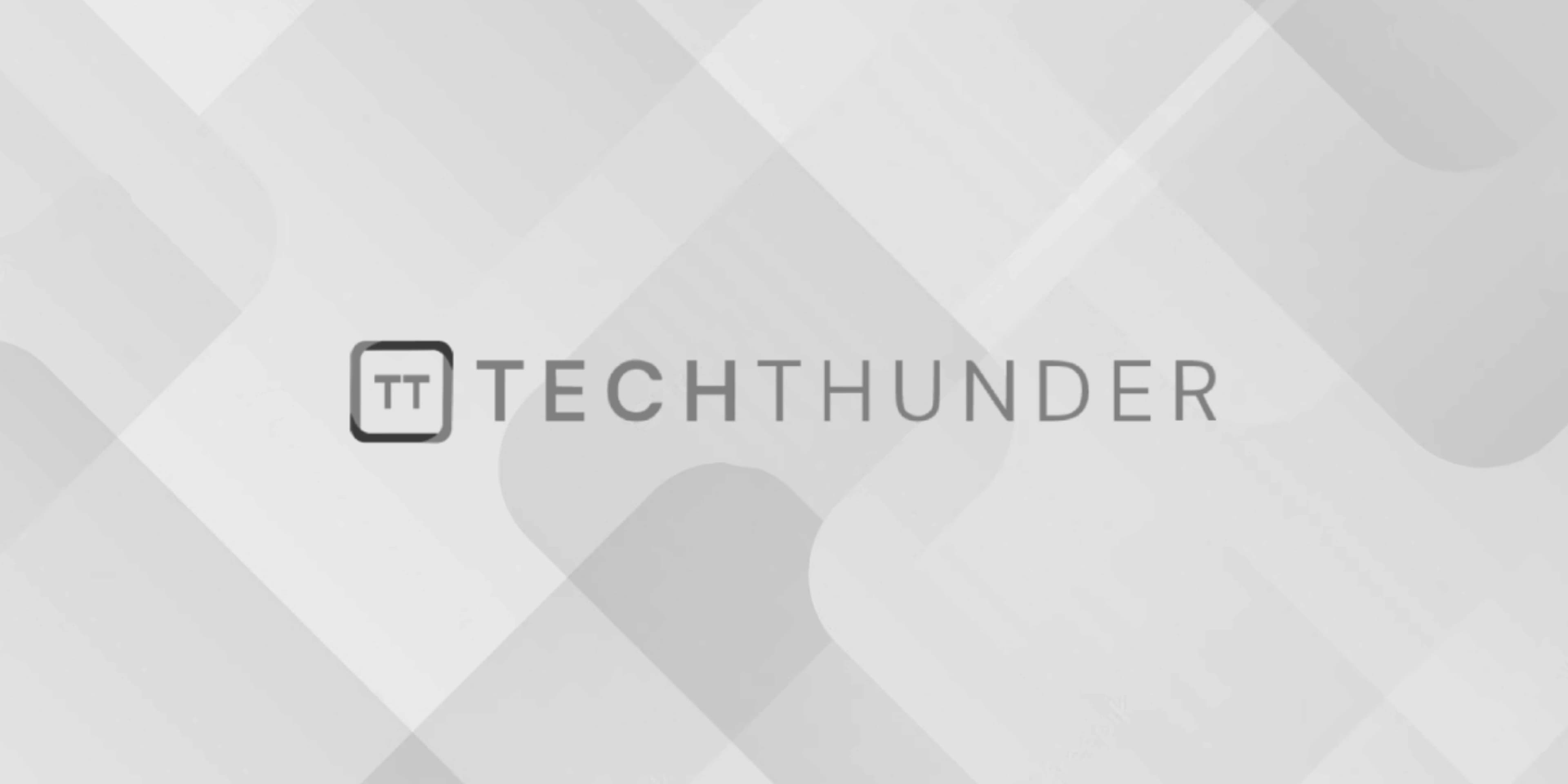
Static vs Const
The JavaScript static
and const
are two different concepts that serve different purposes.
1. static
keyword: In JavaScript, the static
keyword is used in class definitions to define static methods or static properties. Static methods and properties belong to the class itself rather than individual instances of the class. They can be accessed directly on the class without the need to create an instance of the class.
Example of using the static
keyword:
class MyClass {
static staticMethod() {
console.log('This is a static method.');
}
static staticProperty = 'This is a static property.';
}
MyClass.staticMethod(); // Output: "This is a static method."
console.log(MyClass.staticProperty); // Output: "This is a static property."
2. const
keyword: In JavaScript, the const
keyword is used to declare a constant variable, which cannot be re-assigned after it is initialized. It provides a way to define variables with a fixed value that remains constant throughout the code execution.
Example of using the const
keyword:
const PI = 3.14159;
console.log(PI); // Output: 3.14159
// Attempting to re-assign a const variable will result in an error
PI = 3.14; // Error: Assignment to constant variable
The const
keyword is commonly used to declare constants like mathematical values, configuration settings, or any other value that should not be modified during the execution of the code.
In summary, static
is used in class definitions to define static methods and properties, while const
is used to declare constants that cannot be re-assigned.