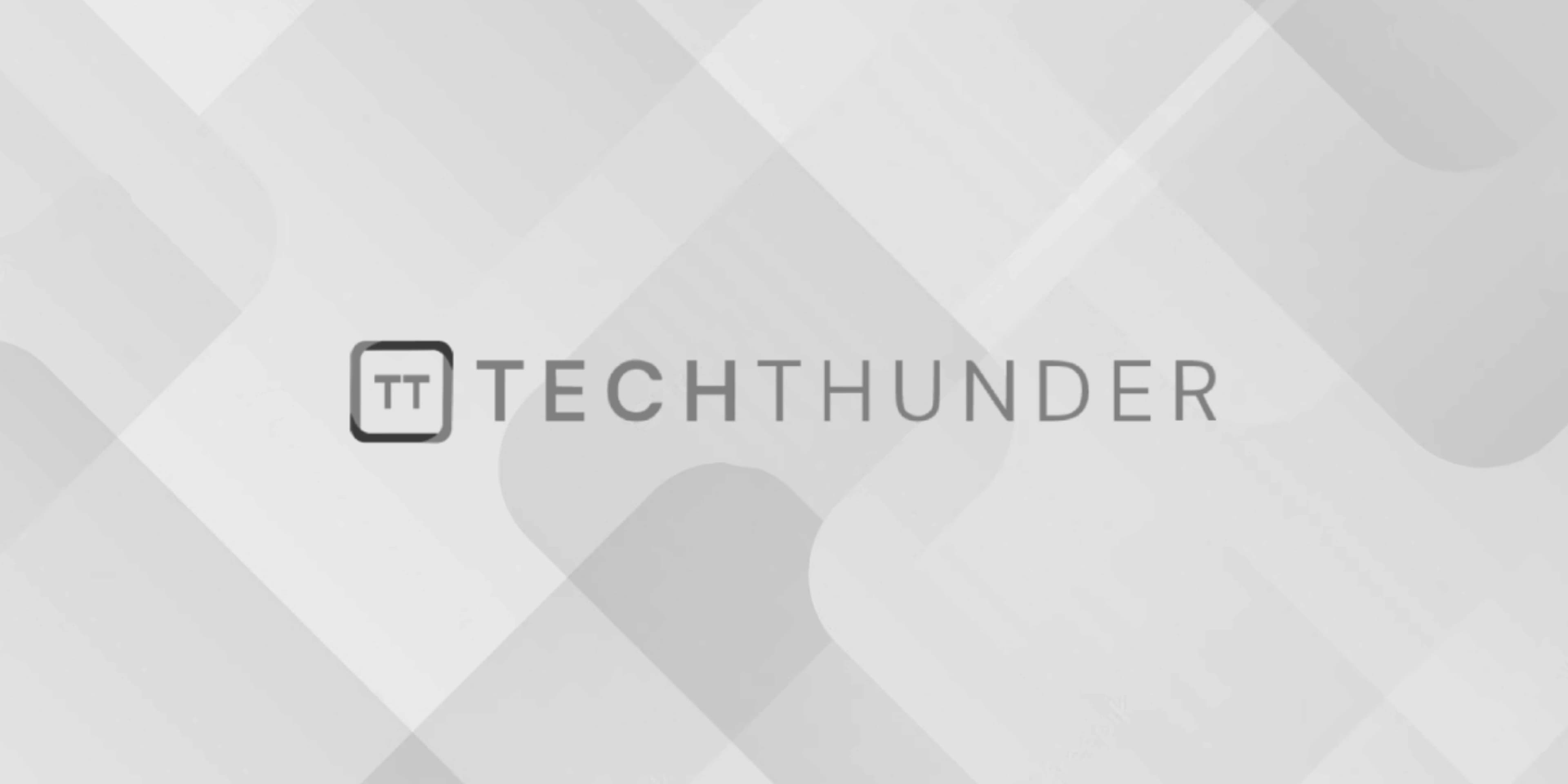
How to Toggle Password Visibility in JavaScript
To toggle password visibility in JavaScript, you need to manipulate the type
attribute of an HTML input element from "password"
to "text"
and vice versa. This can be achieved by handling an event, such as clicking on an eye icon, and toggling the type
attribute accordingly. Here’s an example:
HTML:
<input type="password" id="passwordInput">
<button id="toggleButton" onclick="togglePasswordVisibility()">Toggle</button>
JavaScript:
function togglePasswordVisibility() {
var passwordInput = document.getElementById("passwordInput");
if (passwordInput.type === "password") {
passwordInput.type = "text";
} else {
passwordInput.type = "password";
}
}
In this example, the HTML code consists of an <input>
element with type="password"
to hide the password characters by default. Additionally, there is a <button>
element with an onclick
attribute calling the togglePasswordVisibility()
function when clicked.
The JavaScript code defines the togglePasswordVisibility()
function. Inside the function, we retrieve the password input element using document.getElementById("passwordInput")
. Replace "passwordInput"
with the actual ID of your input element.
The function checks the current type
attribute of the input element. If it is "password"
, indicating that the password is currently hidden, the function sets the type
attribute to "text"
, making the password visible. If the type
attribute is already "text"
, indicating that the password is currently visible, the function sets the type
attribute back to "password"
to hide the password characters.
By executing this code, clicking the “Toggle” button will alternate between showing and hiding the password characters in the input field, providing a password visibility toggle functionality.