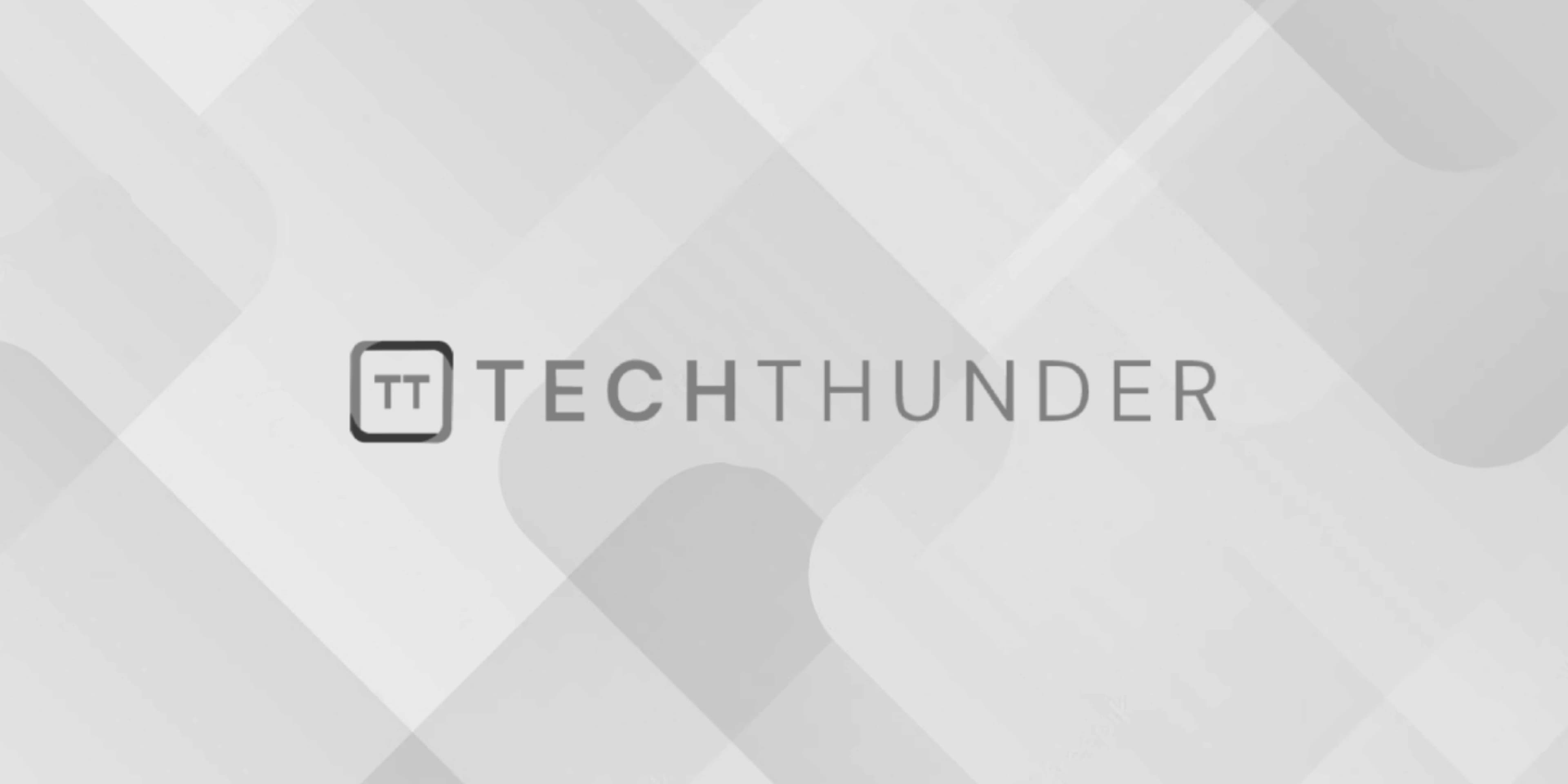
How to remove a Key/Property from an object in JavaScript
To remove a key/property from an object in JavaScript, you can use the delete
keyword or create a new object without the specific key/property. Here are two common approaches:
- Using the
delete
keyword:
const obj = {
key1: 'value1',
key2: 'value2',
key3: 'value3'
};
delete obj.key2;
console.log(obj); // Output: { key1: 'value1', key3: 'value3' }
In this example, the delete
keyword is used to remove the key2
property from the obj
object. After executing the delete
statement, the obj
object will no longer contain the key2
property.
- Creating a new object without the specific key/property:
const obj = {
key1: 'value1',
key2: 'value2',
key3: 'value3'
};
const { key2, ...newObj } = obj;
console.log(newObj); // Output: { key1: 'value1', key3: 'value3' }
In this approach, an ES6 destructuring assignment is used to create a new object (newObj
) by omitting the key2
property from the original obj
object. The rest/spread operator (...
) is used to gather the remaining properties into newObj
, excluding the specified key/property.
Both approaches achieve the same result of removing a key/property from an object. The choice between them depends on your specific use case and coding style. If you need to mutate the original object, the delete
keyword can be used. If you prefer immutability and want to create a new object without the specific key/property, the destructuring assignment approach can be used.