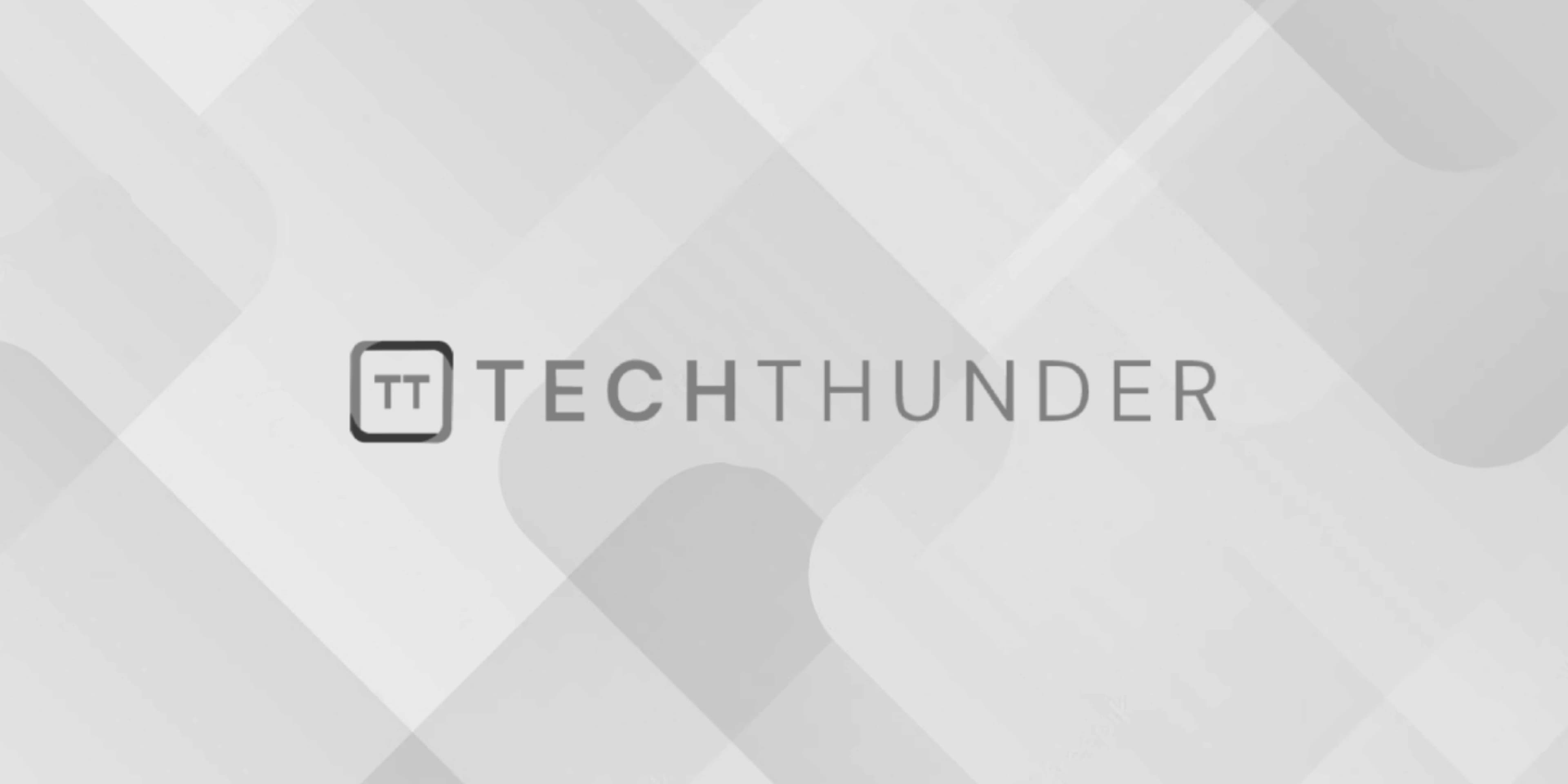
109 views
Get and Set Scroll Position of an Element
To get and set the scroll position of an element in JavaScript, you can use the scrollTop
property. Here’s an example:
JavaScript
// Get the scroll position of an element
function getScrollPosition(element) {
return element.scrollTop;
}
// Set the scroll position of an element
function setScrollPosition(element, position) {
element.scrollTop = position;
}
// Example usage
var myElement = document.getElementById('myElement');
// Get the current scroll position
var currentPosition = getScrollPosition(myElement);
console.log(currentPosition);
// Set the scroll position to a specific value
setScrollPosition(myElement, 200);
In this code, the getScrollPosition
function takes an element as input and returns its current scroll position using the scrollTop
property.
The setScrollPosition
function takes an element and a position as input and sets the scroll position of the element to the specified value by assigning the position
value to the scrollTop
property.
You can replace 'myElement'
with the appropriate ID or reference to the element you want to manipulate. The example demonstrates how to get the current scroll position and set it to a specific value (200 in this case).