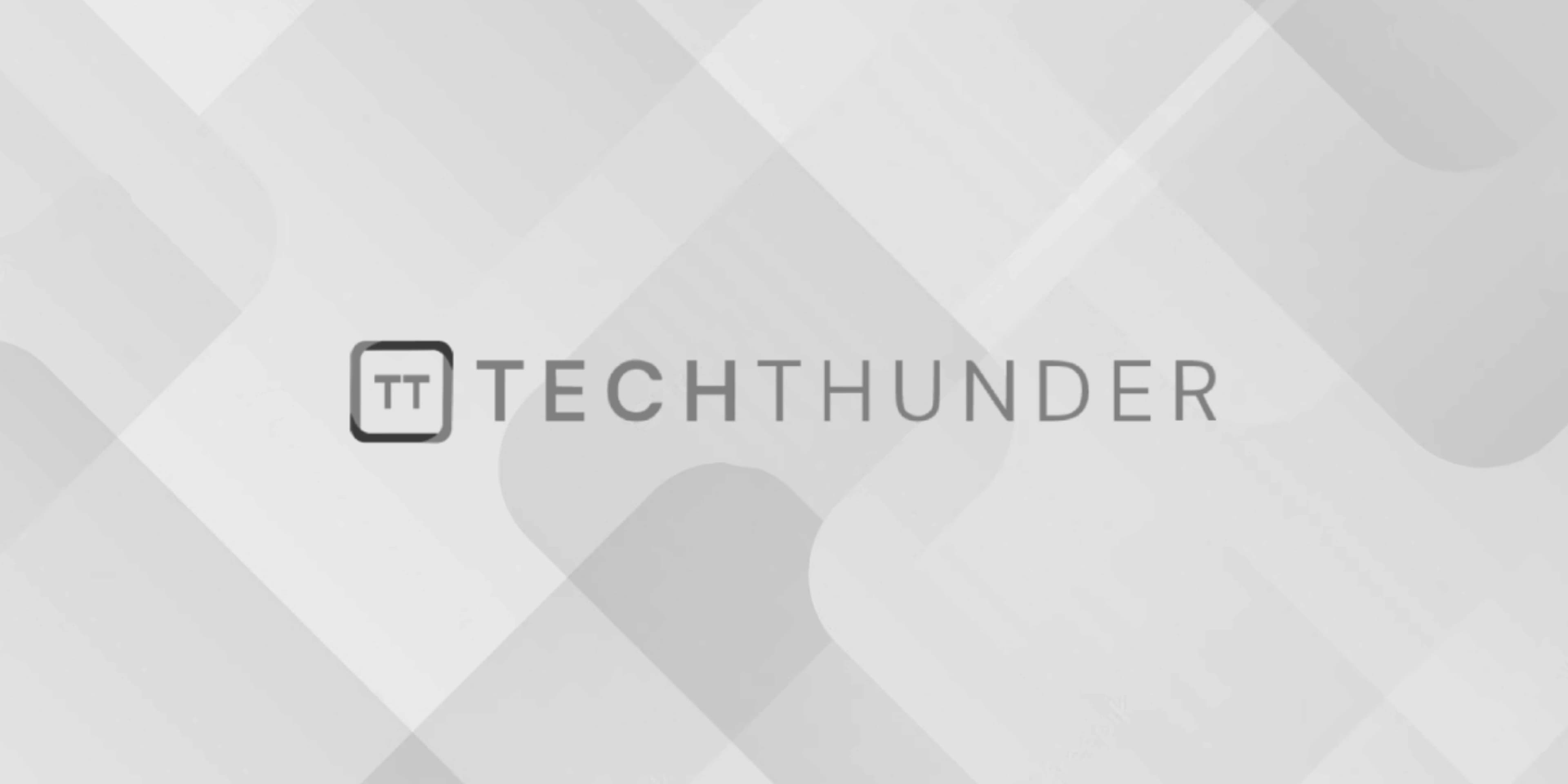
183 views
Calculate days between two dates in JavaScript
To calculate the number of days between two dates in JavaScript, you can use the following steps:
- Create two Date objects representing the start and end dates.
- Calculate the difference between the two dates in milliseconds.
- Convert the milliseconds difference to days.
Here’s an example of how you can implement it:
JavaScript
function calculateDaysBetweenDates(startDate, endDate) {
// Convert the start and end dates to Date objects
var start = new Date(startDate);
var end = new Date(endDate);
// Calculate the difference in milliseconds
var differenceMs = end - start;
// Convert milliseconds to days
var days = Math.floor(differenceMs / (1000 * 60 * 60 * 24));
return days;
}
// Example usage
var startDate = '2023-01-01';
var endDate = '2023-01-10';
var daysBetween = calculateDaysBetweenDates(startDate, endDate);
console.log(daysBetween); // Output: 9
In this example, the calculateDaysBetweenDates
function takes two date strings as input (in the format ‘YYYY-MM-DD’). It converts the strings to Date objects, calculates the difference in milliseconds using the subtraction operator, and then divides the difference by the number of milliseconds in a day (1000 * 60 * 60 * 24) to get the number of days between the dates.
Note that the result is rounded down using the Math.floor()
function to ensure we get an integer number of days.