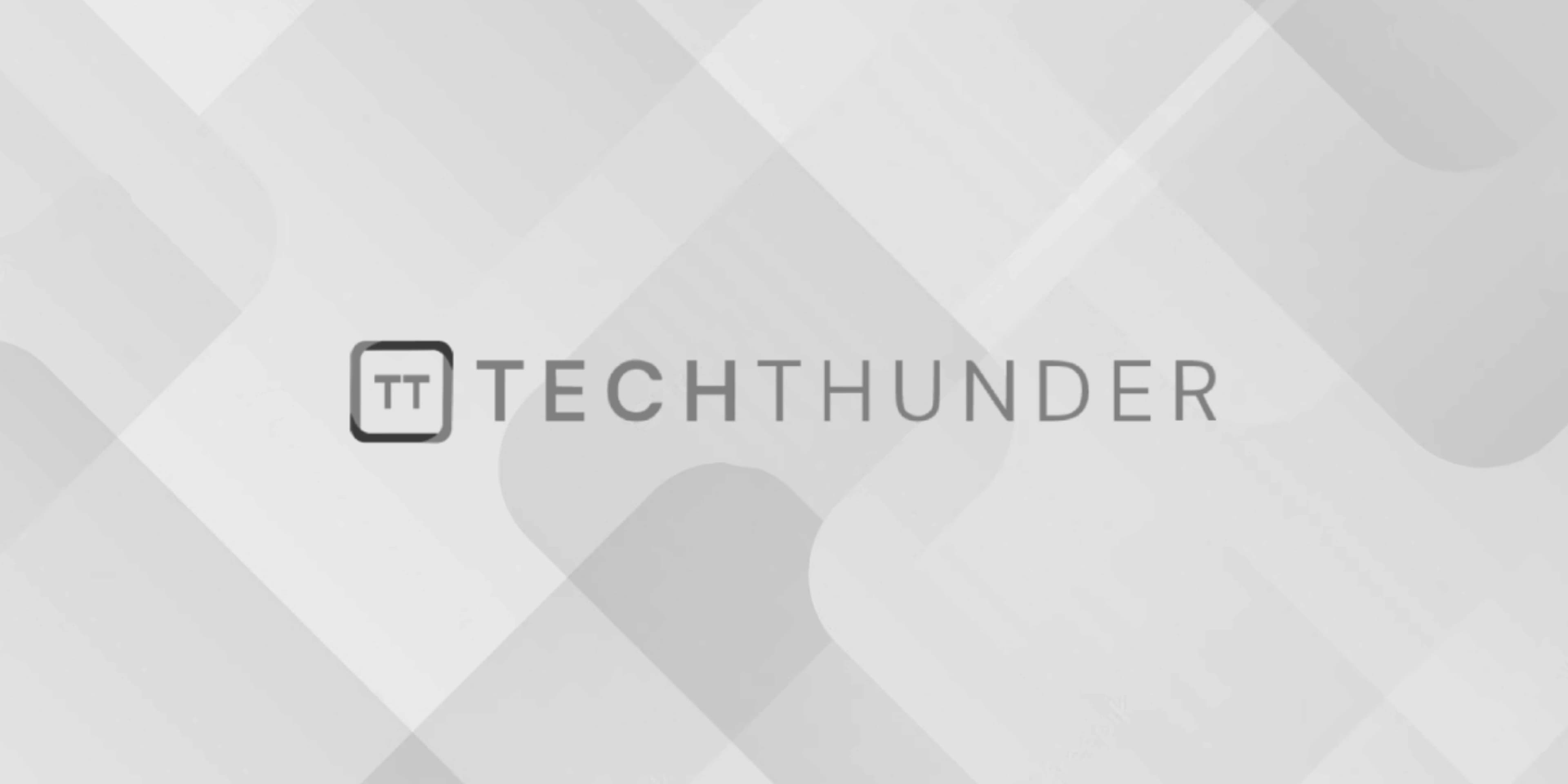
115 views
JavaScript date difference
To calculate the difference between two dates in JavaScript, you can use the Date
object and perform some simple arithmetic calculations. Here’s an example:
JavaScript
function dateDifference(date1, date2) {
// Convert the dates to milliseconds
var date1Ms = date1.getTime();
var date2Ms = date2.getTime();
// Calculate the difference in milliseconds
var differenceMs = Math.abs(date1Ms - date2Ms);
// Convert the difference to days
var differenceDays = Math.floor(differenceMs / (1000 * 60 * 60 * 24));
return differenceDays;
}
// Usage example
var startDate = new Date('2022-01-01');
var endDate = new Date('2022-01-10');
var daysDifference = dateDifference(startDate, endDate);
console.log('Difference in days:', daysDifference);
In the above example, the dateDifference
function accepts two Date
objects as parameters. It converts the dates to milliseconds using the getTime()
method and calculates the absolute difference in milliseconds. Then, it divides the difference by the number of milliseconds in a day to get the difference in days. Finally, it returns the difference in days.
You can modify the example based on your specific requirements, such as calculating the difference in hours, minutes, or seconds by adjusting the division factor accordingly.