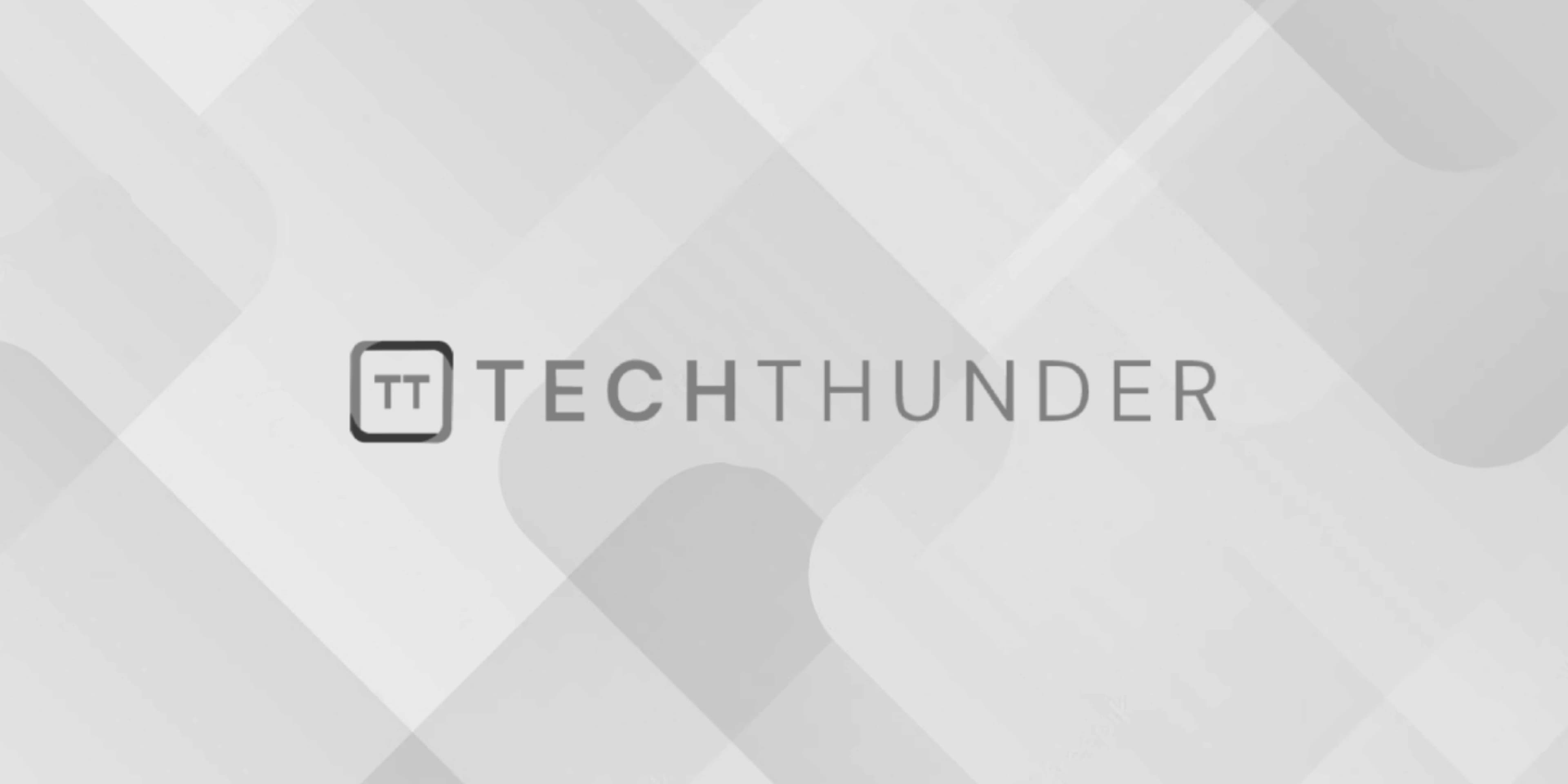
JavaScript create and download CSV file
To create and download a CSV file in JavaScript, you can dynamically generate the CSV content and create a data URL that represents the file. Then, you can create a link with the data URL and trigger a click event to initiate the file download. Here’s an example:
function createAndDownloadCSV(csvContent, fileName) {
var encodedUri = encodeURI(csvContent);
var link = document.createElement("a");
link.setAttribute("href", "data:text/csv;charset=utf-8," + encodedUri);
link.setAttribute("download", fileName);
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
}
// Example usage:
var csvContent = "Name,Email\nJohn Doe,[email protected]\nJane Smith,[email protected]";
var fileName = "data.csv";
createAndDownloadCSV(csvContent, fileName);
In this example, the createAndDownloadCSV
function takes two parameters: csvContent
and fileName
.
Inside the function, encodeURI
is used to encode the CSV content to ensure proper handling of special characters.
A new <a>
element is created using document.createElement("a")
. The href
attribute is set to a data URL representing the CSV content. The download
attribute is set to the desired file name.
The link element is appended to the document body using document.body.appendChild(link)
.
A click event is triggered on the link element using link.click()
, which initiates the file download.
Finally, the link element is removed from the document body using document.body.removeChild(link)
.
To create and download a CSV file, you can call the createAndDownloadCSV
function with the CSV content and file name as arguments. In the example usage, we provide a sample CSV content with two columns (Name and Email) and two rows of data. The file is named “data.csv”.
Executing this code will create and download a CSV file containing the provided CSV content with the specified file name.