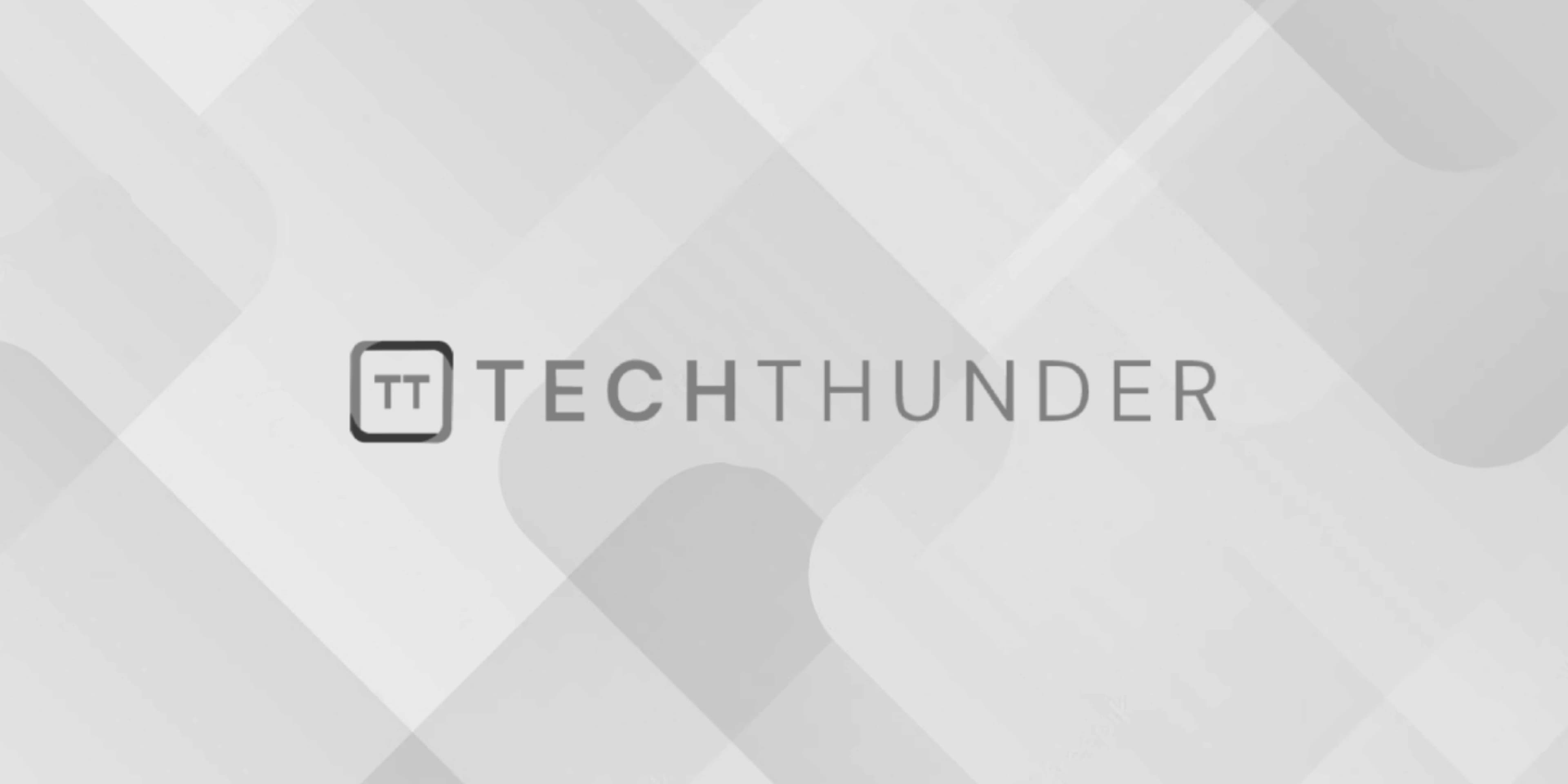
169 views
JavaScript date format
The JavaScript Date
object provides various methods to format dates according to specific patterns. Here are some commonly used methods for formatting dates in JavaScript:
toLocaleDateString()
: This method returns a localized string representation of the date portion of aDate
object. It uses the browser’s default locale settings to format the date. Example:
JavaScript
var date = new Date();
var formattedDate = date.toLocaleDateString();
console.log(formattedDate); // Output: "6/26/2023" (example output)
toLocaleTimeString()
: This method returns a localized string representation of the time portion of aDate
object. It uses the browser’s default locale settings to format the time. Example:
JavaScript
var date = new Date();
var formattedTime = date.toLocaleTimeString();
console.log(formattedTime); // Output: "12:34:56 PM" (example output)
toLocaleString()
: This method returns a localized string representation of both the date and time portions of aDate
object. It uses the browser’s default locale settings to format the date and time. Example:
JavaScript
var date = new Date();
var formattedDateTime = date.toLocaleString();
console.log(formattedDateTime); // Output: "6/26/2023, 12:34:56 PM" (example output)
- Formatting with libraries: To have more control over date formatting, you can use external libraries like Moment.js or date-fns. These libraries provide extensive formatting options and support for custom date formats. Example with Moment.js:
JavaScript
var date = moment();
var formattedDate = date.format('YYYY-MM-DD');
console.log(formattedDate); // Output: "2023-06-26" (example output)
Note that Moment.js needs to be included in your project for the above example to work.
- Manually formatting dates: You can also manually format dates by using the
get
methods of theDate
object, such asgetDate()
,getMonth()
,getFullYear()
, etc., and constructing the desired date string using string concatenation or template literals.
JavaScript
var date = new Date();
var formattedDate = `${date.getMonth() + 1}/${date.getDate()}/${date.getFullYear()}`;
console.log(formattedDate); // Output: "6/26/2023" (example output)
These are just a few examples of how you can format dates in JavaScript. The choice of formatting method depends on your specific requirements and the level of control you need over the date format.