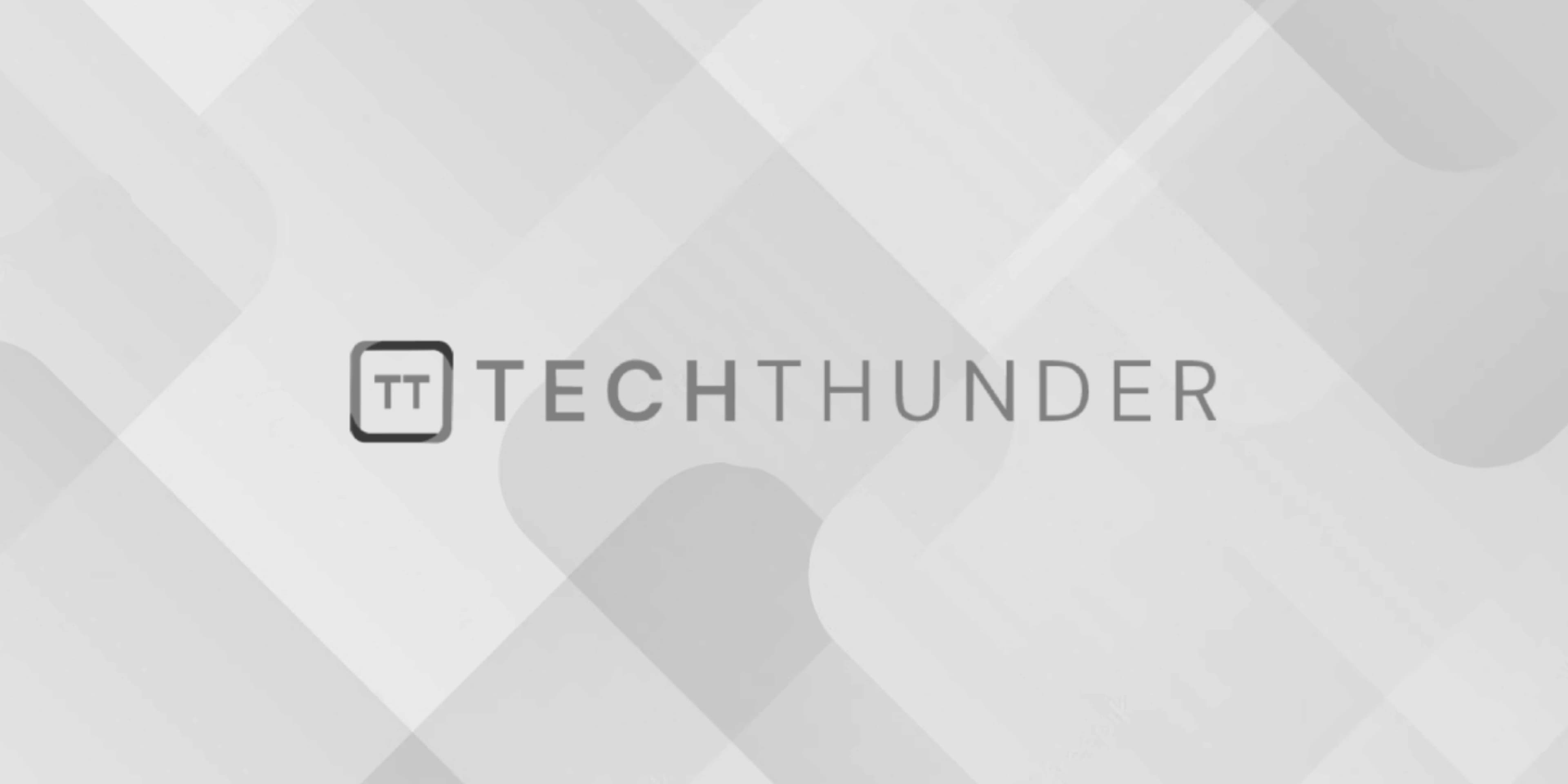
366 views
Difference between preventDefault() and stopPropagation() methods
The preventDefault()
and stopPropagation()
methods are both used in event handling in JavaScript, but they serve different purposes:
preventDefault()
: ThepreventDefault()
method is used to prevent the default behavior of an event. By callingevent.preventDefault()
, you can stop the browser’s default action associated with the event from occurring. For example, if you have a link (<a>
element) and you want to prevent it from navigating to a new page when clicked, you can useevent.preventDefault()
to cancel that default behavior. It is commonly used with events like clicks, form submissions, and key presses.
JavaScript
document.querySelector('a').addEventListener('click', function(event) {
event.preventDefault();
});
stopPropagation()
: ThestopPropagation()
method is used to stop the propagation of an event through the event’s bubbling or capturing phases. In the event propagation model, when an event occurs on an element, it can trigger handlers on that element and also on its ancestor elements (capturing phase) and descendant elements (bubbling phase). By callingevent.stopPropagation()
, you can prevent the event from triggering handlers on the ancestor or descendant elements.
JavaScript
document.querySelector('.inner').addEventListener('click', function(event) {
event.stopPropagation();
console.log('Inner element clicked');
});
document.querySelector('.outer').addEventListener('click', function(event) {
console.log('Outer element clicked');
});
In this example, when you click on the inner element, the event handler on the inner element is triggered. However, with event.stopPropagation()
, the event propagation is stopped, and the event handler on the outer element is not triggered. The message “Inner element clicked” is logged to the console, but “Outer element clicked” is not.
To summarize, preventDefault()
is used to prevent the default behavior of an event, while stopPropagation()
is used to stop the event from further propagating to ancestor or descendant elements. They serve different purposes in controlling event behavior in JavaScript.