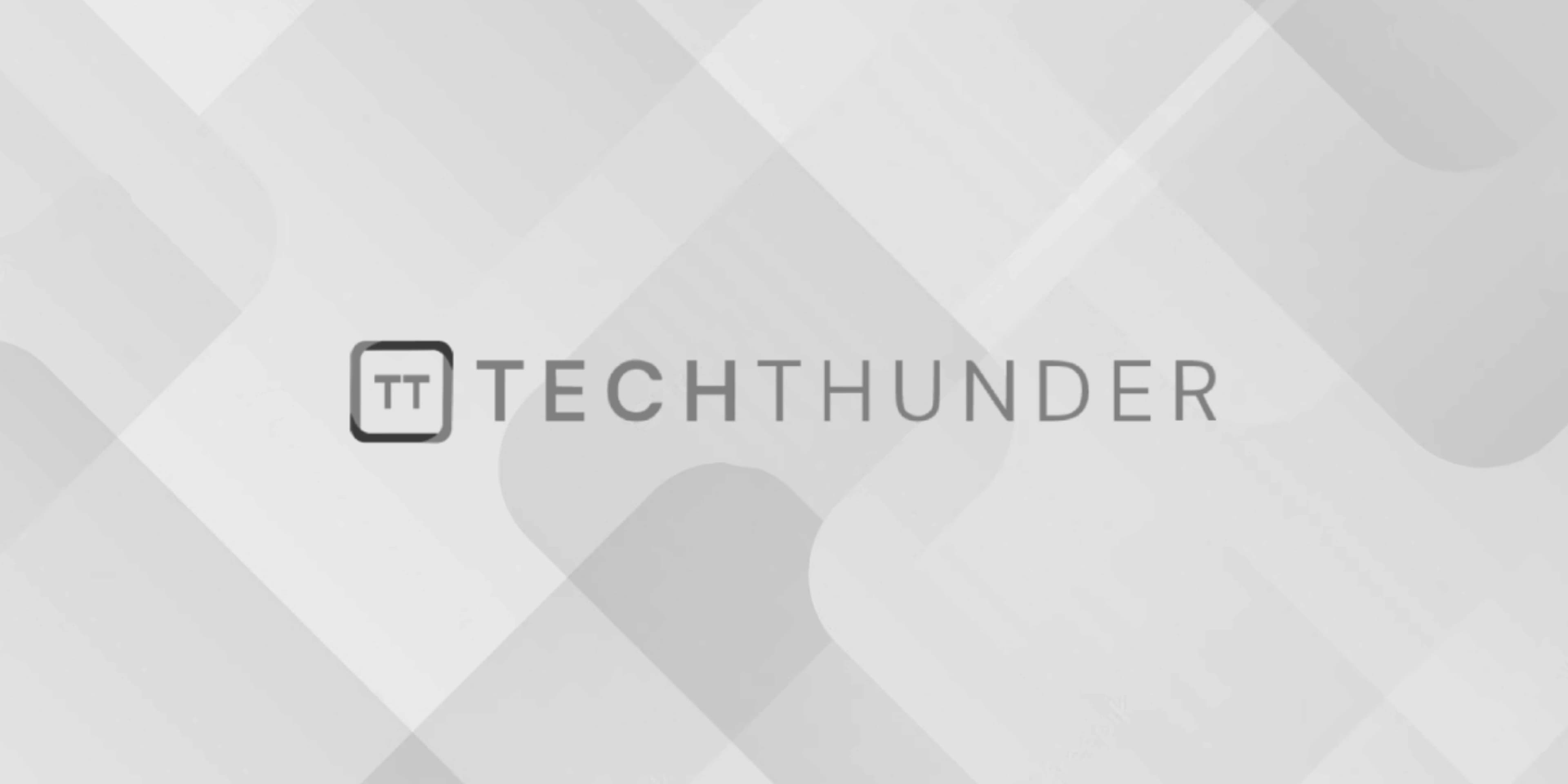
JavaScript Promise
JavaScript Promise is an object used for asynchronous programming. It represents the eventual completion or failure of an asynchronous operation and allows you to handle the result asynchronously without blocking the execution of other code.
A Promise can be in one of three states:
- Pending: The initial state before the promise is settled, neither fulfilled nor rejected.
- Fulfilled: The state when the promise is successfully resolved with a value.
- Rejected: The state when the promise encounters an error or is rejected with a reason.
A Promise is created using the Promise constructor, which takes a callback function with two parameters: resolve and reject. Inside the callback function, you perform an asynchronous operation and call the appropriate function based on the outcome:
const promise = new Promise((resolve, reject) => {
// Asynchronous operation
// If the operation is successful, call resolve(value)
// If the operation fails, call reject(reason)
});
You can chain multiple asynchronous operations using the then() method, which takes two optional callback functions: onFulfilled and onRejected. The onFulfilled callback is executed when the promise is successfully resolved, and the onRejected callback is executed when the promise is rejected.
promise.then(
(value) => {
// Handle the fulfilled value
},
(reason) => {
// Handle the rejection reason
}
);
Promises also provide a catch() method to handle any errors that occur during the promise chain. It’s used as a shorthand for handling only the rejection cases:
promise.catch((reason) => {
// Handle the rejection reason
});
Promises provide a powerful mechanism for handling asynchronous operations in a more structured and readable way. They help avoid callback hell and provide better error handling and control flow. With the introduction of Promises, asynchronous programming in JavaScript has become more efficient and manageable.