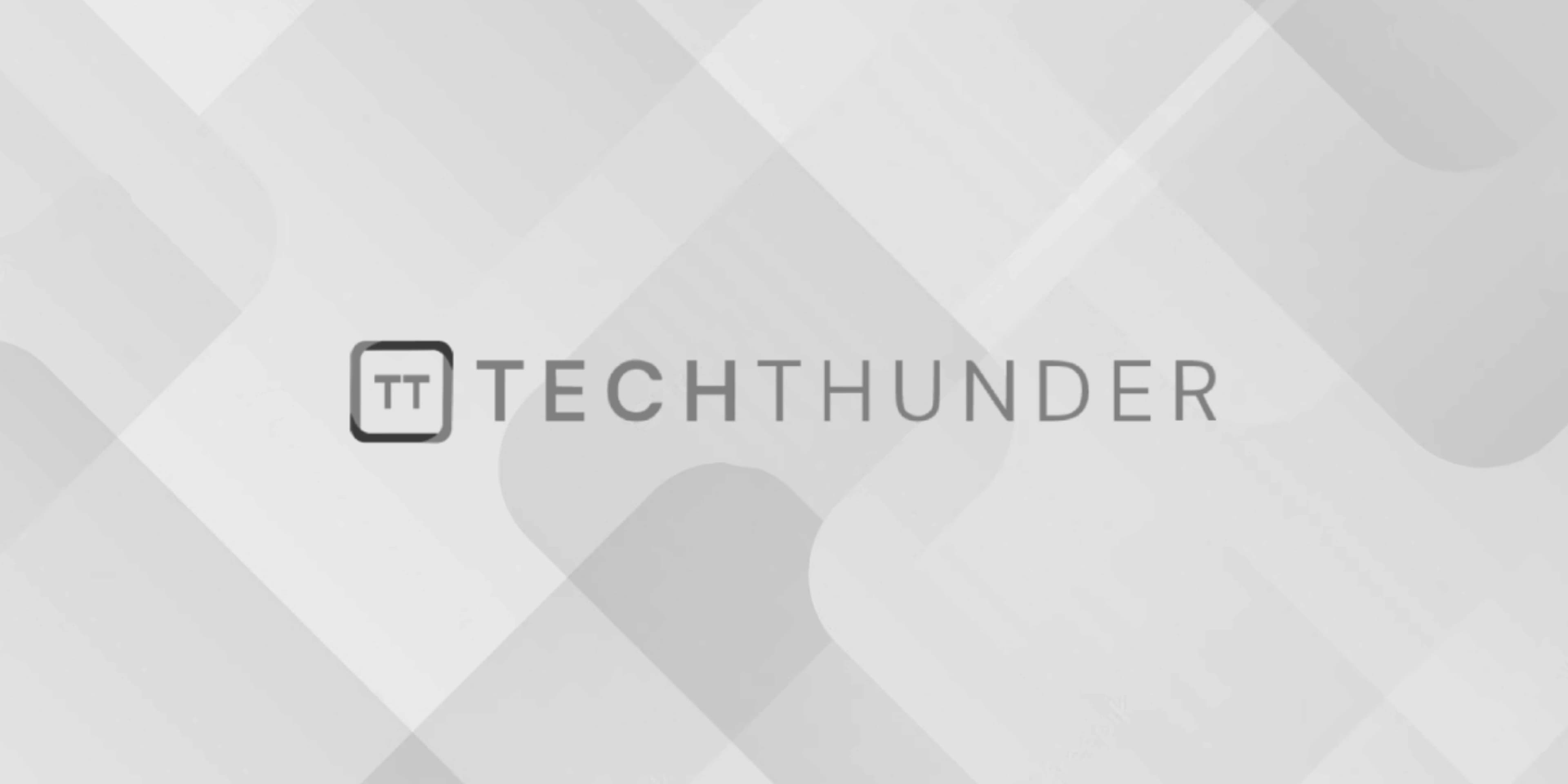
JavaScript Factory Function
The JavaScript factory function is a function that creates and returns objects. It is a pattern for object creation that allows you to encapsulate the object creation process within a function and provide a way to customize the objects being created.
Here’s an example of a factory function that creates person objects:
function createPerson(name, age) {
return {
name: name,
age: age,
greet: function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
}
};
}
// Create a person using the factory function
var person1 = createPerson('John', 25);
person1.greet(); // Output: Hello, my name is John and I am 25 years old.
// Create another person using the factory function
var person2 = createPerson('Jane', 30);
person2.greet(); // Output: Hello, my name is Jane and I am 30 years old.
In this example, the createPerson
function is a factory function that takes name
and age
as parameters. It returns an object with properties name
and age
, as well as a greet
method.
You can create multiple person objects by calling the createPerson
function with different arguments, and each object will have its own unique name and age values.
The advantage of using a factory function is that it provides a way to create objects with consistent structure and behavior, while allowing customization through function arguments. It encapsulates the object creation process and promotes code reusability.
Factory functions can be a flexible alternative to using constructor functions or classes for object creation in JavaScript. They are especially useful when you need to create multiple objects with similar characteristics but don’t require the use of prototypes or inheritance.