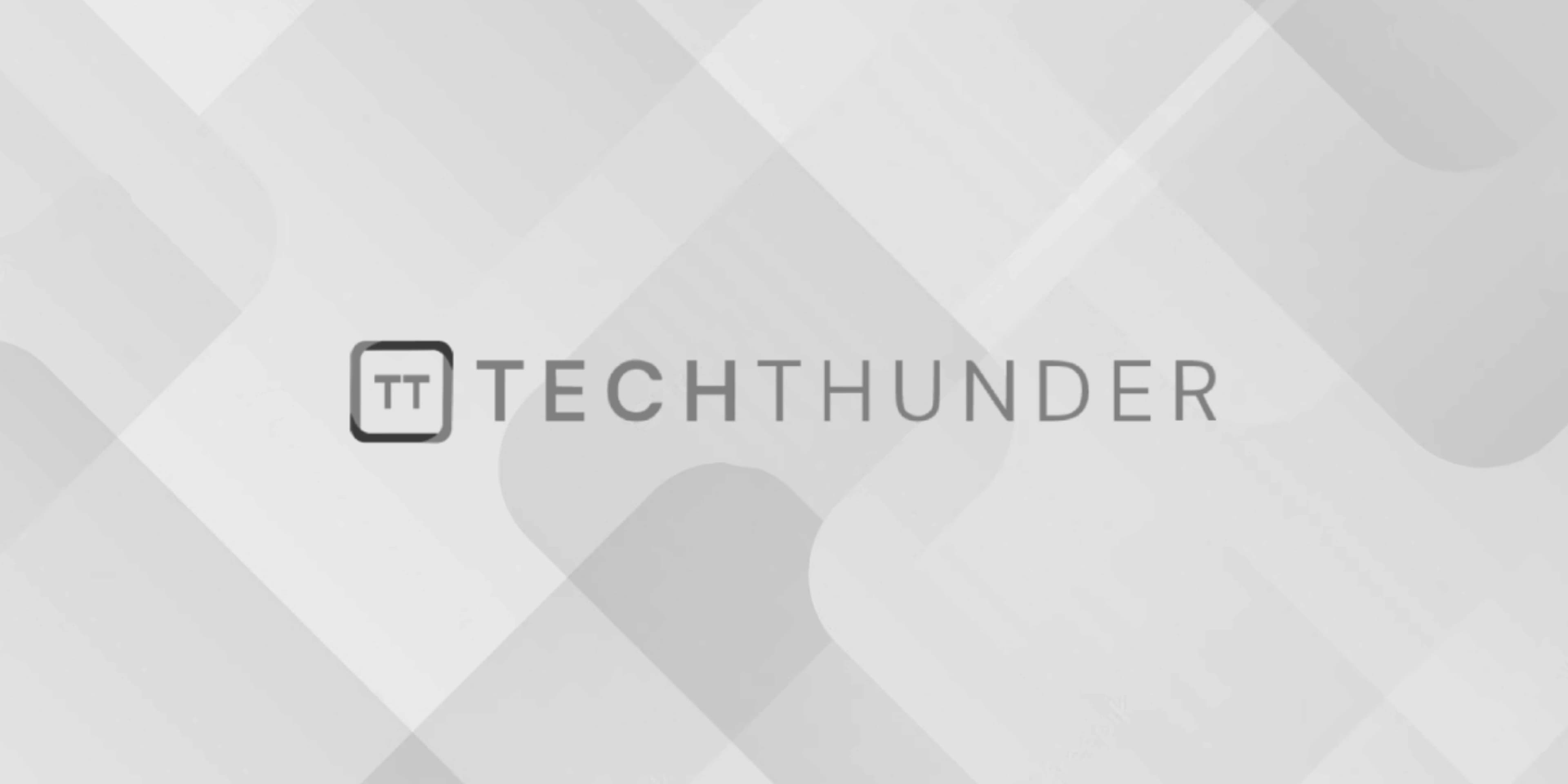
230 views
How to find factorial of a number in JavaScript
To find the factorial of a number in JavaScript, you can use a loop or a recursive function. Here’s an example using both approaches:
- Using a loop (for loop):
JavaScript
function factorialLoop(n) {
if (n === 0 || n === 1) {
return 1;
} else {
let factorial = 1;
for (let i = 2; i <= n; i++) {
factorial *= i;
}
return factorial;
}
}
console.log(factorialLoop(5)); // Output: 120
- Using recursion:
JavaScript
function factorialRecursive(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorialRecursive(n - 1);
}
}
console.log(factorialRecursive(5)); // Output: 120
In both approaches, the factorial of a number n
is calculated by multiplying all the numbers from 1 to n
together. The base case for the recursion is when n
is 0 or 1, where the factorial is always 1. The loop iterates from 2 to n
, accumulating the factorial value in each iteration. The recursive function calls itself with a decreasing value of n
until it reaches the base case.
You can replace the argument (5
in the examples) with any positive integer to calculate the factorial of that number.