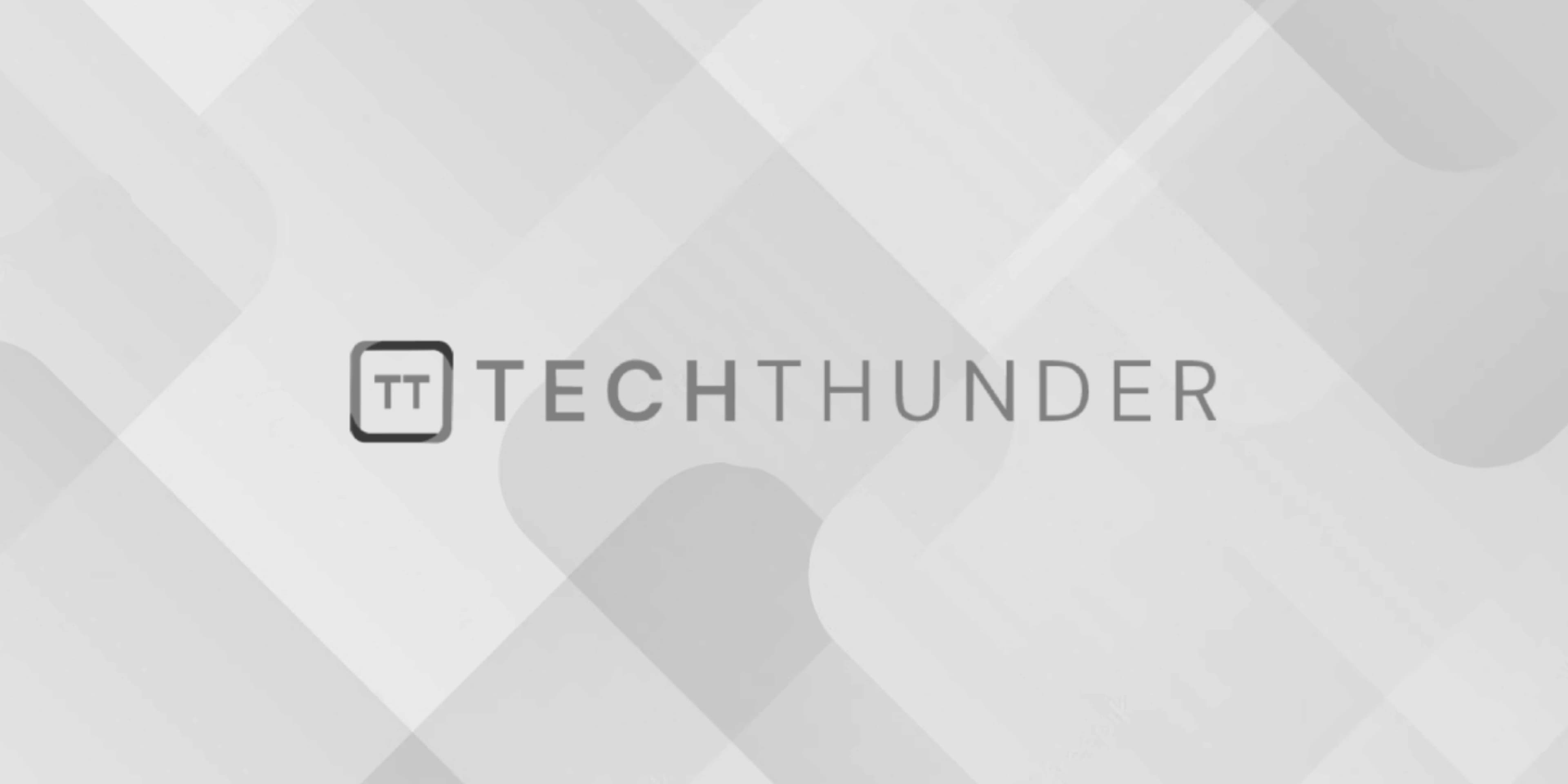
JavaScript MutationObserver function
The MutationObserver is a JavaScript API that allows you to listen for changes in the DOM (Document Object Model) and be notified when specific mutations occur. It provides a way to observe and react to changes in elements, attributes, or the structure of the DOM tree. Here’s an example of how to use the MutationObserver function:
// Select the target node to observe
var targetNode = document.getElementById('myElement');
// Options for the observer (optional)
var observerOptions = {
attributes: true, // Observe changes to attributes
childList: true, // Observe changes to child elements
subtree: true, // Observe changes in all descendants of the target node
// ... other options if needed
};
// Create a new instance of the MutationObserver
var observer = new MutationObserver(callback);
// Define the callback function to be executed when mutations occur
function callback(mutationsList, observer) {
// Handle the mutations here
for (var mutation of mutationsList) {
if (mutation.type === 'attributes') {
console.log('Attribute changed: ', mutation.attributeName);
} else if (mutation.type === 'childList') {
console.log('Child list changed');
}
// ... handle other types of mutations if needed
}
}
// Start observing the target node with the specified options
observer.observe(targetNode, observerOptions);
// To stop observing, call observer.disconnect():
// observer.disconnect();
In this example, we first select the target node that we want to observe using document.getElementById()
. The targetNode
can be any element in the DOM.
Then, we define the observerOptions
object, which specifies the types of mutations to observe. In this case, we set attributes
to true
to observe changes to attributes, childList
to true
to observe changes to child elements, and subtree
to true
to observe changes in all descendants of the target node. You can modify these options according to your specific needs.
Next, we create a new instance of the MutationObserver
by passing the callback function to be executed when mutations occur.
The callback
function is called with two parameters: mutationsList
and observer
. The mutationsList
contains an array of MutationRecord
objects, which represent the specific mutations that occurred. In the example, we iterate through the mutationsList
and log the type of mutation (either attributes
or childList
) to the console.
Finally, we start observing the target node by calling the observe()
method of the MutationObserver
instance, passing in the targetNode
and observerOptions
.
To stop observing, you can call observer.disconnect()
.
By using the MutationObserver function, you can monitor and respond to changes in the DOM dynamically.