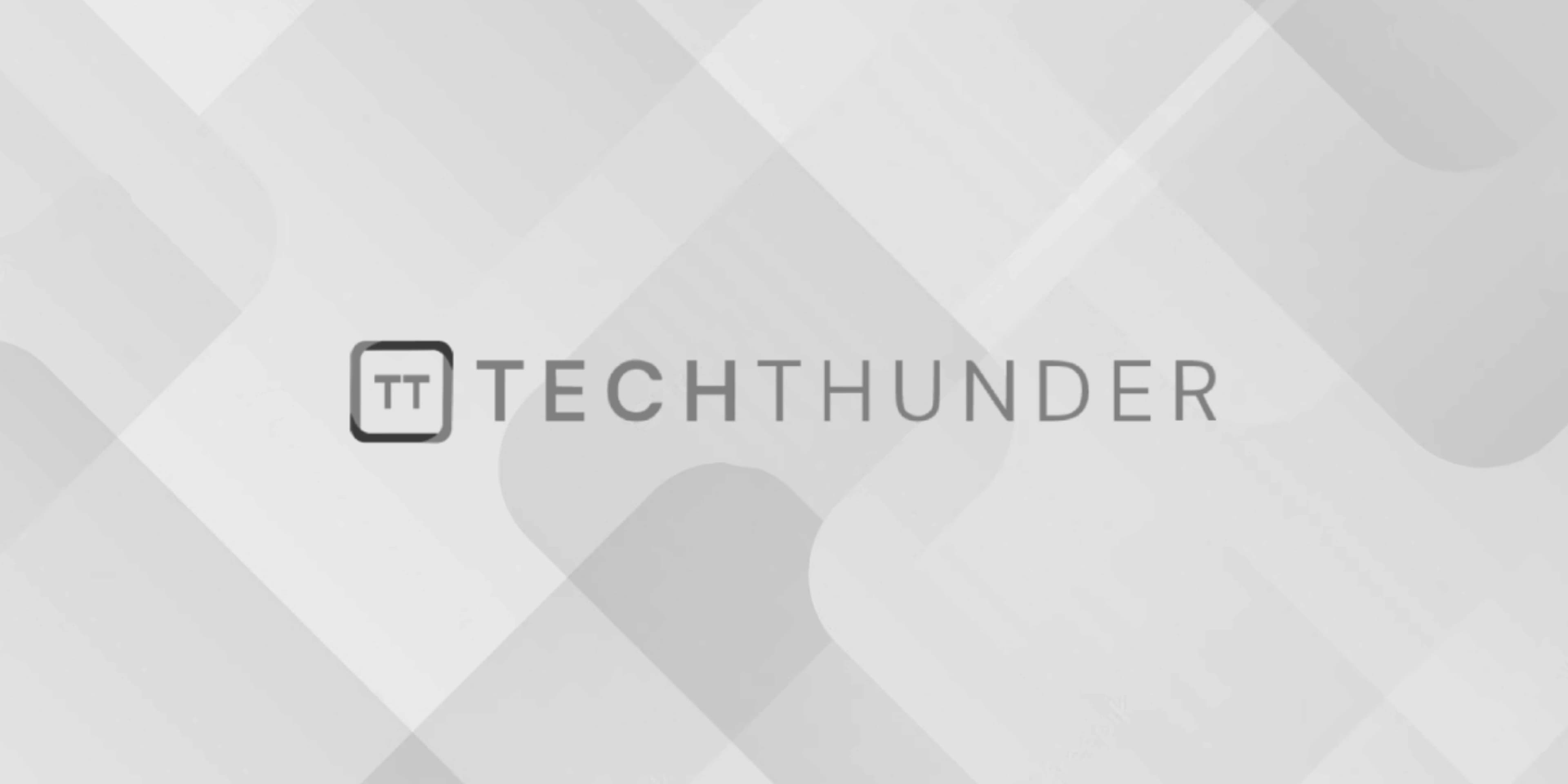
90 views
Check if the value exists in Array in Javascript
To check if a value exists in an array in JavaScript, you can use various methods such as Array.prototype.includes()
, Array.prototype.indexOf()
, or Array.prototype.find()
. Here are examples of each method:
- Using
includes()
method:
JavaScript
var array = [1, 2, 3, 4, 5];
var value = 3;
if (array.includes(value)) {
console.log("Value exists in the array");
} else {
console.log("Value does not exist in the array");
}
- Using
indexOf()
method:
JavaScript
var array = [1, 2, 3, 4, 5];
var value = 3;
if (array.indexOf(value) !== -1) {
console.log("Value exists in the array");
} else {
console.log("Value does not exist in the array");
}
- Using
find()
method:
JavaScript
var array = [1, 2, 3, 4, 5];
var value = 3;
var found = array.find(function(element) {
return element === value;
});
if (found !== undefined) {
console.log("Value exists in the array");
} else {
console.log("Value does not exist in the array");
}
In all of these examples, we have an array (array
) and a value (value
) that we want to check for existence in the array. If the value is found, it means it exists in the array, and we can perform the desired actions. If the value is not found, it means it does not exist in the array.