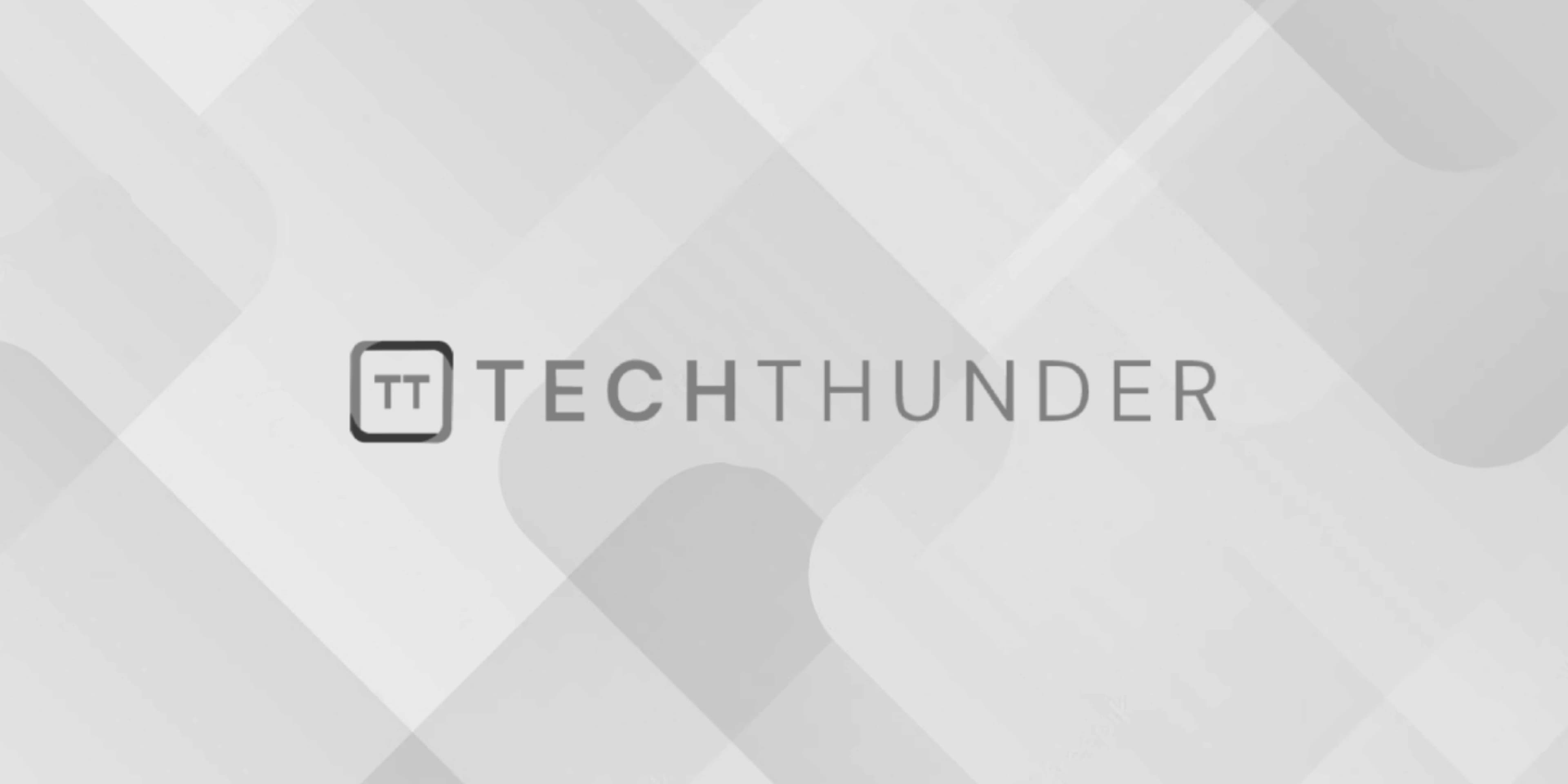
JavaScript If statement
The javascript if
statement is used for conditional branching. It allows the code to make a decision based on a condition and execute different parts of the code depending on whether the condition is true or false.
Here is an example of using an if
statement in JavaScript:
let num = 5;
if (num > 0) {
console.log("Number is positive");
}
In this example, the if
statement checks whether the value of num
is greater than 0. If the condition is true, the code inside the curly braces will be executed, which in this case is to print “Number is positive” to the console. If the condition is false, the code inside the curly braces will be skipped.
We can also add an else
block to execute a different block of code if the condition is false:
let num = -3;
if (num > 0) {
console.log("Number is positive");
} else {
console.log("Number is negative");
}
In this example, if the condition is true, the code inside the first block will be executed, otherwise the code inside the else
block will be executed. In this case, since the value of num
is less than 0, the output will be “Number is negative”.