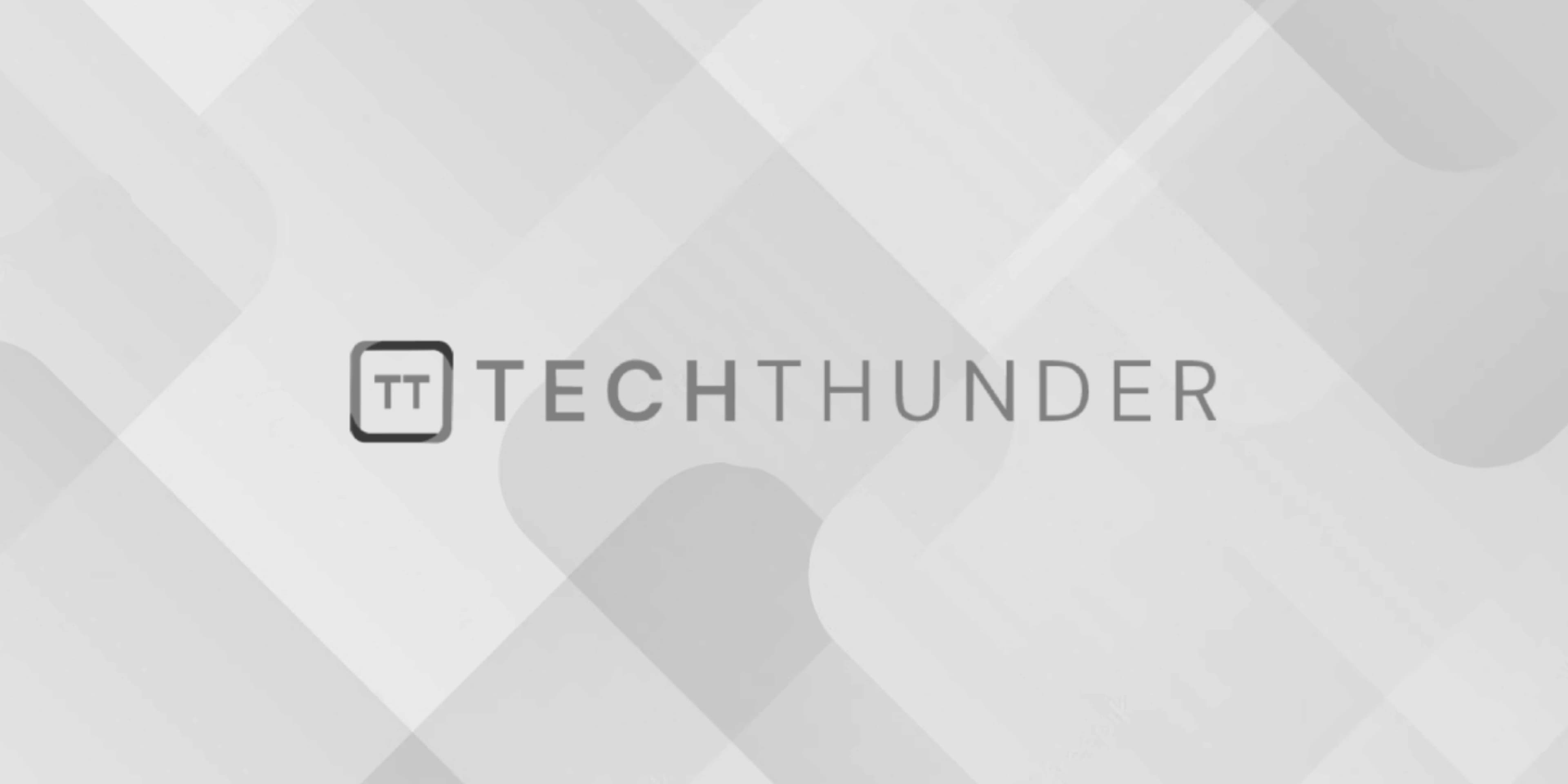
How to add object in array using JavaScript
To add an object to an array in JavaScript, you can use the push()
method or the array’s index. Here are two approaches you can take:
- Using the
push()
method:
// Define an array
var myArray = [];
// Create an object
var myObject = {
key1: value1,
key2: value2,
// ... other key-value pairs
};
// Add the object to the array
myArray.push(myObject);
In this approach, you declare an empty array (myArray
), create an object (myObject
) with the desired key-value pairs, and then use the push()
method to add the object to the array.
- Using the array’s index:
// Define an array
var myArray = [];
// Create an object
var myObject = {
key1: value1,
key2: value2,
// ... other key-value pairs
};
// Add the object to the array
myArray[myArray.length] = myObject;
In this approach, you declare an empty array (myArray
), create an object (myObject
), and then use the array’s length property (myArray.length
) as the index to add the object to the end of the array.
Both approaches achieve the same result of adding the object to the array. Choose the one that suits your coding style and preference.
Note: Make sure to replace value1
, value2
, and other placeholders with actual values or variables based on your requirements.