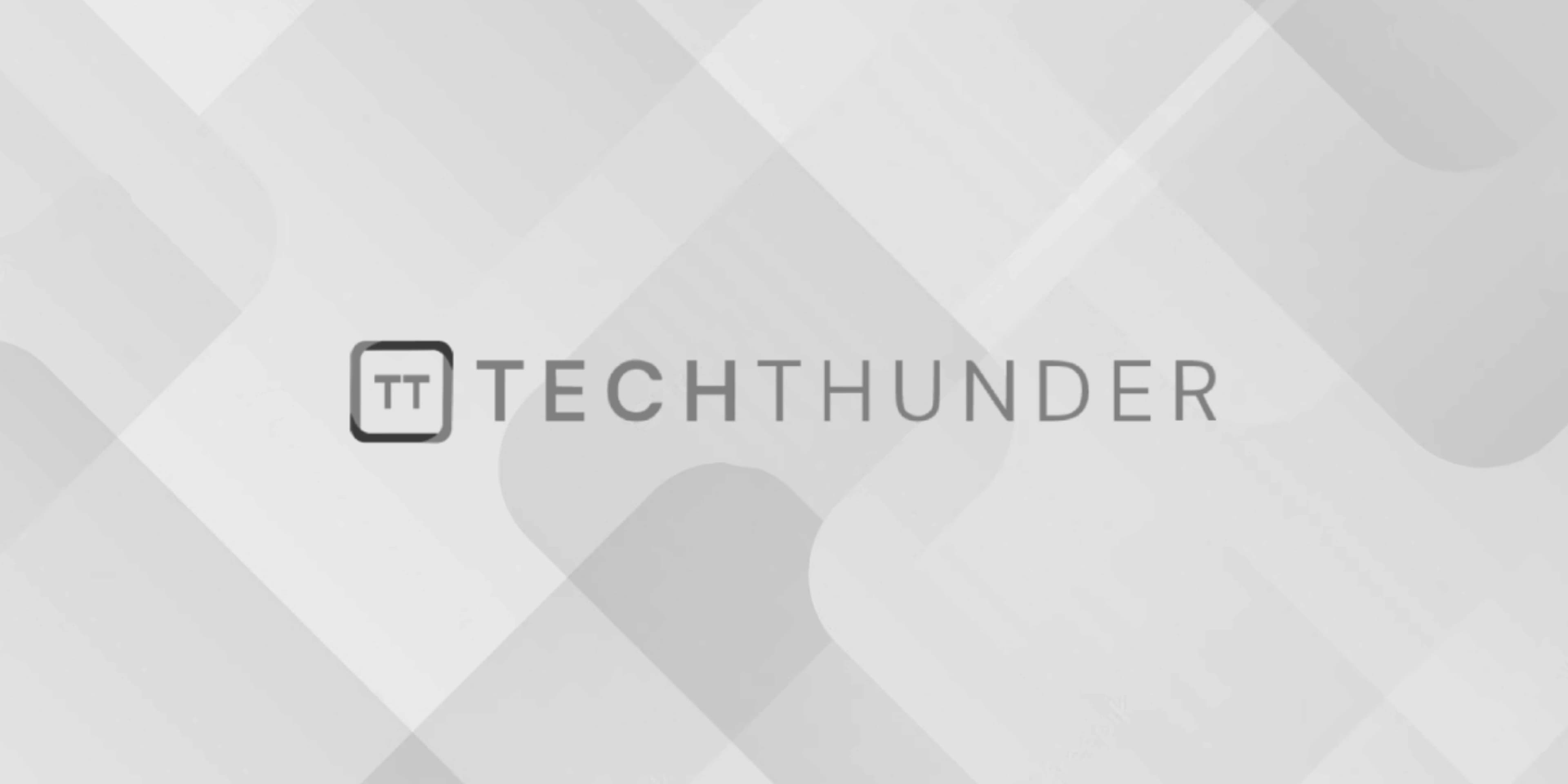
JavaScript form validation
JavaScript form validation is the process of validating user input in HTML forms using JavaScript code. It allows you to ensure that the data entered by the user meets certain criteria or constraints before it is submitted to the server.
There are different approaches to implementing form validation in JavaScript, but here’s a basic example using the onsubmit
event handler and the required
attribute:
HTML form:
<form onsubmit="return validateForm()">
<input type="text" id="name" required>
<input type="email" id="email" required>
<input type="submit" value="Submit">
</form>
JavaScript validation function:
function validateForm() {
const nameInput = document.getElementById("name");
const emailInput = document.getElementById("email");
if (nameInput.value.trim() === "") {
alert("Please enter your name.");
return false; // prevent form submission
}
if (emailInput.value.trim() === "") {
alert("Please enter your email.");
return false; // prevent form submission
}
// Additional validation logic...
return true; // allow form submission
}
In this example, the validateForm()
function is called when the form is submitted (onsubmit
). It retrieves the input values from the name and email fields, trims any leading/trailing spaces, and checks if they are empty. If any field is empty, an alert is shown and the function returns false
to prevent form submission. If all fields pass validation, the function returns true
to allow form submission.
You can add additional validation logic as needed, such as validating email format, password strength, or enforcing specific constraints on input values.