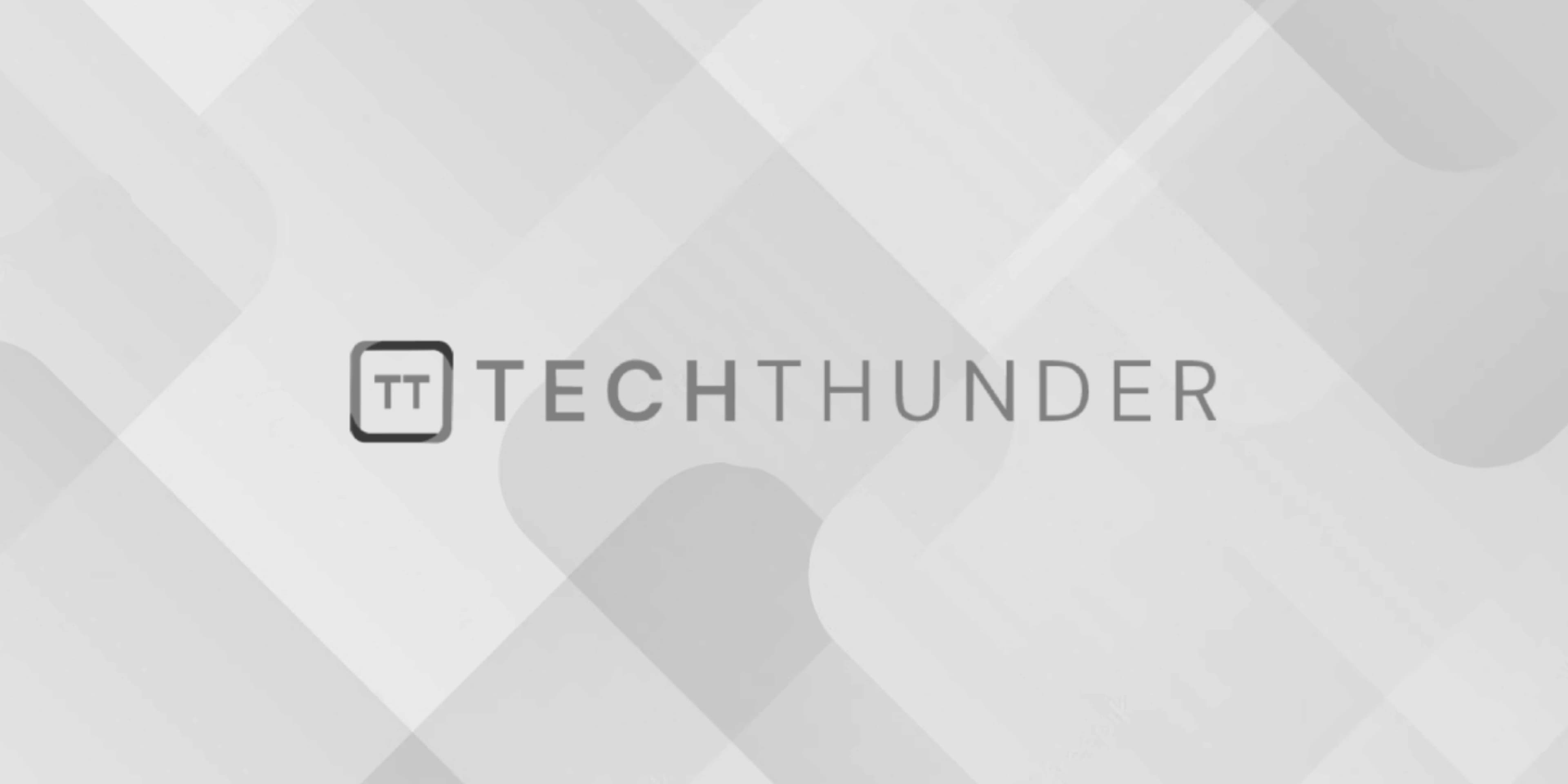
JavaScript insertBefore
The insertBefore()
method in JavaScript is used to insert a specified element as a child element before a reference element. It is commonly used to insert a new element into an existing DOM structure at a specific position.
Here’s the syntax of the insertBefore()
method:
parentNode.insertBefore(newNode, referenceNode);
parentNode
: The parent node where the new element will be inserted as a child.newNode
: The element or node to be inserted.referenceNode
: The existing child element that will serve as the reference point for the insertion. The new element will be inserted before this reference element.
Here’s an example that demonstrates how to use the insertBefore()
method:
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 4</li>
</ul>
<script>
const myList = document.getElementById("myList");
const newItem = document.createElement("li");
newItem.textContent = "Item 3";
const referenceItem = myList.childNodes[2]; // Reference the existing third list item
myList.insertBefore(newItem, referenceItem);
</script>
In this example, we have an unordered list (<ul>
) with three list items (<li>
). We create a new list item (newItem
) using the createElement()
method and set its text content. Then, we select the reference item (referenceItem
) using the childNodes
property and provide the index of the desired position. Finally, we use the insertBefore()
method on the parent node (myList
) to insert the new item before the reference item.
After running this code, the new list item with the text content “Item 3” will be inserted before the existing third list item, resulting in the updated list:
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
Note that the insertBefore()
method allows you to dynamically insert elements at a specific position within a parent element. It gives you control over the ordering and placement of elements in the DOM structure.