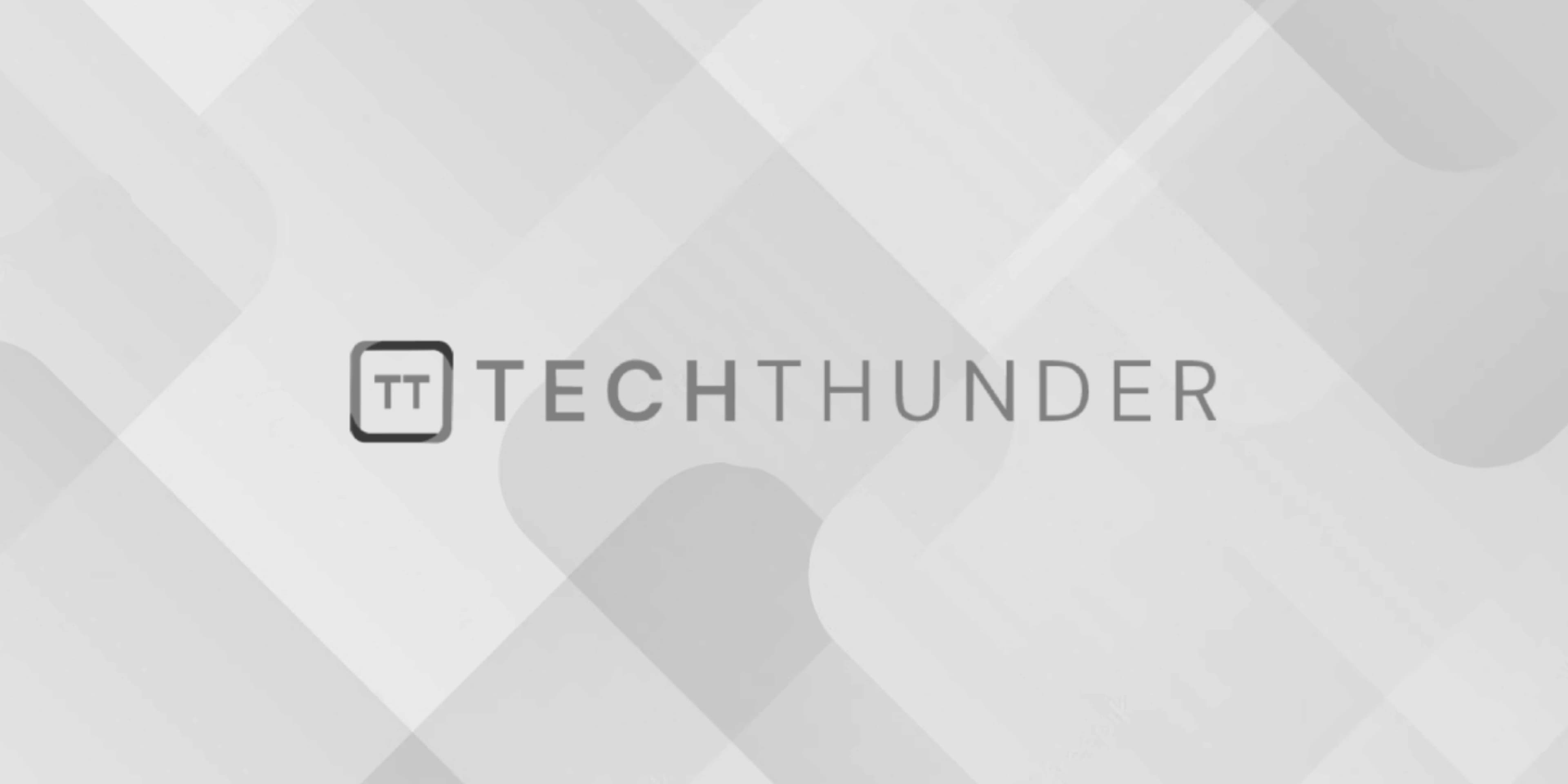
206 views
Palindrome in JavaScript
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. In JavaScript, you can check if a string is a palindrome using various approaches. Here’s one way to check for a palindrome in JavaScript:
JavaScript
function isPalindrome(str) {
// Remove non-alphanumeric characters and convert to lowercase
var cleanedStr = str.toLowerCase().replace(/[^a-zA-Z0-9]+/g, '');
// Reverse the string
var reversedStr = cleanedStr.split('').reverse().join('');
// Compare the original and reversed strings
return cleanedStr === reversedStr;
}
// Test the function
console.log(isPalindrome("level")); // true
console.log(isPalindrome("Hello")); // false
console.log(isPalindrome("A man, a plan, a canal: Panama")); // true
console.log(isPalindrome("12321")); // true
console.log(isPalindrome("12345")); // false
In this code, the isPalindrome
function takes a string (str
) as input and performs the following steps:
- It cleans the string by converting it to lowercase and removing any non-alphanumeric characters using a regular expression.
- It reverses the cleaned string by splitting it into an array of characters, reversing the array, and joining the characters back together.
- It compares the cleaned string with the reversed string and returns
true
if they are equal, indicating that the original string is a palindrome. Otherwise, it returnsfalse
.
You can test this function with different strings to see if they are palindromes.