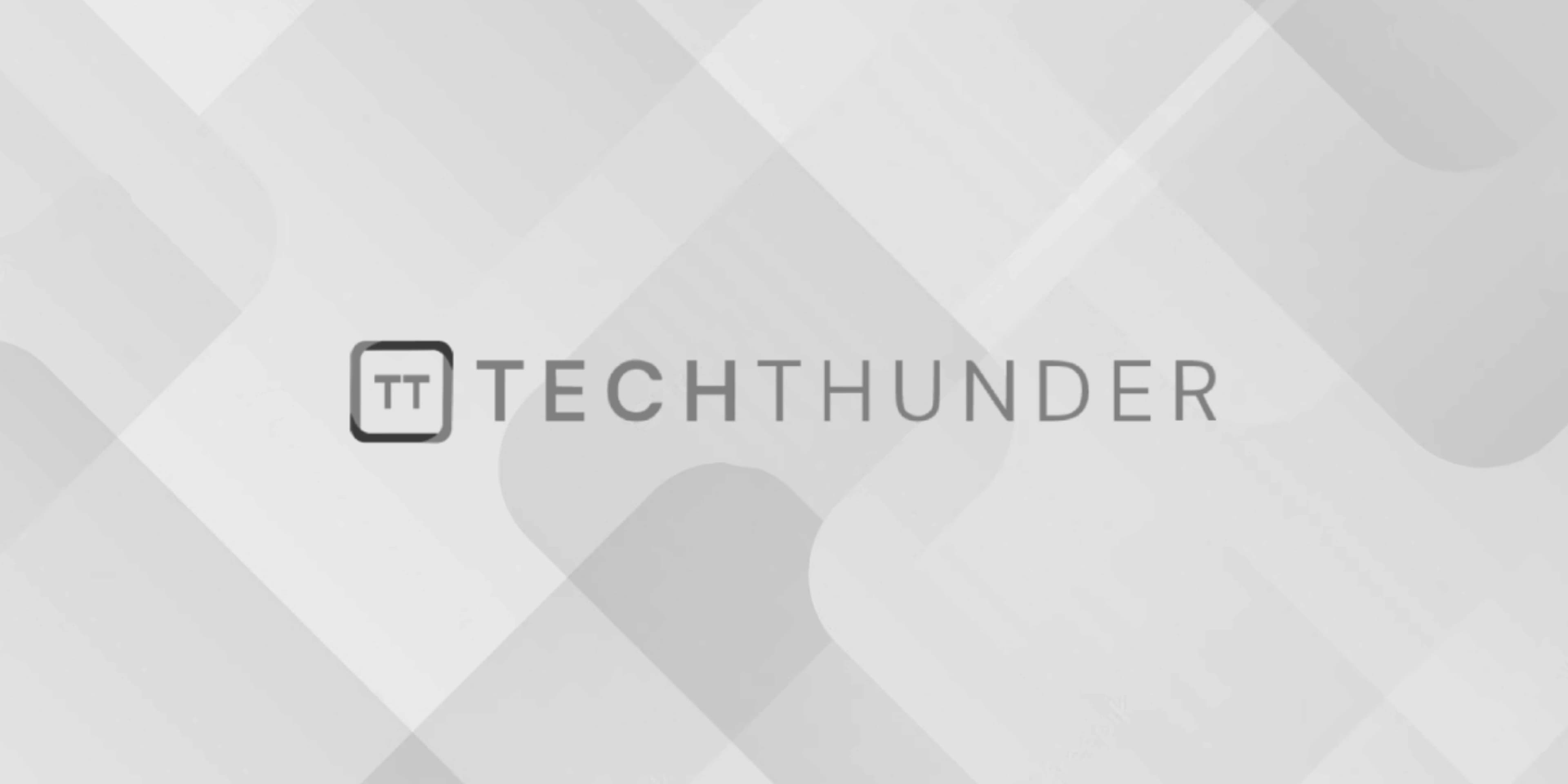
JavaScript Queue
The JavaScript can implement a queue data structure using an array or a linked list. Here’s an example of implementing a queue using an array:
// Queue class
class Queue {
constructor() {
this.items = [];
}
// Add an element to the queue
enqueue(element) {
this.items.push(element);
}
// Remove an element from the front of the queue
dequeue() {
if (this.isEmpty()) {
return null;
}
return this.items.shift();
}
// Get the front element of the queue
front() {
if (this.isEmpty()) {
return null;
}
return this.items[0];
}
// Check if the queue is empty
isEmpty() {
return this.items.length === 0;
}
// Get the size of the queue
size() {
return this.items.length;
}
// Clear the queue
clear() {
this.items = [];
}
}
// Example usage:
var queue = new Queue();
queue.enqueue("A");
queue.enqueue("B");
queue.enqueue("C");
console.log(queue.front()); // Output: A
console.log(queue.dequeue()); // Output: A
console.log(queue.size()); // Output: 2
console.log(queue.isEmpty()); // Output: false
queue.clear();
console.log(queue.isEmpty()); // Output: true
In this example, the Queue
class is defined with various methods to perform queue operations. The items of the queue are stored in an array (this.items
).
The enqueue
method adds an element to the end of the queue using the push
method.
The dequeue
method removes and returns the element from the front of the queue using the shift
method.
The front
method returns the front element of the queue (the first element in the array).
The isEmpty
method checks if the queue is empty by checking the length of the array.
The size
method returns the size (number of elements) of the queue.
The clear
method empties the queue by assigning an empty array to this.items
.
You can create a new Queue
object and use its methods to enqueue, dequeue, access the front element, check the size, check if it’s empty, and clear the queue. The example usage above demonstrates the basic operations.
Note: There are other more efficient ways to implement a queue in JavaScript using a linked list or a circular buffer, but the example above illustrates the basic concept using an array.