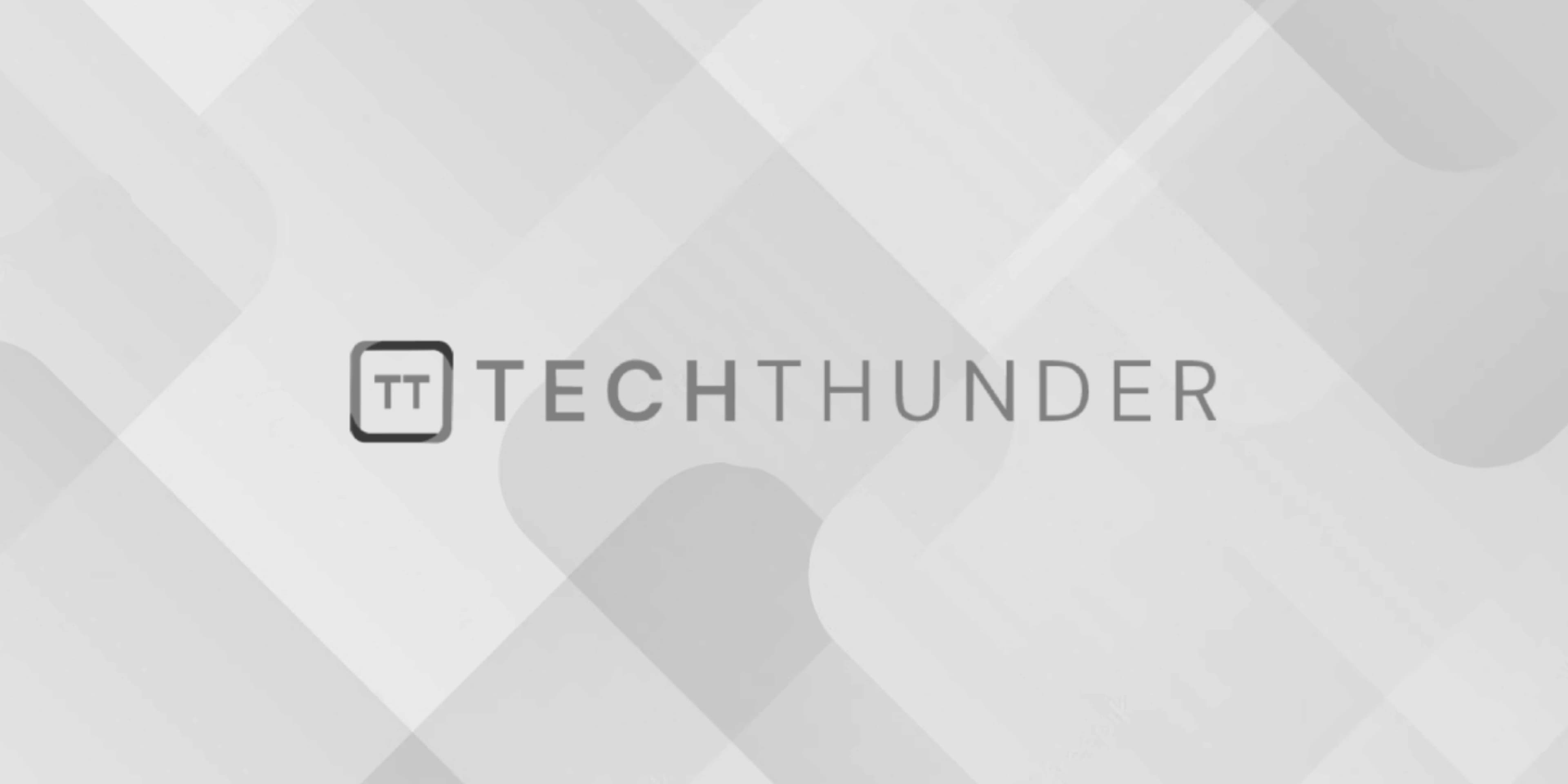
JavaScript constructor Method
In JavaScript, the constructor method is a special method that is automatically called when a new instance of an object is created using the new
keyword. It is used to initialize the object’s properties and perform any setup or initialization tasks.
The constructor method is defined within the object’s constructor function. It is named constructor
and does not have a return type. Here’s an example:
function Person(name, age) {
this.name = name;
this.age = age;
this.sayHello = function() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
};
}
const john = new Person("John", 25);
john.sayHello(); // Output: "Hello, my name is John and I'm 25 years old."
In the above example, the Person
constructor function defines a constructor
method that sets the name
and age
properties of the Person
object. The constructor
method is automatically called when a new Person
instance is created using the new
keyword.
It’s important to note that the constructor method is optional. If you don’t define a constructor method in your object’s constructor function, an empty constructor will be created automatically.
function Person(name, age) {
this.name = name;
this.age = age;
}
const john = new Person("John", 25);
console.log(john.name); // Output: "John"
console.log(john.age); // Output: 25
In this case, the properties name
and age
are still set correctly without an explicit constructor method. However, if you need to perform any additional setup or initialization tasks, you can define a custom constructor method.
It’s worth mentioning that in JavaScript, constructor functions are conventionally named with an initial capital letter to distinguish them from regular functions and indicate that they should be called with the new
keyword.