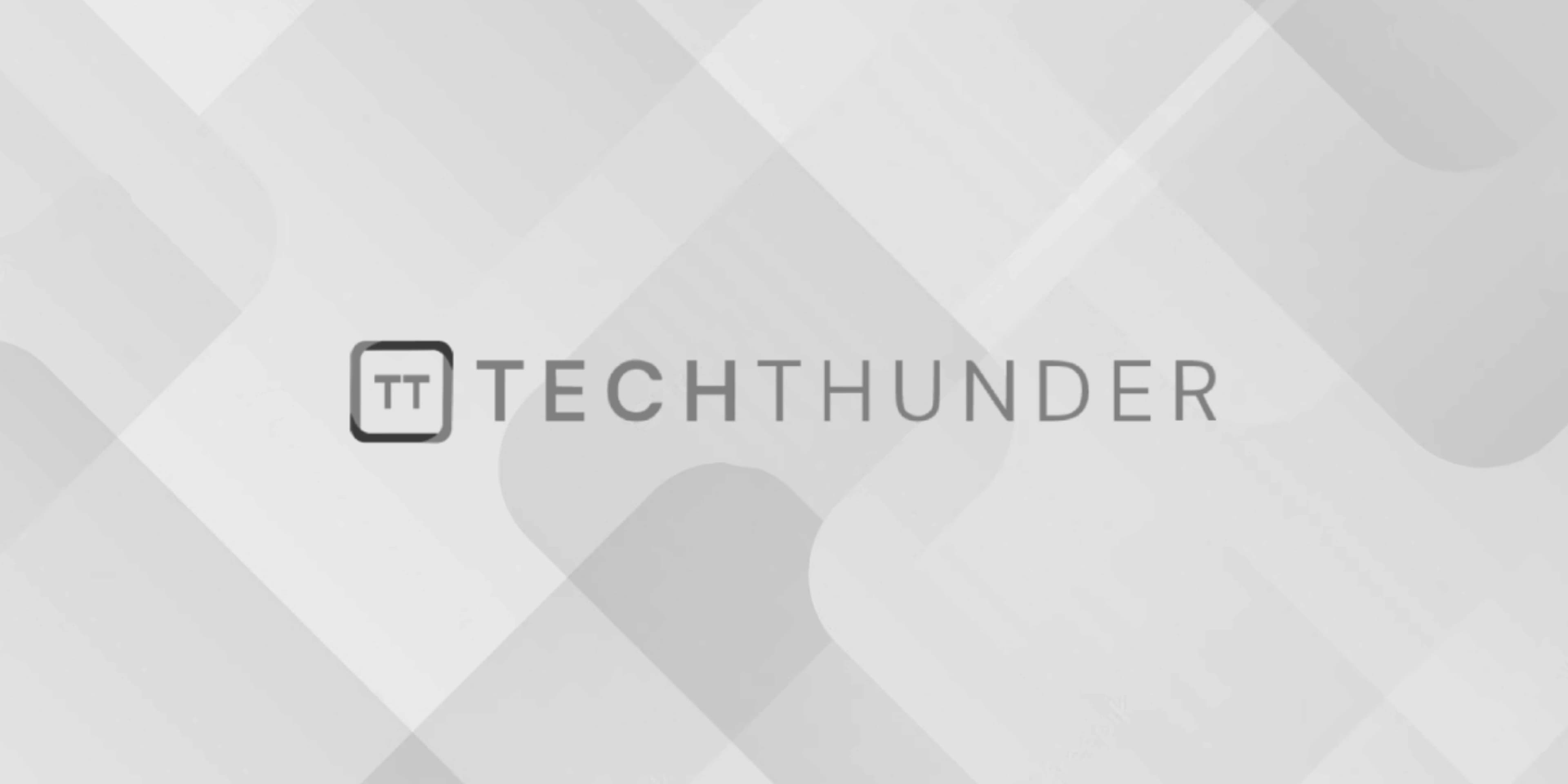
JavaScript onload event
The onload
event is a JavaScript event that occurs when a particular element or the entire document has finished loading. It is commonly used to trigger actions or functions that need to be executed once the page has finished loading.
The onload
event can be attached to various HTML elements, such as the <body>
element or individual <img>
tags, as well as the window
object to handle the entire document’s load event.
Here’s an example of using the onload
event with the <body>
element:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<script>
function onPageLoad() {
// Code to execute when the page loads
console.log('Page loaded!');
}
</script>
</head>
<body onload="onPageLoad()">
<!-- Page content goes here -->
</body>
</html>
In this example, the onload
event is specified on the <body>
element and calls the onPageLoad
function when the page finishes loading. Inside the onPageLoad
function, you can add any JavaScript code or actions that you want to perform once the page is loaded. In this case, it logs a message to the console.
Similarly, you can use the onload
event with other HTML elements, such as <img>
, to trigger actions once specific elements have finished loading. Here’s an example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<script>
function onImageLoad() {
// Code to execute when the image loads
console.log('Image loaded!');
}
</script>
</head>
<body>
<img src="image.jpg" onload="onImageLoad()">
</body>
</html>
In this example, the onload
event is attached to the <img>
element, and the onImageLoad
function is called when the image finishes loading. You can replace image.jpg
with the actual path to your image.
By using the onload
event, you can ensure that specific actions or functions are triggered only after the necessary content has been loaded, providing a more controlled and reliable user experience.