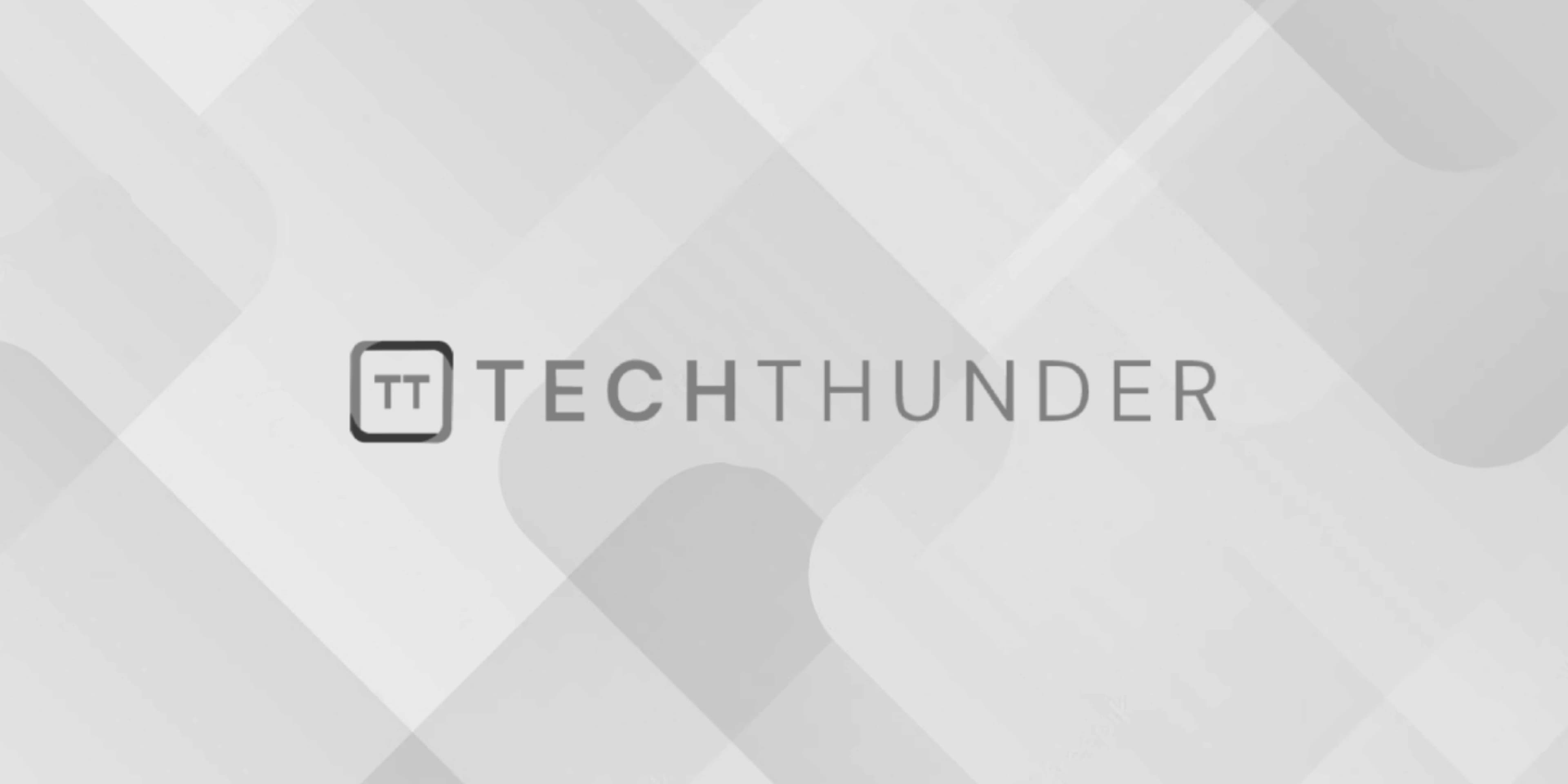
123 views
Remove elements from array
To remove elements from an array in JavaScript, you can use various array methods. Here are a few common methods you can use:
splice()
: Thesplice()
method can be used to remove elements from an array by specifying the starting index and the number of elements to remove. It also allows you to add new elements in place of the removed elements. Here’s an example:
JavaScript
let fruits = ['apple', 'banana', 'orange', 'mango'];
fruits.splice(1, 2); // Removes 'banana' and 'orange' from the array
console.log(fruits); // Output: ['apple', 'mango']
pop()
: Thepop()
method removes the last element from an array and returns that element. It modifies the original array. Here’s an example:
JavaScript
let fruits = ['apple', 'banana', 'orange', 'mango'];
let removedElement = fruits.pop(); // Removes 'mango' from the array and returns it
console.log(fruits); // Output: ['apple', 'banana', 'orange']
console.log(removedElement); // Output: 'mango'
shift()
: Theshift()
method removes the first element from an array and returns that element. It also modifies the original array. Here’s an example:
JavaScript
let fruits = ['apple', 'banana', 'orange', 'mango'];
let removedElement = fruits.shift(); // Removes 'apple' from the array and returns it
console.log(fruits); // Output: ['banana', 'orange', 'mango']
console.log(removedElement); // Output: 'apple'
These are just a few examples, and there are other array methods like filter()
, slice()
, etc., that can be used to remove elements from an array based on specific conditions. The choice of method depends on your specific requirements.