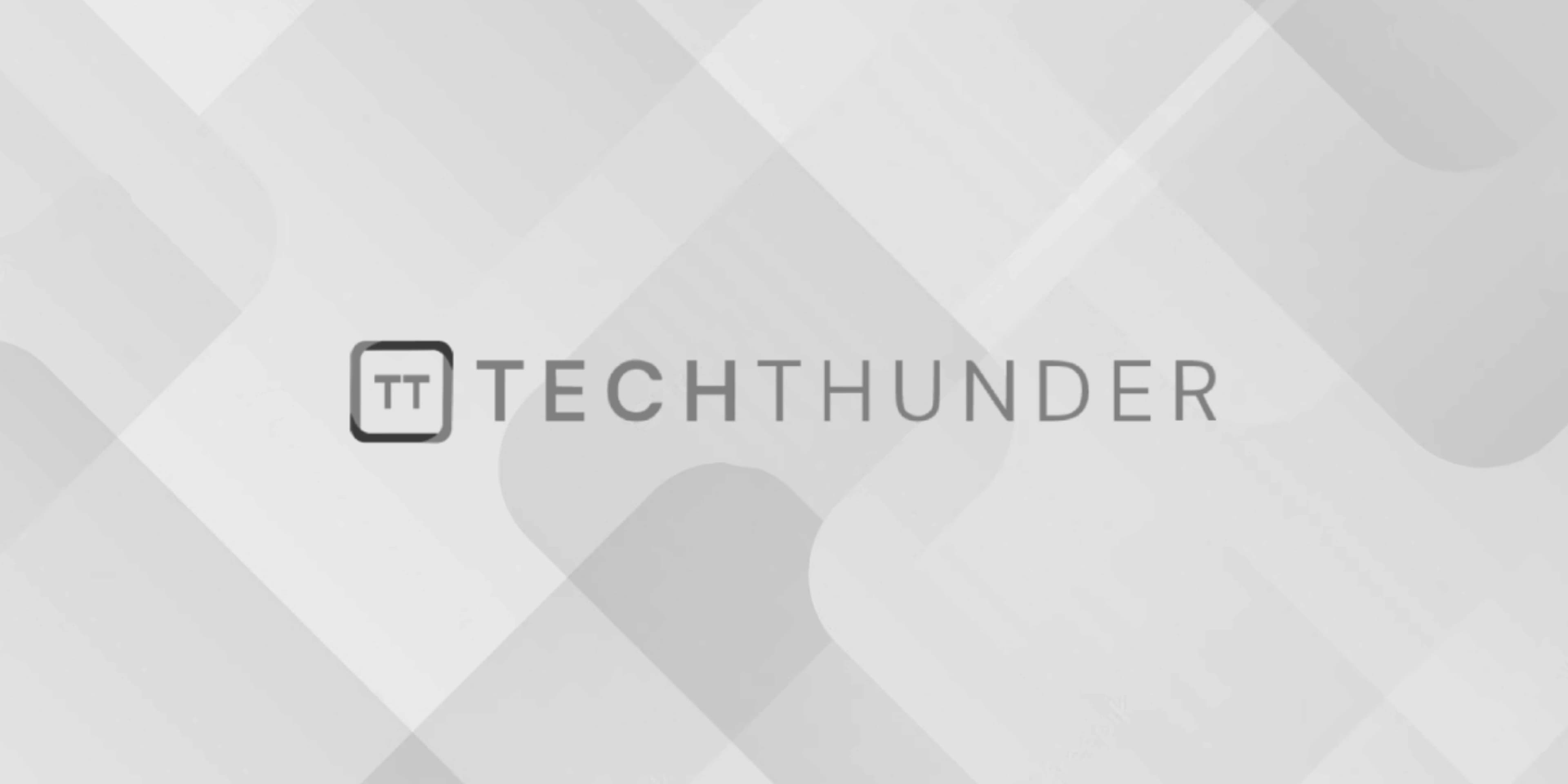
Javascript promise chaining
JavaScript promises allow for chaining multiple asynchronous operations in a sequential manner using the .then()
method. The .then()
method returns a new promise that can be further chained with additional .then()
methods. This pattern is known as promise chaining. Here’s an example to illustrate how promise chaining works:
function asyncOperation1() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Operation 1 completed");
}, 1000);
});
}
function asyncOperation2(data) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(`${data}, Operation 2 completed`);
}, 1000);
});
}
asyncOperation1()
.then((result1) => {
console.log(result1); // Output: "Operation 1 completed"
return asyncOperation2(result1);
})
.then((result2) => {
console.log(result2); // Output: "Operation 1 completed, Operation 2 completed"
})
.catch((error) => {
console.log(error);
});
In this example, we have two asynchronous functions, asyncOperation1()
and asyncOperation2()
, which both return promises. We call asyncOperation1()
first and then chain the .then()
method to it. Inside the .then()
callback, we receive the result of asyncOperation1()
and pass it as an argument to asyncOperation2()
. This creates a sequential flow where the result of the first operation is passed to the second operation.
By chaining multiple .then()
methods, each subsequent operation waits for the previous one to complete before executing. The final .then()
callback receives the result of the last operation in the chain. If any promise in the chain is rejected, the control jumps to the nearest .catch()
method to handle the error.
Promise chaining allows you to perform a series of asynchronous operations in a readable and sequential manner, avoiding callback hell and improving code organization.