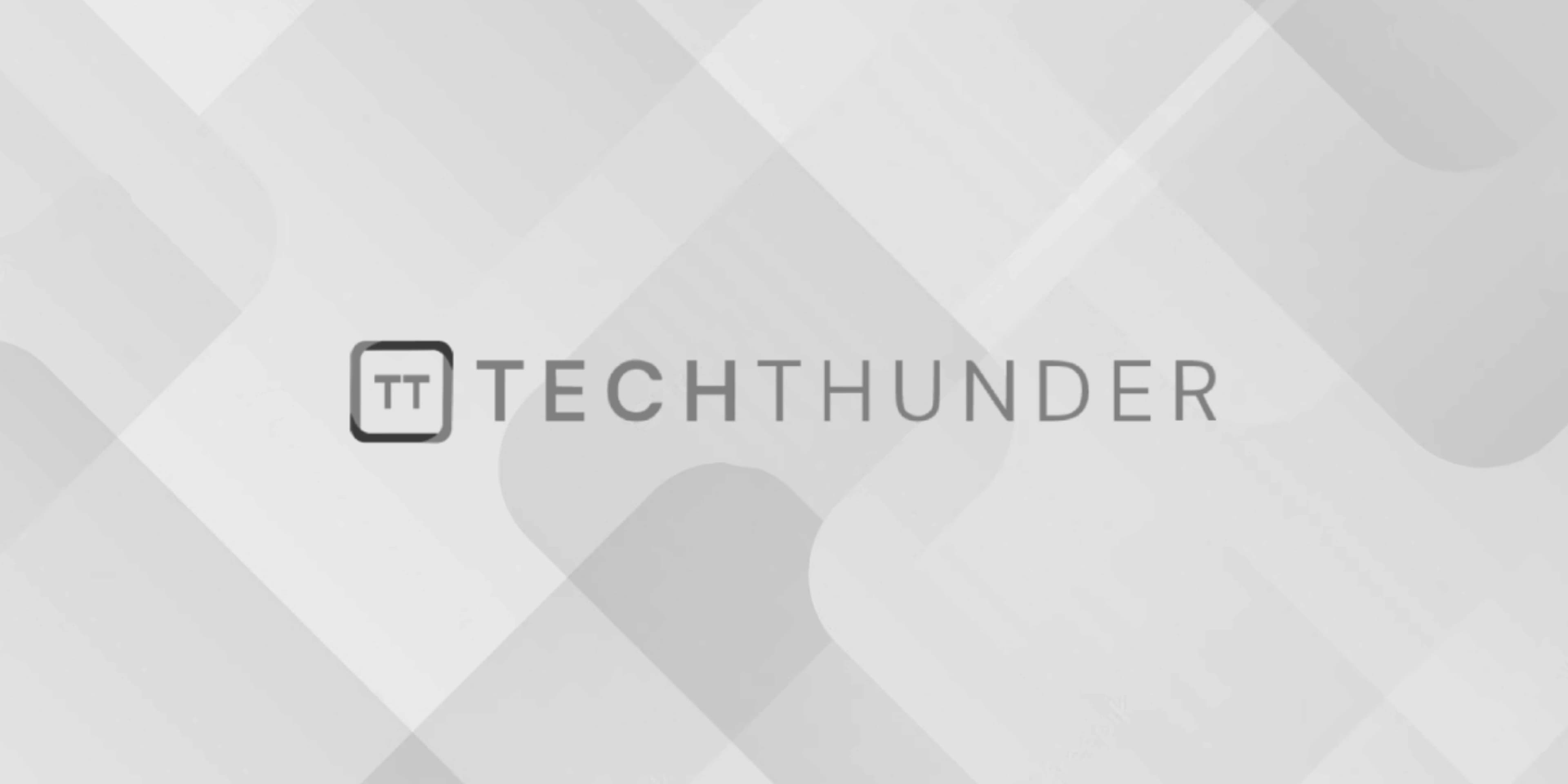
Javascript – document.getElementsByName()
The JavaScript document.getElementsByName()
method is used to get a collection of all the elements in the document with a specific name attribute. It returns a NodeList
object, which is an ordered collection of elements with the same name attribute.
The getElementsByName()
method takes a name attribute value as its parameter and returns a NodeList
object that contains all the elements in the document with the specified name attribute.
The getElementsByName()
method is called with the argument “myInput” to get all the <input>
elements with the name attribute “myInput” in the document. The returned NodeList
object is then logged to the console, which will output an array-like object containing all the matching <input>
elements in the document.
Note that the NodeList
object is not a true array and therefore cannot be manipulated using array methods, but it can be looped over using a for
loop or converted to an array using Array.from()
.
Here is an example of how to use getElementsByName()
method to get all the <input>
elements with the name attribute “myInput” in an HTML document:
<html>
<head>
<title>Example</title>
</head>
<body>
<input type="text" name="myInput">
<input type="text" name="myInput">
<script>
const inputs = document.getElementsByName("myInput");
console.log(inputs); // Output: NodeList[<input>, <input>]
</script>
</body>
</html>