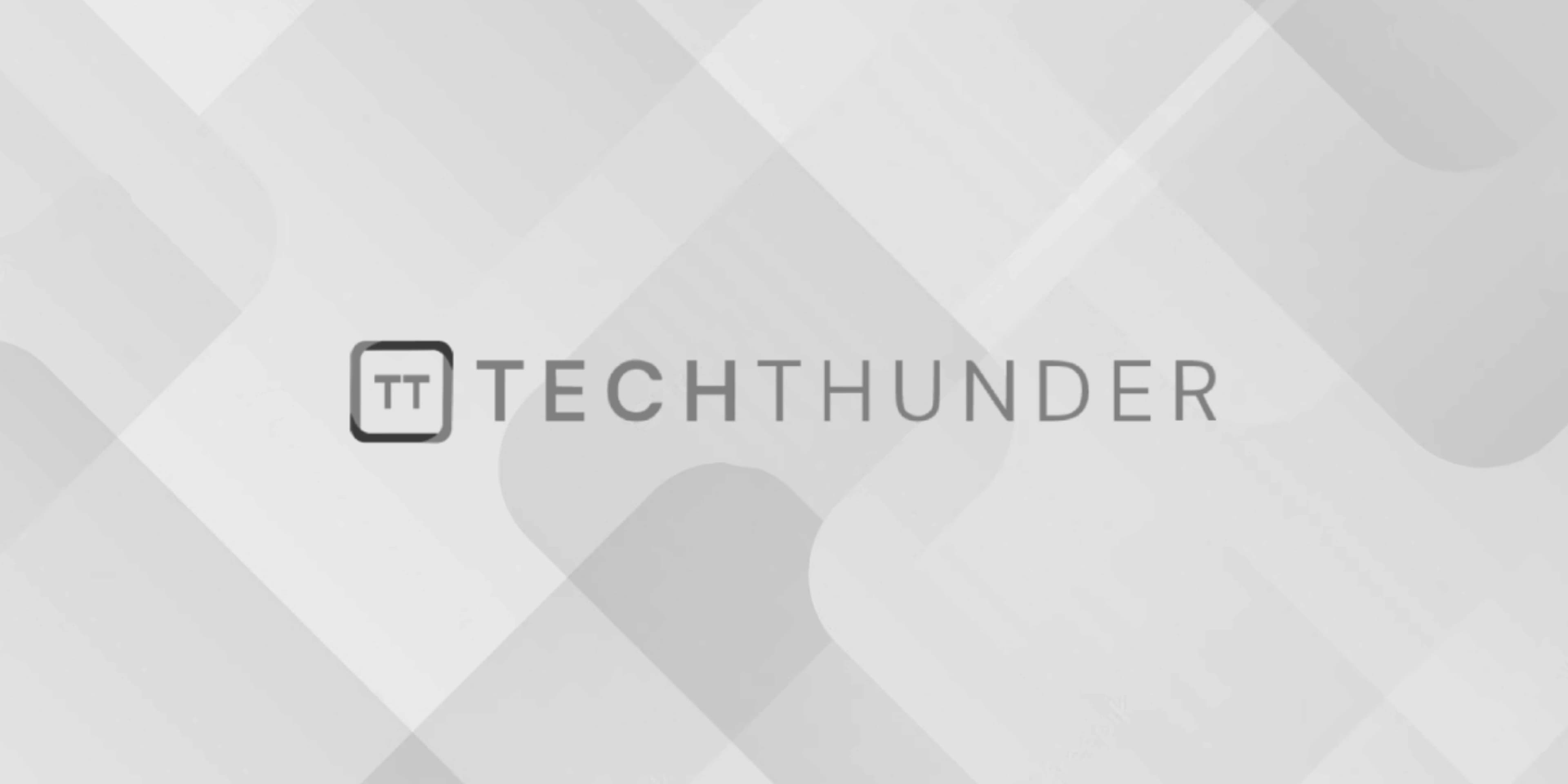
119 views
JavaScript localStorage
JavaScript localStorage
is a web storage mechanism that allows you to store key-value pairs locally in the user’s web browser. It provides a way to persistently store data on the client-side, even after the browser is closed and reopened.
Here’s an overview of how to use localStorage
in JavaScript:
- Storing Data:
To store data inlocalStorage
, you can use thesetItem()
method. It takes two parameters: a key and a value.
JavaScript
localStorage.setItem('key', 'value');
- Retrieving Data:
To retrieve data fromlocalStorage
, you can use thegetItem()
method. It takes the key as a parameter and returns the corresponding value.
JavaScript
const value = localStorage.getItem('key');
- Updating Data:
To update the value of a stored item inlocalStorage
, you can simply callsetItem()
again with the same key.
JavaScript
localStorage.setItem('key', 'new value');
- Removing Data:
To remove an item fromlocalStorage
, you can use theremoveItem()
method. It takes the key as a parameter.
JavaScript
localStorage.removeItem('key');
- Clearing Data:
To remove all items fromlocalStorage
, you can use theclear()
method.
JavaScript
localStorage.clear();
- Checking for Item Existence:
You can check if a specific item exists inlocalStorage
by using thegetItem()
method and checking if the returned value is notnull
.
JavaScript
const exists = localStorage.getItem('key') !== null;
It’s important to note that the data stored in localStorage
is persistent and will remain available even after the browser is closed and reopened. However, it is limited to the specific domain and protocol of the web page.
Also, keep in mind that the data stored in localStorage
is accessible to JavaScript code running in the same domain, so avoid storing sensitive or confidential information in localStorage
.