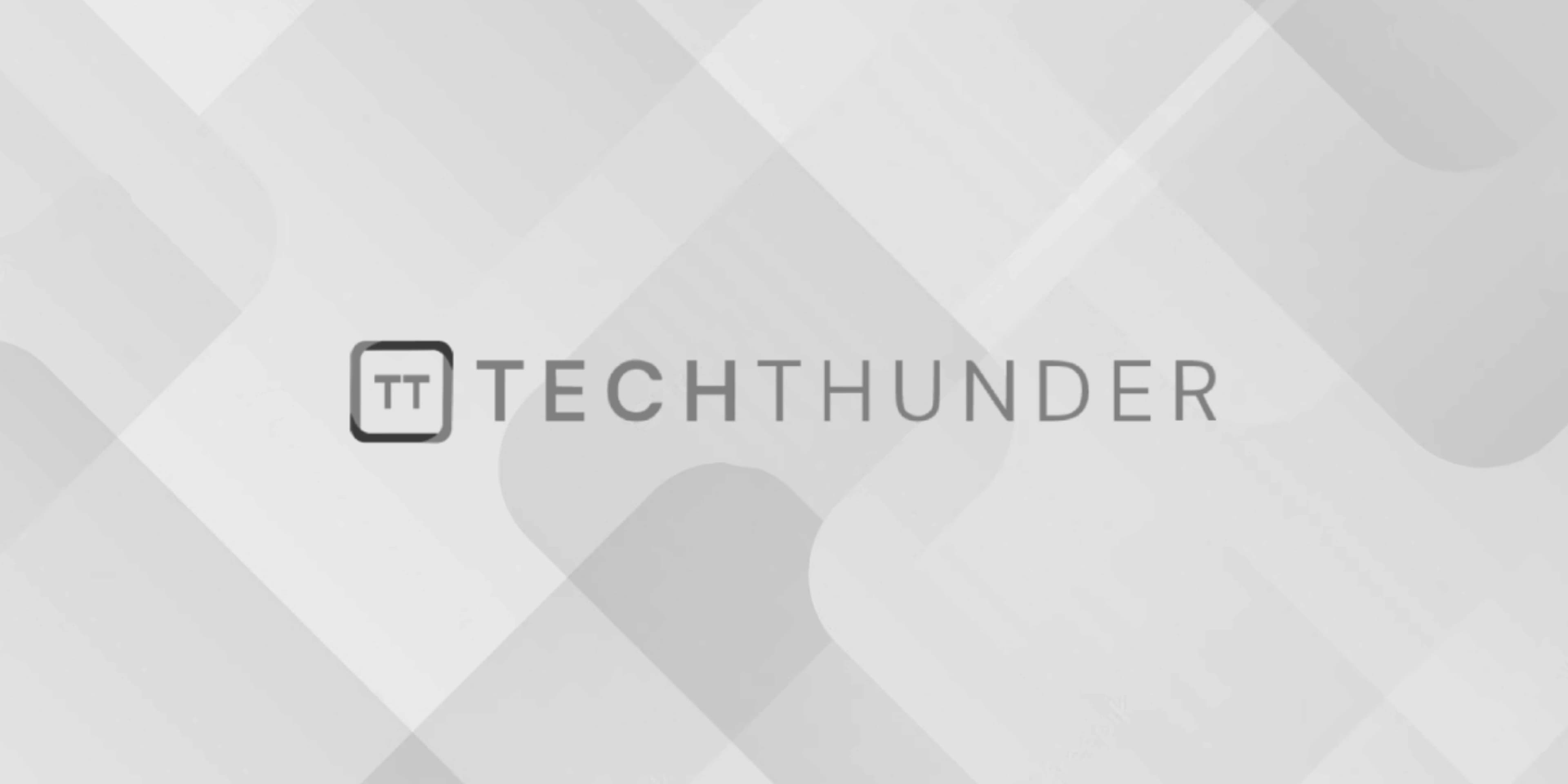
JavaScript appendchild() method
The appendChild()
method in JavaScript is used to add a new child element to an existing parent element. It allows you to dynamically manipulate the structure of an HTML document by adding new elements or moving existing elements within the DOM (Document Object Model).
The appendChild()
method is typically called on a parent element and takes a single argument, which is the child element you want to add. Here’s the basic syntax:
parentElement.appendChild(childElement);
Here, parentElement
is the existing element to which you want to append the child, and childElement
is the element you want to add as a child.
For example, let’s say you have an existing <div>
element with an id
of “myDiv” in your HTML document, and you want to add a new <p>
element as a child to it. You can achieve this using appendChild()
as follows:
const parentElement = document.getElementById('myDiv');
const childElement = document.createElement('p');
childElement.textContent = 'This is a new paragraph.';
parentElement.appendChild(childElement);
In this example, getElementById()
is used to retrieve the parent element with the id
“myDiv”. Then, createElement()
is used to create a new <p>
element, and textContent
is set to provide the text content for the paragraph. Finally, appendChild()
is called on the parent element (parentElement
), passing the childElement
as the argument, which adds the <p>
element as a child to the <div>
element.
After executing the above code, the resulting HTML structure will be:
<div id="myDiv">
<p>This is a new paragraph.</p>
</div>
Note that the appendChild()
method will place the new child element as the last child of the parent element. If the child element already exists in the DOM, it will be moved from its current position to the new location within the parent element.