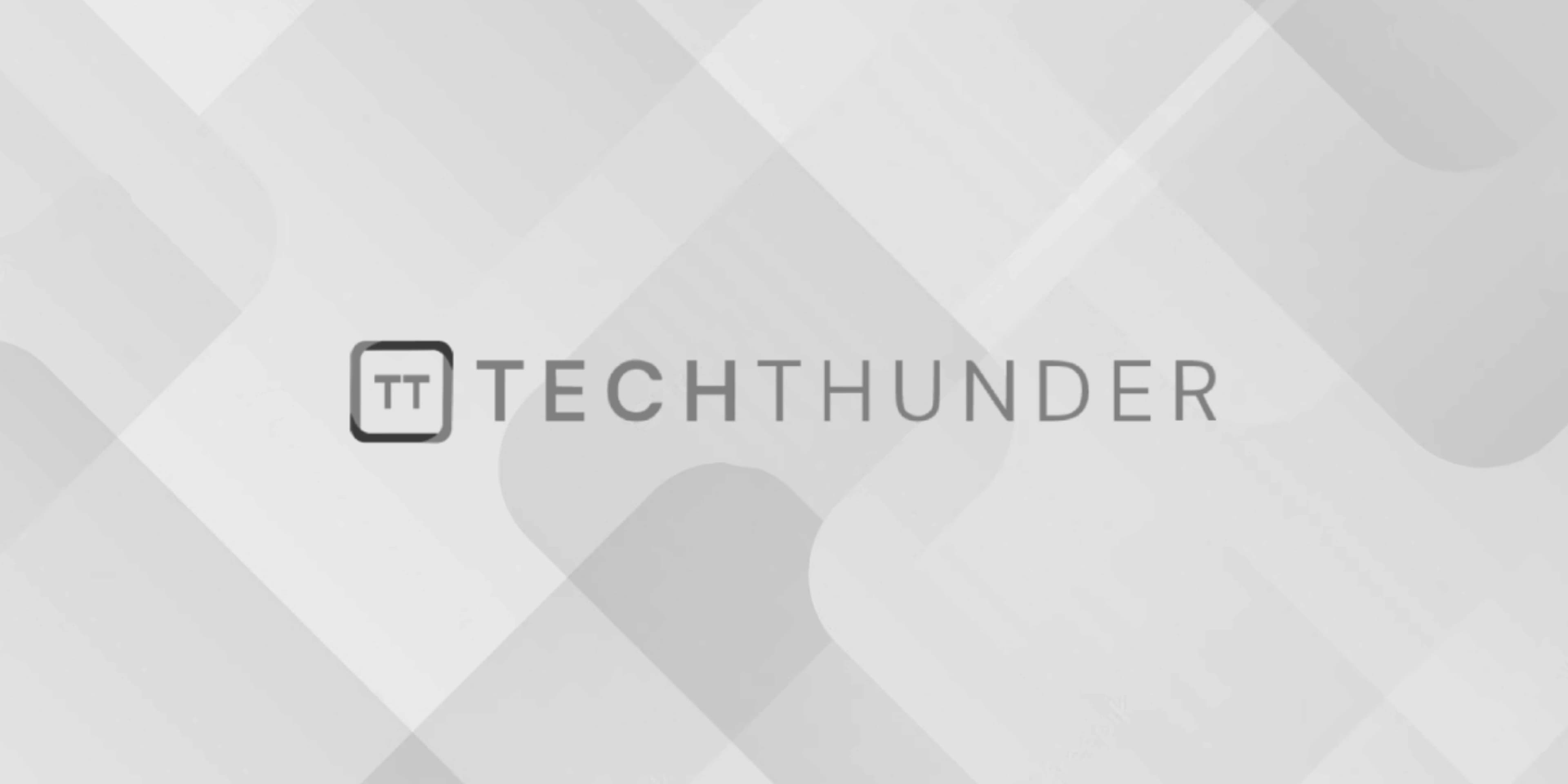
Lodash_.chain() Method
The _.chain()
method in Lodash is used to create a chain sequence. It wraps the given value into a Lodash wrapper object, allowing you to chain multiple Lodash methods together to perform a series of operations on the data. It provides a more concise and fluent way of working with Lodash functions.
Here’s an example to demonstrate the usage of _.chain()
:
var _ = require('lodash');
var numbers = [1, 2, 3, 4, 5];
var result = _.chain(numbers)
.filter(function(num) {
return num % 2 === 0;
})
.map(function(num) {
return num * 2;
})
.value();
console.log(result); // [4, 8]
In this example, we have an array numbers
containing integers. We use _.chain()
to create a chain sequence, and then we chain multiple Lodash methods together: filter()
and map()
. Finally, we call .value()
to retrieve the final result.
The _.chain()
method takes a single argument, which is the initial value to be wrapped in the Lodash wrapper object. In the example, we pass the numbers
array to create the chain.
By chaining multiple Lodash methods, you can perform a series of transformations on the data. In the example, we filter out the even numbers using filter()
, and then we double each number using map()
.
Finally, to retrieve the result, we call .value()
at the end of the chain. This will extract the final value from the chain sequence.
Note that when using _.chain()
, you need to end the chain with .value()
to obtain the final result. Without .value()
, the chain sequence remains unresolved and won’t execute the chained operations.
The _.chain()
method is useful when you want to perform multiple Lodash operations in a concise and chained manner, improving the readability and maintainability of your code.