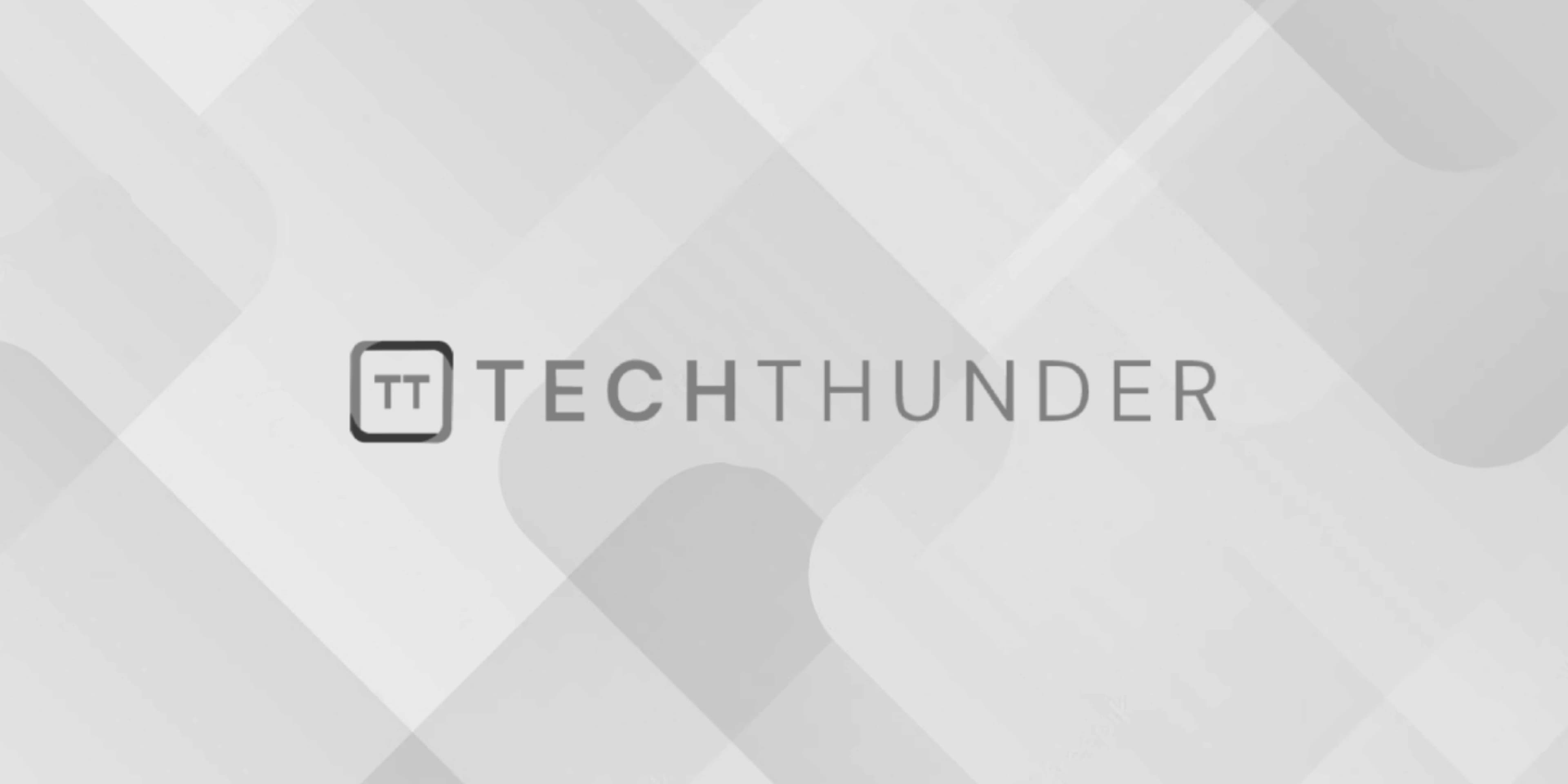
JavaScript padStart() Method
The padStart()
method in JavaScript is used to pad a string with another string or characters at the beginning (left side) until it reaches a specified length. It is often used to ensure that a string has a certain minimum length by adding padding characters.
The syntax for padStart()
is as follows:
str.padStart(targetLength [, padString])
str
: The string to pad.targetLength
: The desired length of the resulting padded string.padString
(optional): The string or characters to use for padding. If not specified, it defaults to a single space character.
Here’s an example usage of padStart()
:
const str = '5';
const paddedStr = str.padStart(4, '0');
console.log(paddedStr); // Output: '0005'
In this example, the str
variable contains the string '5'
. We call the padStart()
method on str
and provide a targetLength
of 4
and a padString
of '0'
. The resulting padded string is '0005'
, which has a length of 4
characters.
If the length of the original string is already equal to or greater than the targetLength
, padStart()
will not perform any padding and simply return the original string:
const str = 'Hello';
const paddedStr = str.padStart(6, '0');
console.log(paddedStr); // Output: 'Hello'
In this case, the length of the original string 'Hello'
is already 5
, which is greater than the targetLength
of 6
. Therefore, padStart()
does not add any padding and returns the original string 'Hello'
.
The padStart()
method is useful for formatting and aligning strings, especially when dealing with fixed-width columns or formatting numbers. It allows you to easily add leading zeros or other characters to ensure consistent string lengths.