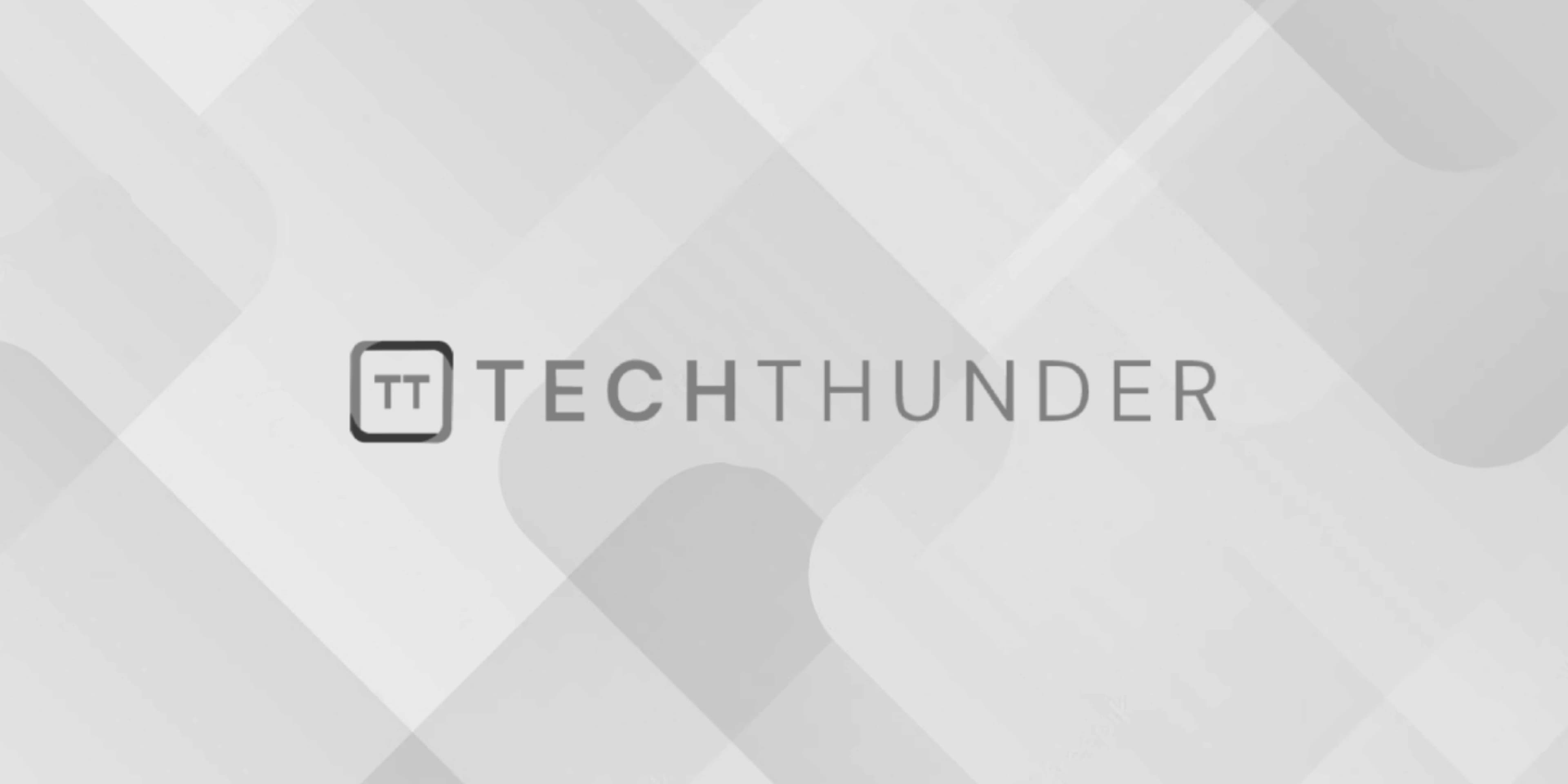
PreviousSibling Property in Javascript
The previousSibling
property in JavaScript is used to retrieve the previous sibling node of a given DOM element. It represents the node immediately preceding the specified element within its parent’s list of child nodes. The previousSibling
property returns the previous sibling as a Node
object or null
if there is no previous sibling.
Here’s an example that demonstrates the usage of the previousSibling
property:
<ul>
<li>Item 1</li>
<li id="target">Item 2</li>
<li>Item 3</li>
</ul>
<script>
const targetElement = document.getElementById("target");
const previousSibling = targetElement.previousSibling;
console.log(previousSibling); // Output: #text
// To skip text nodes and get the previous element sibling, you can use previousElementSibling
const previousElementSibling = targetElement.previousElementSibling;
console.log(previousElementSibling); // Output: <li>Item 1</li>
</script>
In this example, we have an unordered list with three list items. We target the second list item using its id
attribute and store it in the targetElement
variable. We then access its previousSibling
property to get the previous sibling node, which in this case is a text node representing the whitespace between the list items.
To skip text nodes and directly access the previous element sibling, you can use the previousElementSibling
property. This property returns the previous sibling element node, excluding any text nodes or other non-element nodes.
It’s important to note that the previousSibling
and previousElementSibling
properties only consider the immediate preceding sibling. If you need to traverse multiple levels of the DOM tree, you may need to use other methods such as parentNode
and previousSibling
in combination or utilize more advanced DOM traversal techniques.