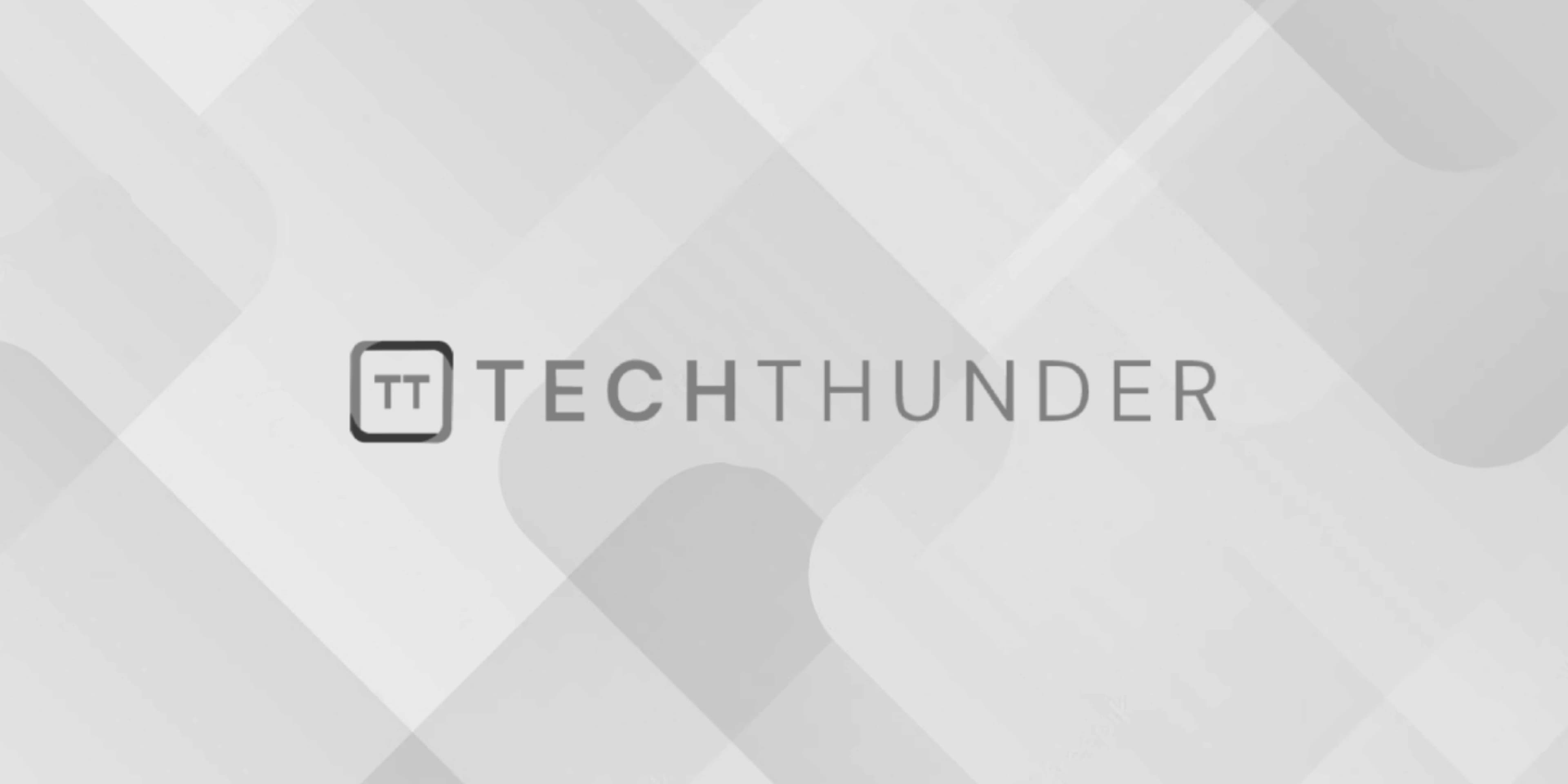
279 views
JavaScript classList
The classList
property in JavaScript is an object that represents the classes of an HTML element. It provides a convenient way to manipulate the class names of an element, allowing you to add, remove, toggle, or check for the presence of classes.
Here are some commonly used methods of the classList
object:
add(className)
: Adds a class to the element’s class list.remove(className)
: Removes a class from the element’s class list.toggle(className)
: Toggles the presence of a class. If the class exists, it is removed; otherwise, it is added.contains(className)
: Checks if the element has a specific class. It returnstrue
if the class is present, orfalse
otherwise.item(index)
: Retrieves the class name at the specified index in the class list.toString()
: Returns a string representation of the class list.
Here’s an example usage of classList
:
JavaScript
// HTML: <div id="myDiv" class="foo bar"></div>
const element = document.getElementById("myDiv");
// Adding a class
element.classList.add("baz");
// Result: <div id="myDiv" class="foo bar baz"></div>
// Removing a class
element.classList.remove("foo");
// Result: <div id="myDiv" class="bar baz"></div>
// Toggling a class
element.classList.toggle("active");
// Result: <div id="myDiv" class="bar baz active"></div>
// Checking if a class exists
const hasClass = element.classList.contains("bar");
console.log(hasClass); // Output: true
// Accessing class names by index
const firstClass = element.classList.item(0);
console.log(firstClass); // Output: "bar"
// Getting a string representation of the class list
const classListString = element.classList.toString();
console.log(classListString); // Output: "bar baz active"
Note that the classList
property is supported in modern browsers, including IE10 and above.