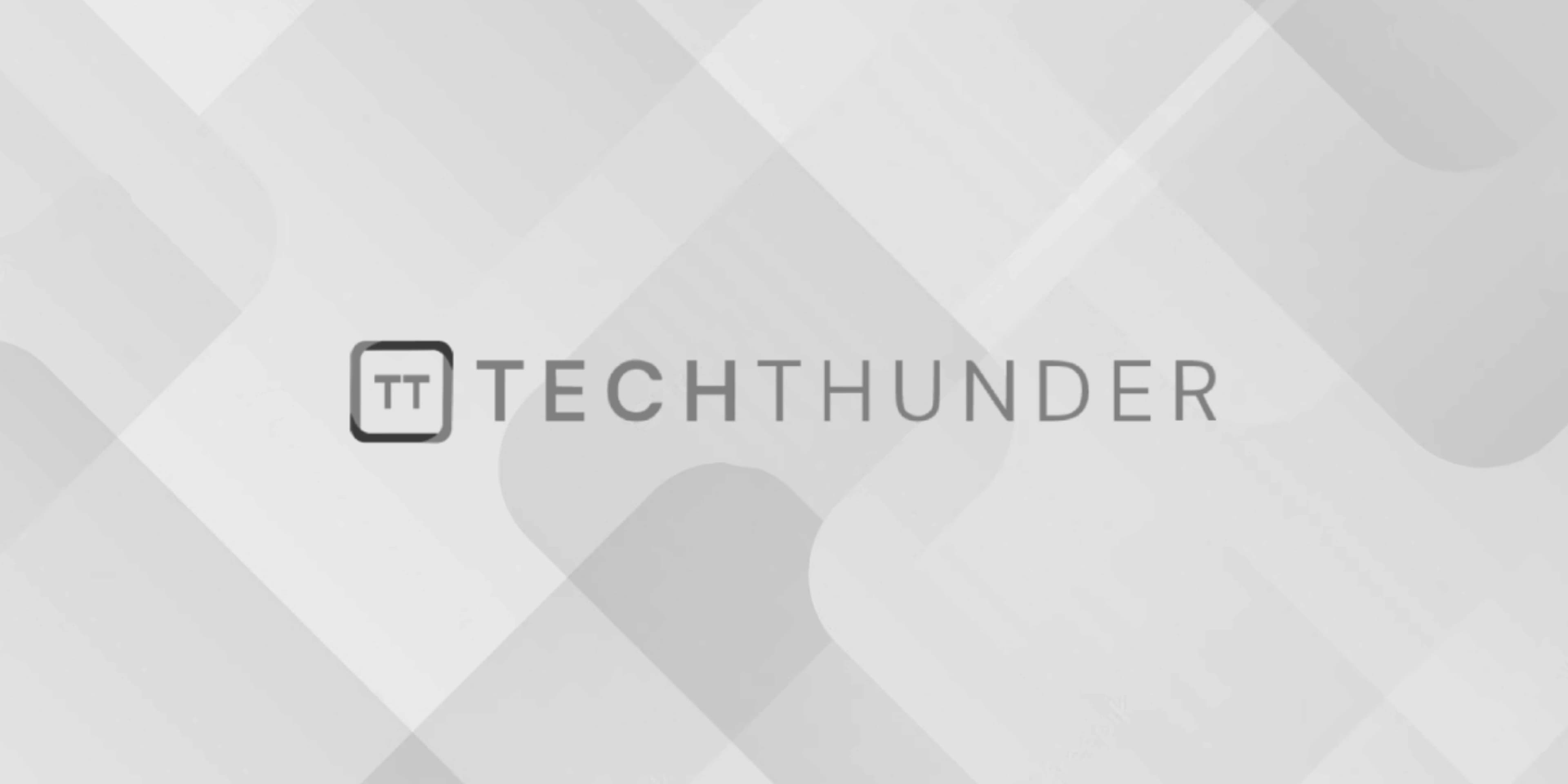
JavaScript return
The JavaScript return
statement is used to end the execution of a function and specify the value to be returned by that function. When the return
statement is encountered, the function will stop executing further code and immediately return the specified value. The returned value can then be assigned to a variable, used in an expression, or passed as an argument to another function.
Here’s an example that demonstrates the use of the return
statement:
function addNumbers(a, b) {
return a + b; // Return the sum of a and b
}
// Call the function and assign the returned value to the 'result' variable
console.log(result); // Output: 8
In this example, the addNumbers()
function takes two arguments a
and b
and returns their sum using the return
statement. The returned value is then assigned to the result
variable and printed to the console.
It’s important to note that once the return
statement is encountered in a function, no further code in that function will be executed. Additionally, a function can have multiple return
statements, but only one will be executed during the function’s execution.