each iteration of the loop, the property
variable represents the name of the current property being iterated.
Here’s an example to demonstrate how to use the for...in
loop:
var person = {
name: 'John',
age: 30,
city: 'New York'
};
for (var key in person) {
console.log(key + ': ' + person[key]);
}
In this example, we have an object called person
with properties such as name
, age
, and city
. The for...in
loop iterates over each property of the person
object, and in each iteration, it logs the property name (key
) and its corresponding value (person[key]
) to the console.
The output of the above code will be:
name: John
age: 30
city: New York
It’s important to note that the for...in
loop iterates over all enumerable properties, including properties inherited from the object’s prototype chain. To iterate over only the object’s own properties (excluding inherited properties), you can use the hasOwnProperty()
method within the loop:
for (var key in object) {
if (object.hasOwnProperty(key)) {
// code to be executed
}
}
By using hasOwnProperty()
, you ensure that only properties directly defined on the object itself are processed within the loop.
It’s worth mentioning that the for...in
loop is primarily used for iterating over object properties. If you want to iterate over elements of an array or perform a loop with a specific number of iterations, other loop constructs like for
or forEach
are more suitable.