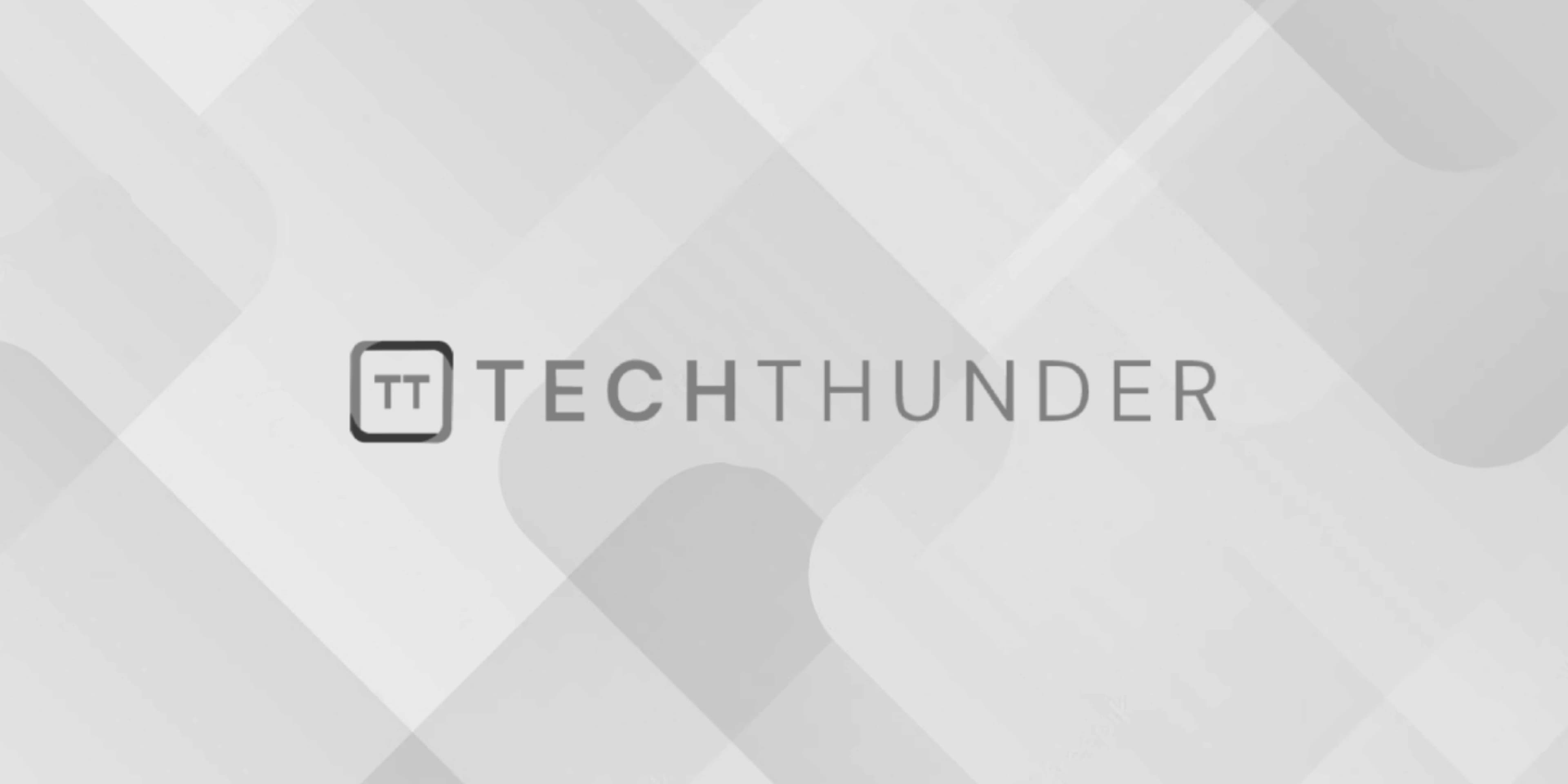
JavaScript Map
The JavaScript Map is a built-in object that allows you to store key-value pairs. It provides an efficient way to associate values with unique keys and perform various operations on the stored data. Here’s an overview of how to use the Map object in JavaScript:
- Creating a Map:
const map = new Map();
- Adding key-value pairs to the Map:
map.set(key1, value1);
map.set(key2, value2);
- Getting the value associated with a key:
const value = map.get(key);
- Checking if a key exists in the Map:
const exists = map.has(key);
- Deleting a key-value pair from the Map:
map.delete(key);
- Clearing all key-value pairs from the Map:
map.clear();
- Getting the number of key-value pairs in the Map:
const size = map.size;
- Iterating over the key-value pairs in the Map:
map.forEach((value, key) => {
// Perform some operation with the key and value
});
// Alternatively, you can use a for...of loop
for (const [key, value] of map) {
// Perform some operation with the key and value
}
Maps can use any value as a key, including objects and primitive values. They provide a more flexible and powerful alternative to using plain JavaScript objects as dictionaries or associative arrays. Additionally, Map maintains the insertion order of the elements, unlike objects where the order is not guaranteed.
It’s important to note that the Map object provides better performance for frequent additions and removals of key-value pairs compared to using plain objects. Maps are commonly used when you need to store and retrieve data using custom keys or when you want to preserve the insertion order of the elements.