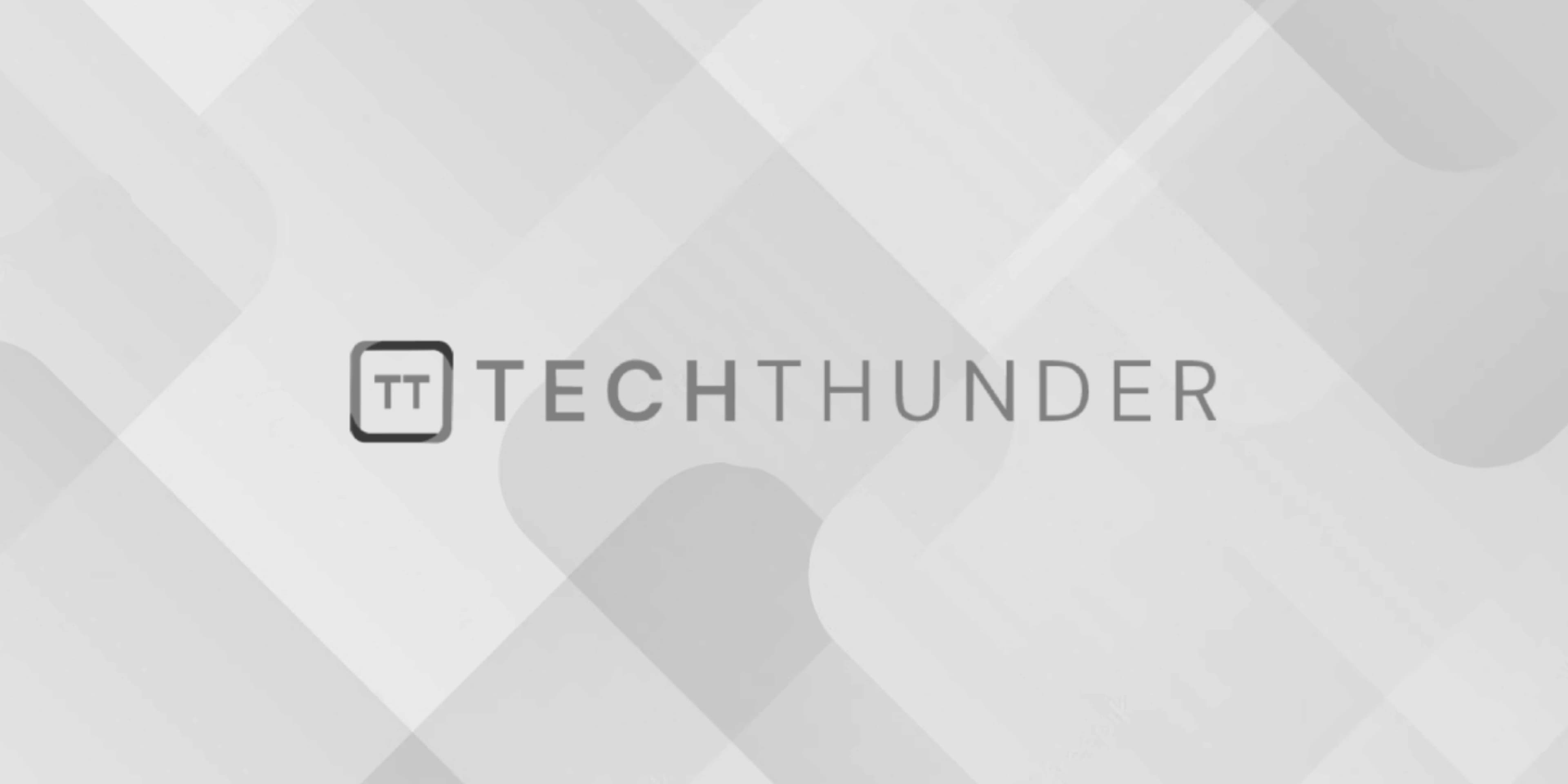
JavaScript Animation
In JavaScript to create animations using various techniques and libraries. Here, I’ll provide you with an example of creating a simple animation using the built-in requestAnimationFrame
function.
The requestAnimationFrame
function is a browser API that allows you to schedule a callback to be executed before the next repaint. This provides a smoother and more efficient way to perform animations compared to using a regular interval-based approach.
Here’s an example of a basic animation that moves an element horizontally across the screen:
var element = document.getElementById('myElement');
var position = 0;
var velocity = 2; // Number of pixels to move per frame
function animate() {
// Update the position
position += velocity;
// Apply the new position to the element
element.style.left = position + 'px';
// Check if animation should continue
if (position < window.innerWidth) {
// Request the next animation frame
requestAnimationFrame(animate);
}
}
// Start the animation
animate();
In this example, we select an HTML element with the ID myElement
and initialize a position
variable to track its position. The velocity
variable determines the number of pixels to move per frame.
The animate
function is called recursively using requestAnimationFrame
. Inside the function, we update the position
variable based on the velocity and apply the new position to the element’s left
CSS property. We then check if the element is still within the window’s width. If so, we request the next animation frame to continue the animation.
You can customize this example or explore more advanced animation techniques using CSS transitions, CSS animations, or JavaScript libraries like GreenSock (GSAP) or anime.js, which provide more features and control over animations.
Remember to handle cross-browser compatibility and consider performance optimization techniques when working with animations in JavaScript.