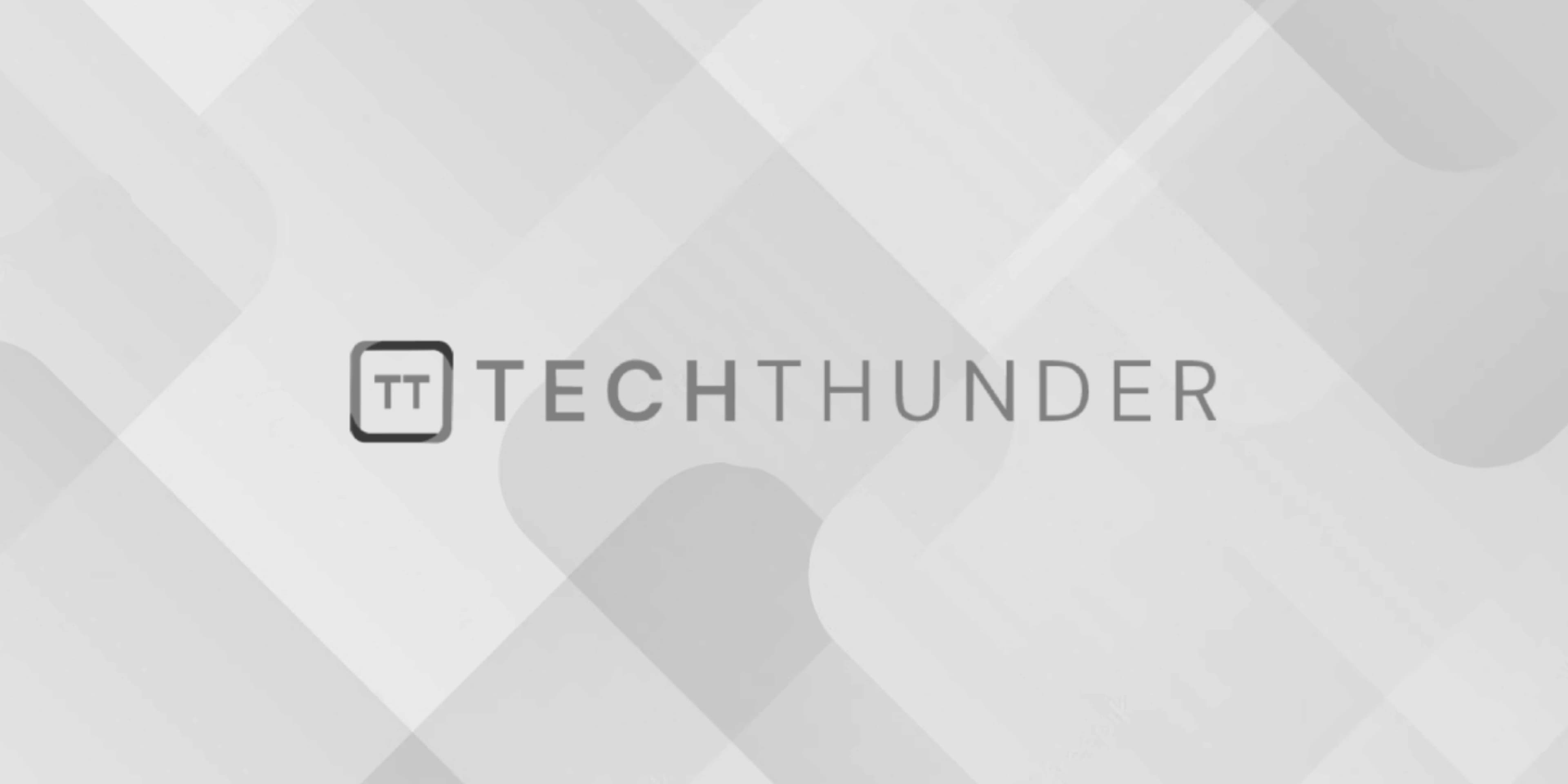
JavaScript Const
The JavaScript const
is a keyword used to declare a constant variable, meaning its value cannot be reassigned after it has been initialized. It provides a way to declare variables that are intended to remain unchanged throughout the program execution.
Here’s an example of using const
to declare a constant variable:
const PI = 3.14159;
In this example, PI
is declared as a constant variable with the value of 3.14159
. Once assigned, the value of PI
cannot be changed.
It’s important to note that const
variables must be assigned a value during declaration. Unlike let
or var
, which can be declared without initialization, const
requires an initial value.
Here are a few key points about const
:
- Reassignment is not allowed: Once a
const
variable is assigned a value, it cannot be reassigned to a new value. Any attempt to reassign aconst
variable will result in a runtime error. - Block scope: Like
let
,const
is block-scoped, which means it is only accessible within the block in which it is defined or declared. - Immutable value: Although a
const
variable cannot be reassigned, it does not make the value itself immutable. For example, if aconst
variable holds an object or an array, the properties or elements of the object or array can still be modified.
const person = {
name: "John",
age: 30
};
person.age = 31; // Valid - Modifying a property of the object
person = {}; // Invalid - Reassignment of the entire object
- Constant-like behavior: While
const
provides a way to declare constants, it does not guarantee immutability or true “constants” in the traditional sense. It primarily prevents reassignment of the variable itself.
It is common practice to use const
when you know that the value of a variable will remain constant throughout its scope. This helps improve code readability and maintainability by conveying the intent of not allowing reassignment.
However, if you need a variable that may have a changing value, you should use let
instead of const
.