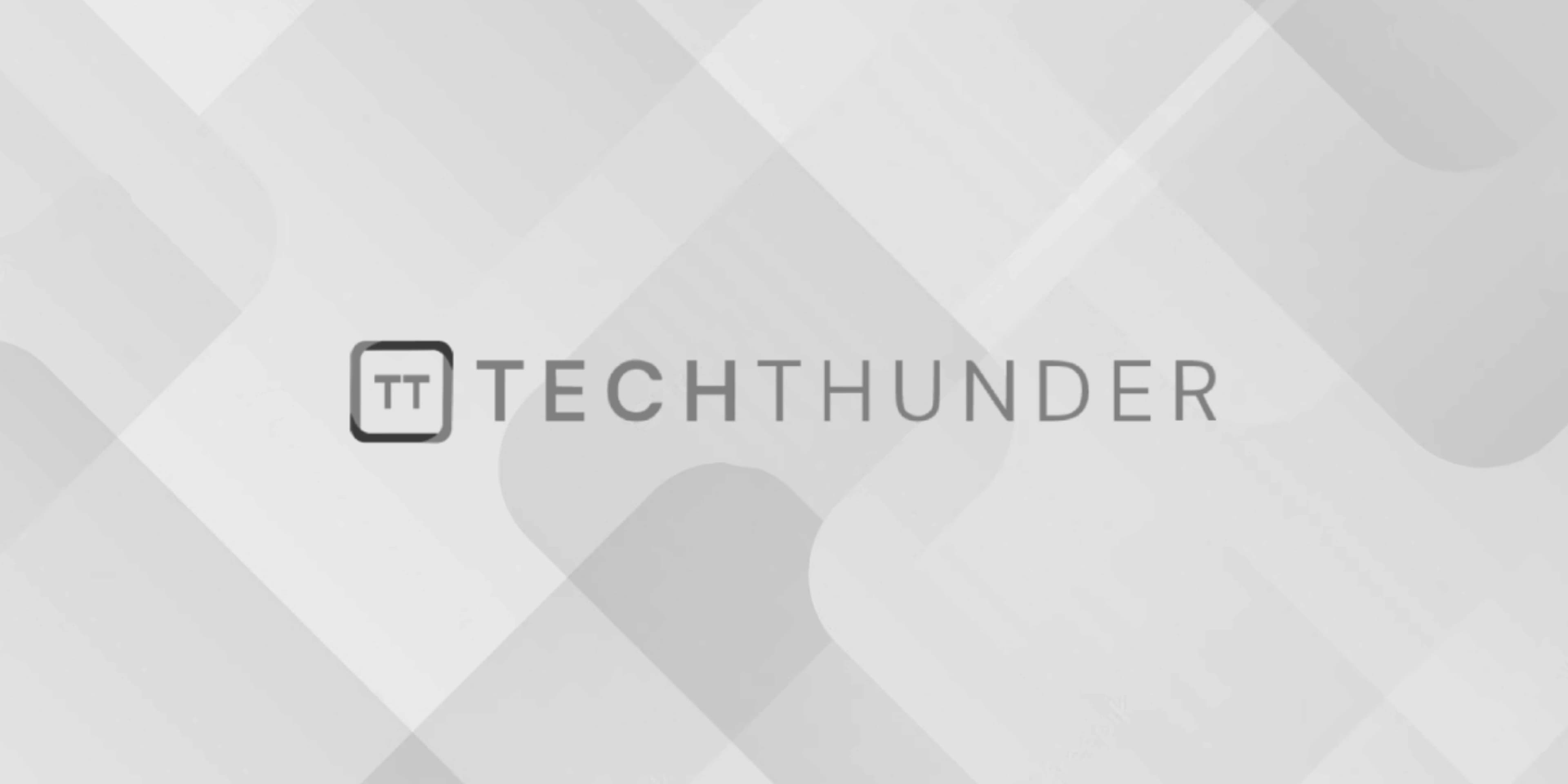
Getting Child Elements of a Node in JavaScript
we can retrieve the child elements of a node using various properties and methods available on the parent node. Here are a few commonly used approaches:
childNodes
property:
ThechildNodes
property returns a collection of all child nodes, including elements, text nodes, and other node types. To get only the child elements, you can filter thechildNodes
using thenodeType
property.
const parent = document.getElementById('parentElement');
const childElements = Array.from(parent.childNodes).filter(node => node.nodeType === Node.ELEMENT_NODE);
In this example, we first retrieve the parent element using its ID, and then we convert the childNodes
collection to an array using Array.from()
. We filter the array to include only elements by checking the nodeType
property against Node.ELEMENT_NODE
constant.
children
property:
Thechildren
property returns a collection of only the child elements of a node, excluding other types of nodes like text nodes or comments.
const parent = document.getElementById('parentElement');
const childElements = Array.from(parent.children);
Here, we directly access the children
property of the parent node and convert the collection to an array using Array.from()
.
querySelectorAll()
method:
ThequerySelectorAll()
method allows you to select elements within a parent node using CSS selectors. You can pass a CSS selector as an argument to retrieve the matching child elements.
const parent = document.getElementById('parentElement');
const childElements = Array.from(parent.querySelectorAll('.childElement'));
In this example, we select the child elements with a class of childElement
within the parent node using the CSS class selector .childElement
. The querySelectorAll()
method returns a collection, which we convert to an array using Array.from()
.
Note: In all the above examples, we convert the collections to arrays using Array.from()
to make it easier to work with and utilize array methods.
Choose the approach that best fits your requirements based on the specific scenario and the structure of your HTML.