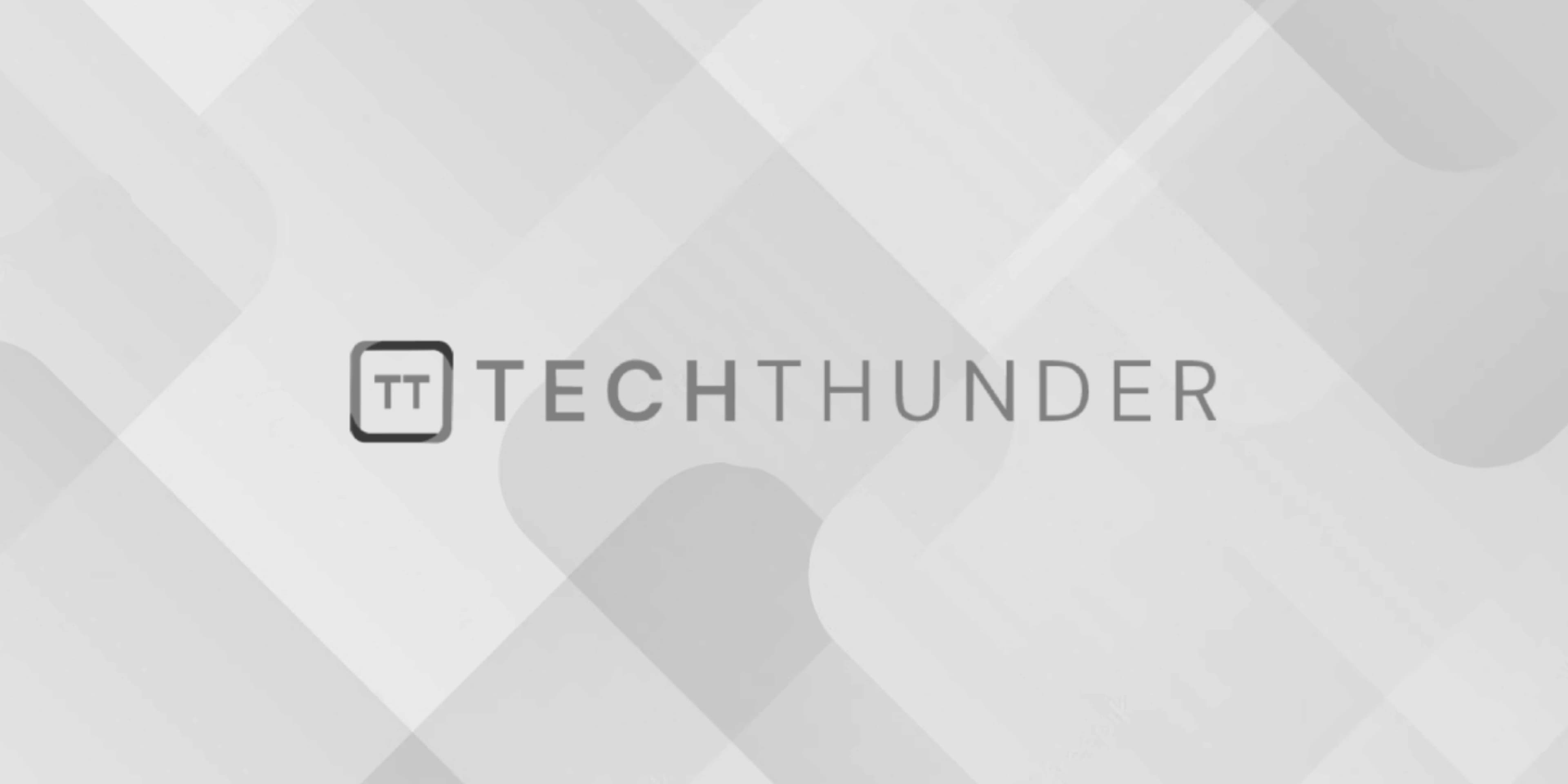
JavaScript prompt()
The prompt()
function in JavaScript is used to display a dialog box with a message and an input field where the user can enter a response. It returns the value entered by the user as a string or null
if the user cancels the prompt.
Here’s the basic syntax of the prompt()
function:
var result = prompt(message, defaultText);
message
(optional): A string that represents the message or question to display in the dialog box.defaultText
(optional): A string that represents the default value displayed in the input field. It is optional and can be omitted.
Here’s an example of using prompt()
to ask the user for their name and display a greeting:
var name = prompt("Please enter your name:");
if (name !== null) {
alert("Hello, " + name + "!");
} else {
alert("You cancelled the prompt.");
}
In this example, the prompt()
function displays a dialog box with the message “Please enter your name:”. If the user enters a name and clicks “OK”, the value entered by the user is stored in the name
variable and a greeting message is displayed. If the user clicks “Cancel”, the value of name
will be null
, and a different message is displayed.
Note that prompt()
is a synchronous function, which means that the code execution will pause until the user responds to the prompt. It’s commonly used for simple input and quick user interactions.