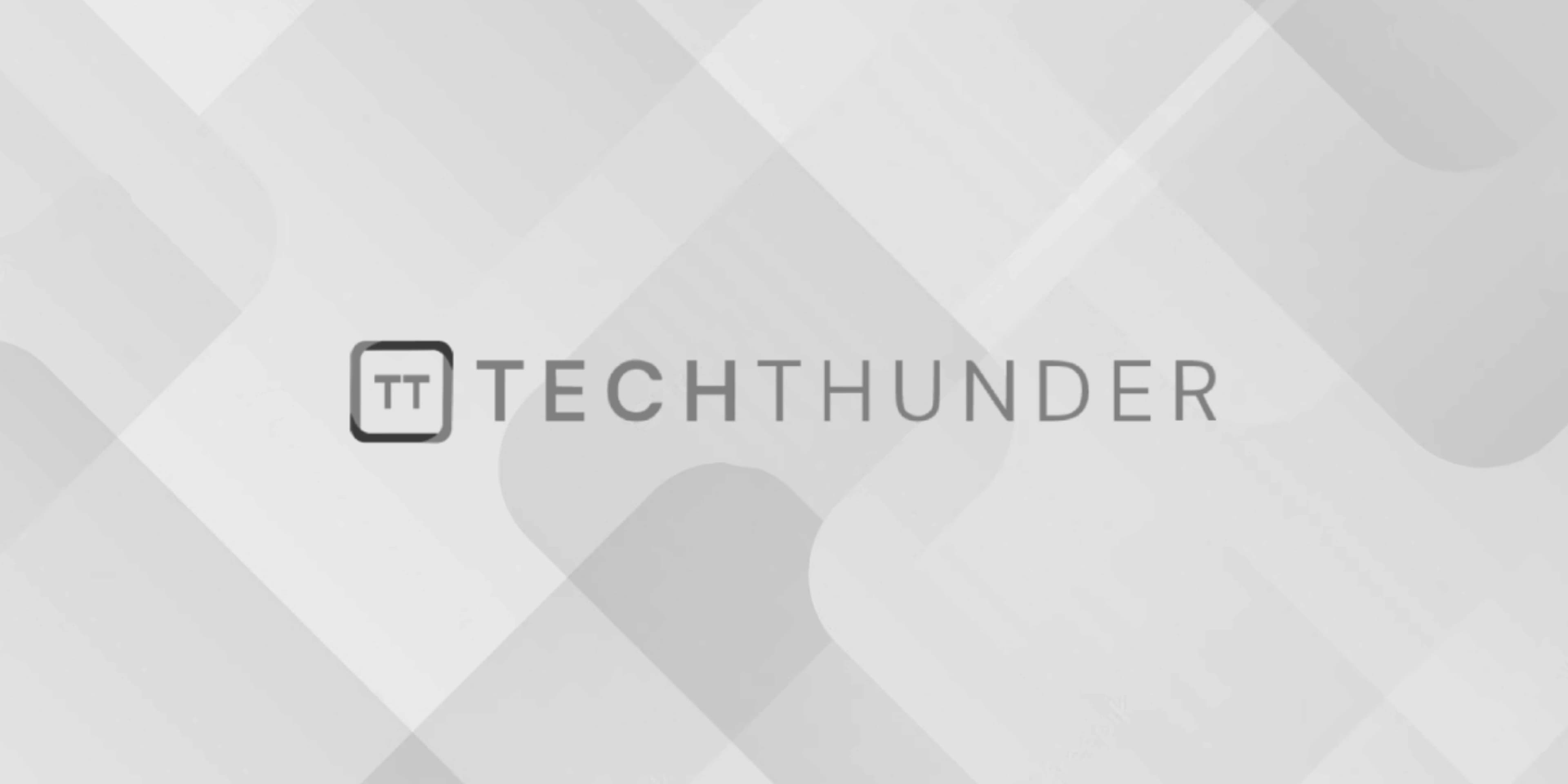
115 views
How to call JavaScript function in html
To call a JavaScript function in HTML, you can use the following methods:
- Inline event handlers: You can directly call a JavaScript function from an HTML element’s event attribute. For example, to call a function named
myFunction()
when a button is clicked, you can use theonclick
attribute:
HTML
<button onclick="myFunction()">Click me</button>
- Event listeners: You can use JavaScript to attach event listeners to HTML elements. This allows you to define the event handler function separately in your JavaScript code. Here’s an example using the
addEventListener
method:
HTML
<button id="myButton">Click me</button>
<script>
// Attach event listener to the button element
document.getElementById("myButton").addEventListener("click", myFunction);
// Event handler function
function myFunction() {
// Function code here
}
</script>
- Script tags: You can place your JavaScript code inside
<script>
tags directly in your HTML file. Declare and define your function within the<script>
tags, and then call it wherever needed in your HTML. Here’s an example:
HTML
<script>
// Function declaration and definition
function myFunction() {
// Function code here
}
</script>
<button onclick="myFunction()">Click me</button>
- External JavaScript file: You can create a separate JavaScript file (e.g.,
script.js
) and include it in your HTML using the<script>
tag with thesrc
attribute. Once the JavaScript file is included, you can call its functions from your HTML. Here’s an example:
HTML file:
HTML
<script src="script.js"></script>
<button onclick="myFunction()">Click me</button>
script.js file:
JavaScript
// Function declaration and definition
function myFunction() {
// Function code here
}
These methods allow you to call JavaScript functions in HTML based on your specific needs and code organization. Choose the method that best suits your requirements and coding style.